-
Notifications
You must be signed in to change notification settings - Fork 293
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Article suggestion: demonstrate multi-step geometry manipulation #578
Comments
That's a nice article, and IMO a candidate to be wrapped into something simpler: a new function or an option under an existing one. Does this activity have a name in GIS or spatial database world? I wrote |
I revised the example to show how this might work as a function instead of a series of I'm not sure if this activity has a formal name in GIS. I've been calling it an "area-weighted spatial join" but others have different names for it: |
Sometimes called majority fill or maximum area overlay -- but I believe a standard (faster?) approach is to rasterize the base layer to an appropriate resolution and then use |
@tiernanmartin something went wrong, it seems, e.g. when assigning |
@mbacou "maximum area overlay" sounds good to me and thanks for the the tip about using @edzer You're right - the function version above contains a mistake. I'll see if I can sleuth it out... I played around with I haven't tested it with large datasets yet, but I'm wondering if this may be another instance where |
"largest" parameter as is now in st_join would also add a lot to raster::extract(), as an additional option for "fun" https://www.rdocumentation.org/packages/raster/versions/3.1-5/topics/extract |
But that is an issue for raster, right? |
I recently found myself scratching my head over how to do a pretty common GIS procedure using the
sf
package: join one set of multipolygon data to another based on the highest amount of spatial intersection.A quick Stackoverflow search turned up a postgis solution, but reverse engineering it with
sf
took me quite a bit of time.That made me wish that the
sf
documentation had an example showing how to apply a sequence of steps that combine verbs fromsf
and othertidyverse
packages likedplyr
,tidyr
, andpurrr
.After my project I threw together a reprex to illustrate the approach that I settled on - my hope is that it sparks a discussion that then informs a new section of the geometry manipulation article (or perhaps a separate article entirely).
Here's an image (reprex below) that uses the
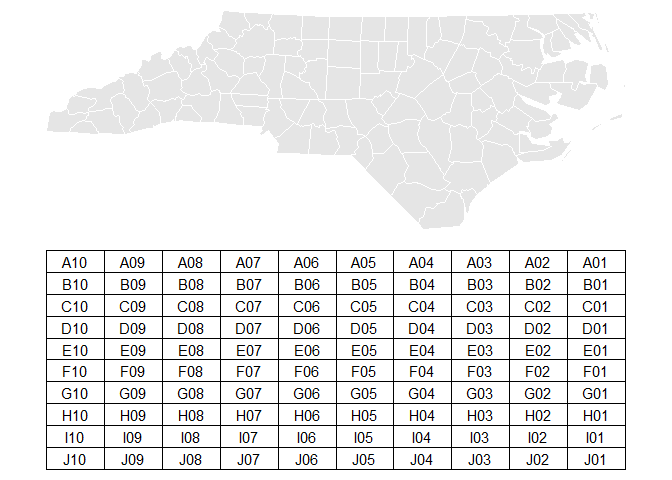
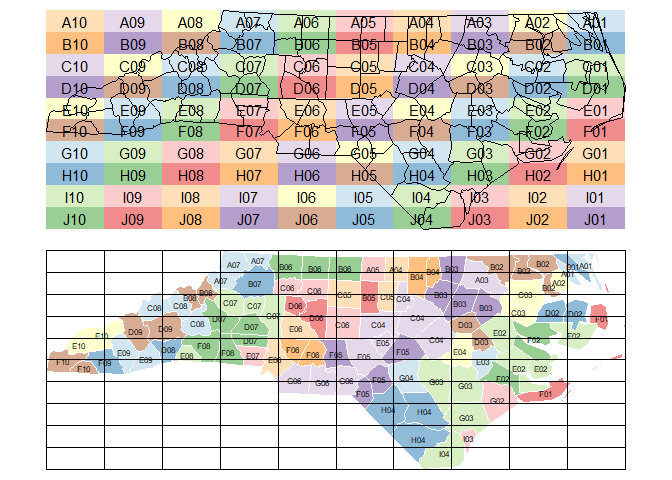
nc
dataset to illustrate the result I was looking for:Update:
Here's my approach wrapped up in a function:
It requires the user to follow up with
group_by %>% top_n(1, INTERSECT_AREA) %>% ungroup
- that could probably be improved.note: the reprex below has been updated to show the above function in action
Reprex + Session Info
The text was updated successfully, but these errors were encountered: