-
Notifications
You must be signed in to change notification settings - Fork 3
10 Fetch Status
JP Barbosa edited this page Oct 15, 2022
·
5 revisions
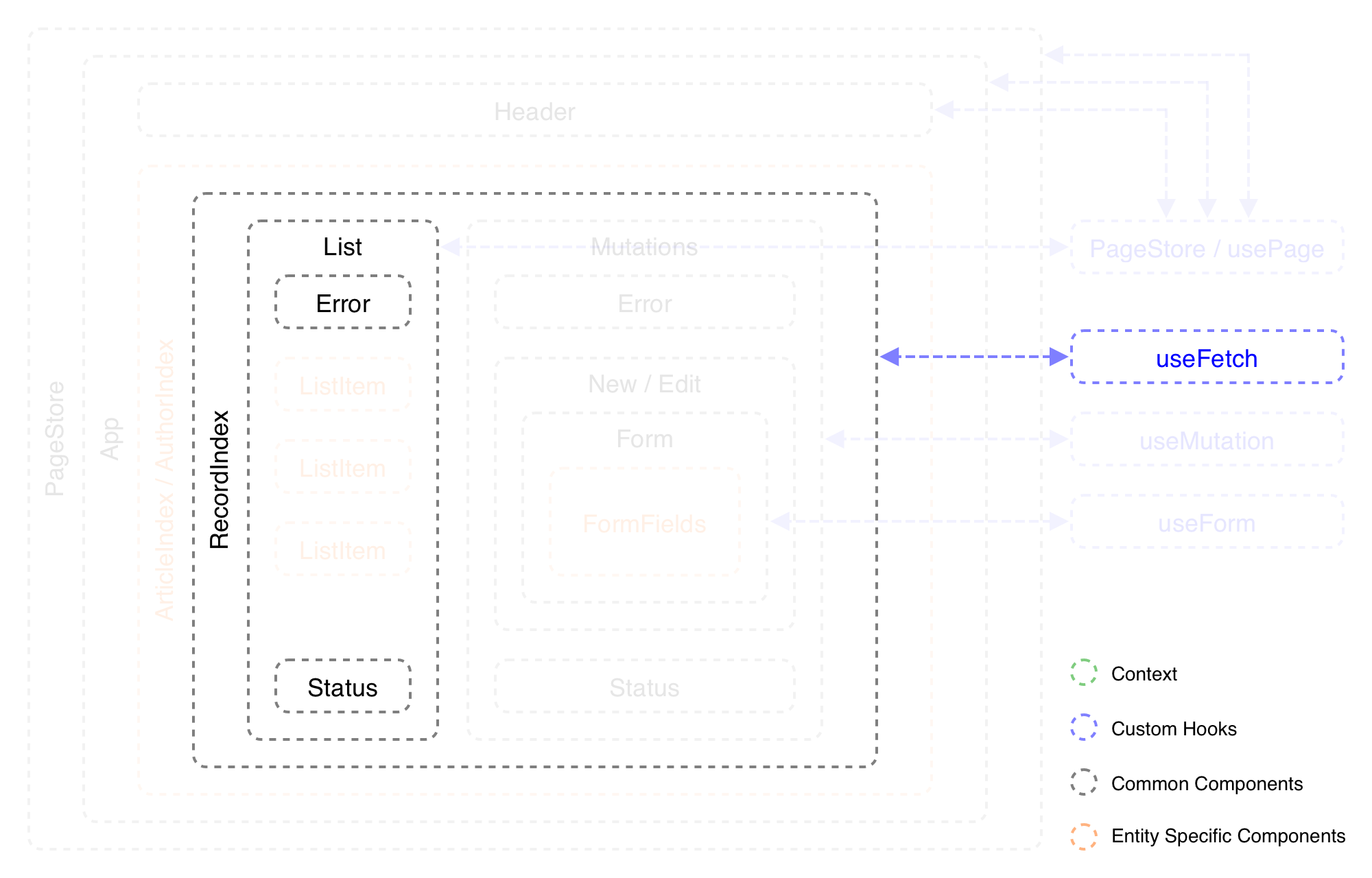
code ./src/hooks/useFetch.ts
import ..., { AxiosError } from 'axios';
...
export const useFetch = <...>(...) => {
...
const [loading, setLoading] = useState<boolean>(false);
const [error, setError] = useState<AxiosError>();
...
useEffect(() => {
const callFetchFunction = async () => {
setLoading(true);
try {
const res = await axios.get<T[]>(url, { params: options });
setRecords(res.data);
} catch (e) {
setError(e as AxiosError);
}
setLoading(false);
};
callFetchFunction();
}, [url, options, version]);
return { ..., loading, error };
};
code ./src/pages/Record/index.tsx
...
export const RecordIndex = <...>(...) => {
...
const { ..., loading, error } = useFetch<T>(...);
...
return (
<div className="page">
<div className="content">
<RecordList<T>
...
loading={loading}
error={error}
/>
...
