-
Notifications
You must be signed in to change notification settings - Fork 3
09 Fetch Callback
JP Barbosa edited this page Apr 28, 2021
·
5 revisions
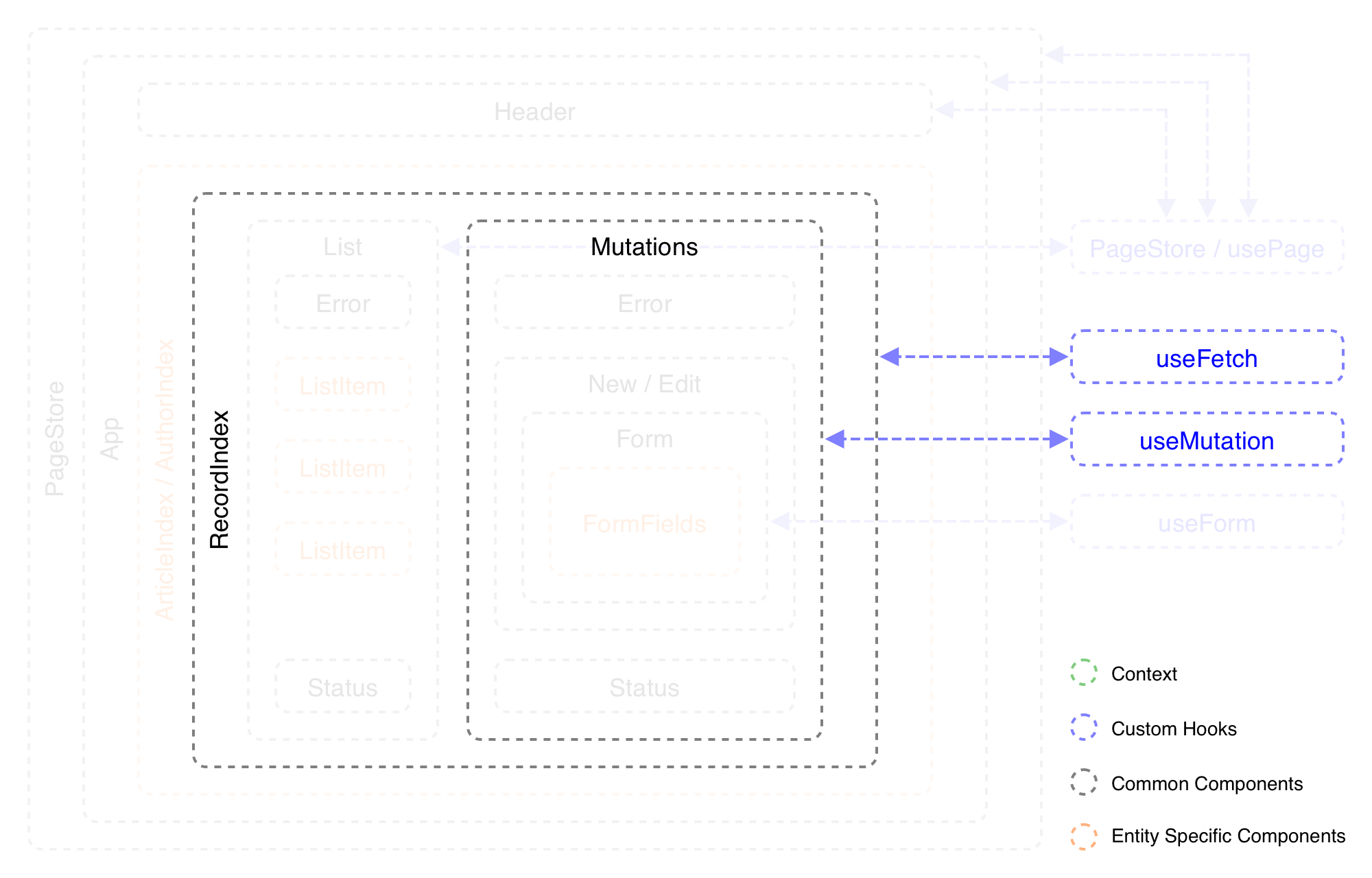
code ./src/hooks/useFetch.ts
...
export const useFetch = <...>(...) => {
...
const [version, setVersion] = useState(+new Date());
...
useEffect(() => {
...
}, [..., version]);
return { ..., setVersion };
};
code ./src/hooks/useMutation.ts
...
export const useMutation = <T extends Record>(
...,
callback?: Function
) => {
...
const wrap = (fn: Action<T>) => {
return async (record: T) => {
fn(record).then(() => {
if (callback) {
callback();
}
});
};
};
const create: Action<T> = wrap(async (record: T) => {
await axios.post(url, record);
});
const update: Action<T> = wrap(async (record: T) => {
await axios.put(`${url}/${record.id}`, record);
});
const remove: Action<T> = wrap(async (record: T) => {
await axios.delete(`${url}/${record.id}`);
});
return {
...