</div>
</div>
);
};
code ./src/interfaces/PagesProps.ts
import { AxiosError } from 'axios';
...
export interface RecordListProps<T> {
...
loading: boolean;
error?: AxiosError;
}
code ./src/pages/Record/List.tsx
...
import { Status } from '../../components/Status';
import { RecordError } from '../../components/RecordError';
export const RecordList = <...>({
...
loading,
error,
}: RecordListProps<T>) => {
return (
<div className="list">
{error && <RecordError error={error} />}
<div>
...
</div>
{loading && <Status text="Loading..." />}
</div>
);
};
code ./src/components/Status.tsx
interface IProps {
text: string;
}
export const Status: React.FC<IProps> = ({ text }) => {
return (
<div className="status">
<span className="status-text">{text}</span>
</div>
);
};
code ./src/components/RecordError.tsx
import { AxiosError } from 'axios';
interface IProps {
error: AxiosError<any>;
}
export const RecordError: React.FC<IProps> = ({ error }) => {
return (
<div className="error">
<div>
<b>Message:</b> {error.message}
</div>
<div>
<b>URL:</b> {error.config?.url}
</div>
{error.response?.data.message && (
<div>
<b>Details:</b> {error.response?.data.message}
</div>
)}
</div>
);
};
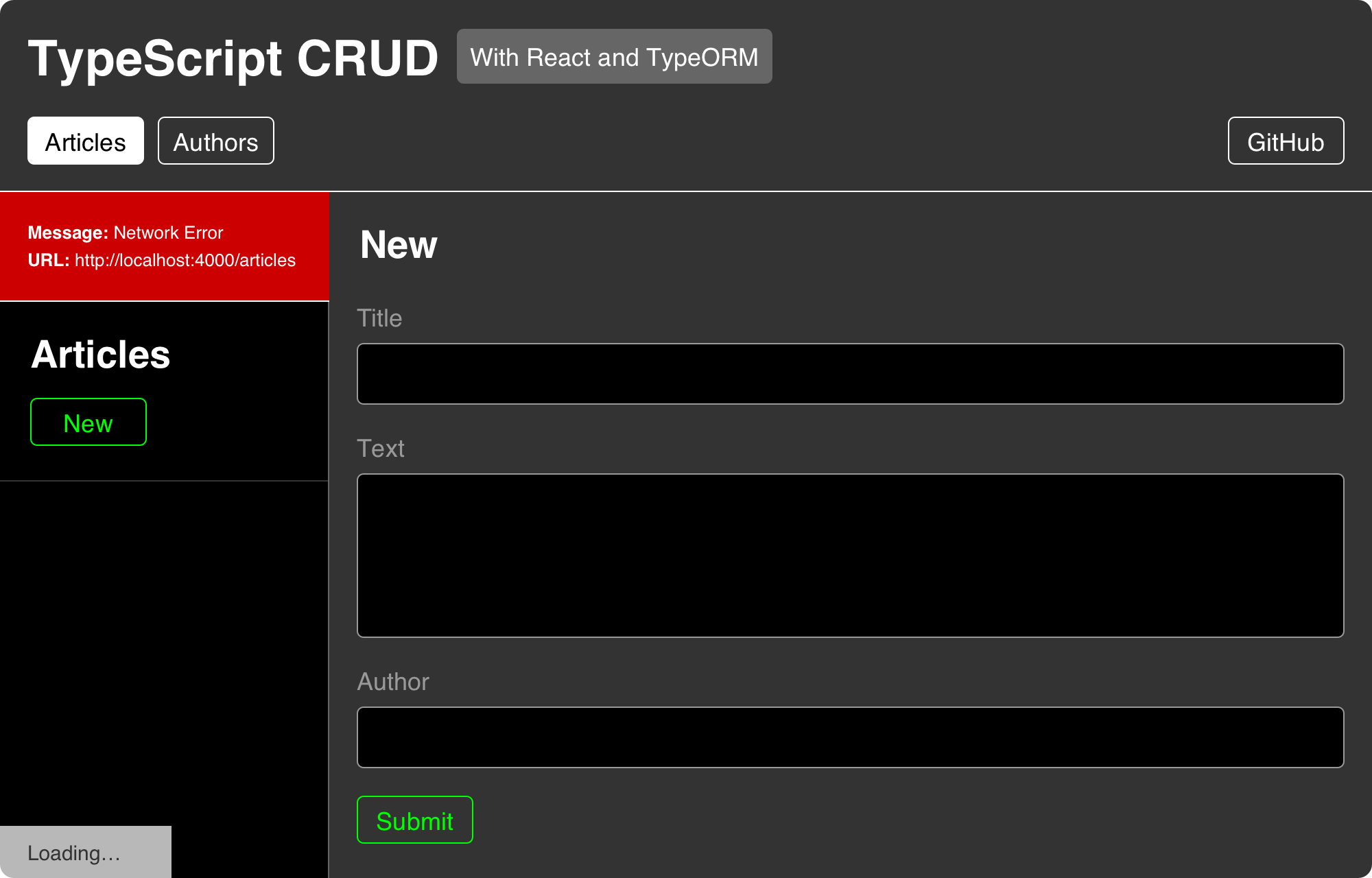
git add .
git commit -m "Fetch Status"