};
};
code ./src/pages/Record/index.tsx
...
export const RecordIndex = <...>(...) => {
...
const { ..., setVersion } = useFetch<T>(...);
const callback = () => {
setVersion(+new Date());
setActiveRecord(emptyRecord);
};
return (
<div className="page">
<div className="content">
...
<RecordMutations<T>
...
callback={callback}
/>
</div>
</div>
);
};
code ./src/interfaces/PagesProps.ts
export interface RecordMutationsProps<T> {
...
callback: Function;
}
code ./src/pages/Record/Mutations.tsx
...
export const RecordMutations = <T extends Record>({
...
callback,
}: RecordMutationsProps<T>) => {
const { ... } = useMutation<T>(..., callback);
return (
...
);
};
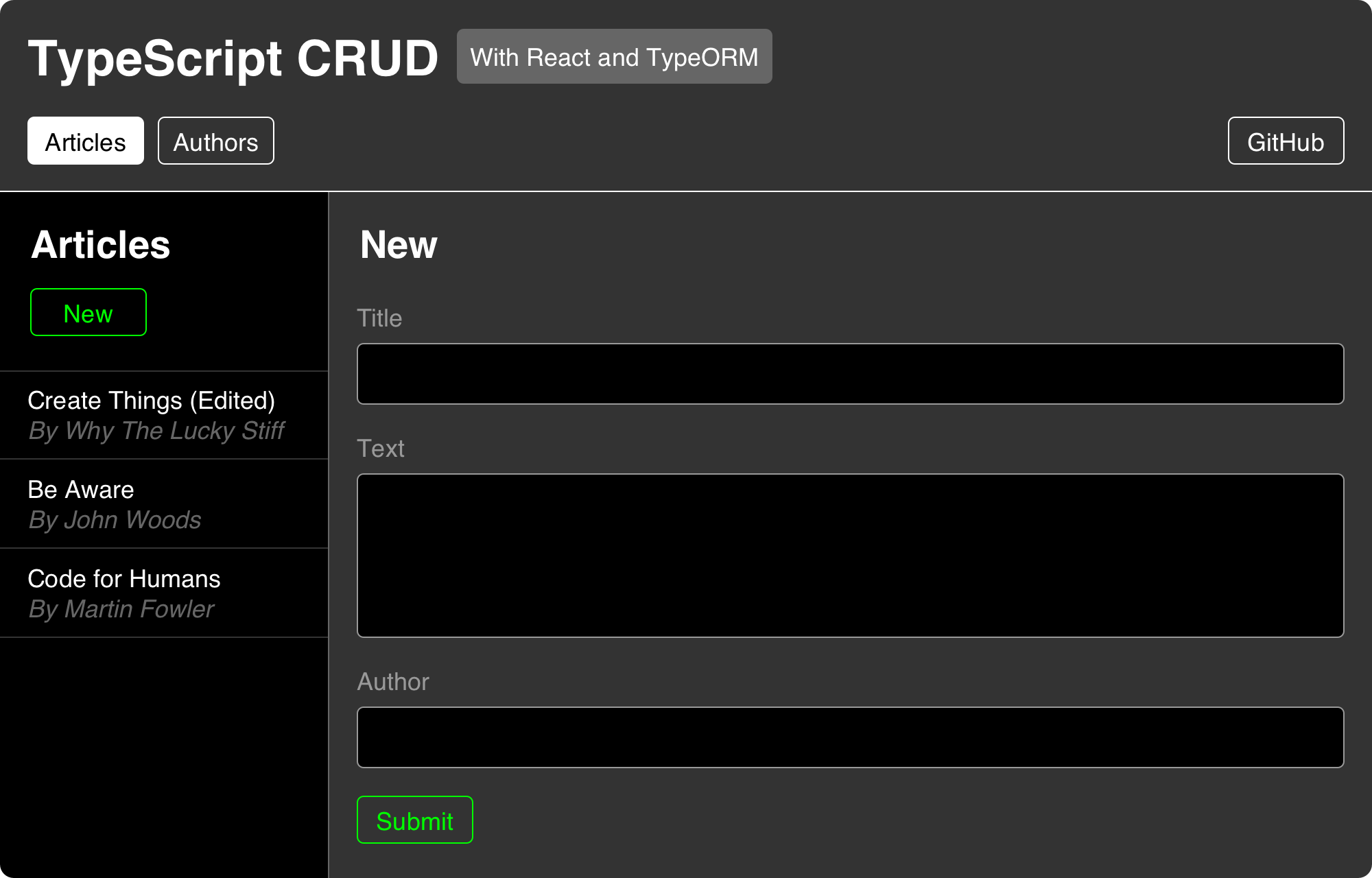
git add .
git commit -m "Fetch Callback"