Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Support customization of fields on derive #129
Support customization of fields on derive #129
Changes from 1 commit
193341a
f73d5f2
8913ec0
66b12e1
9dd430d
727ab88
f3225dd
fb58d7a
8cbad14
479da32
2701ed8
4fdf16f
755b8e0
File filter
Filter by extension
Conversations
Jump to
There are no files selected for viewing
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Can we also add some doc tests that use
compile_fail
to check that we return compiler errors when#[arbitrary]
is used multiple times on a single field#[arbitrary(unknown_attr)]
and#[arbitrary(unknown_attr = unknown_val)]
are used#[arbitrary(value)]
and#[arbitrary(with)]
are used without RHS valuesShould be able to just do something like the following in this file:
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Addressed in 8913ec0
I was puzzled where to put such doc tests (I don't think users really want to see them in the docs).
Eventually I look into
serde
again, they're using trybuild to test compile failures.So I've decided to introduce
trybuild
to this project too, I think it's a nice tool for this purpose.There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Was doing doc tests on a
pub struct CompileFailTests
intests/derive.rs
not getting picked up by rustdoc?If not, then we could maybe avoid a new dependency by doing
So that it never shows up in the docs and only is built when we are running tests (with the derive enabled).
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@fitzgen I just tried doc tests within
derive/src/lib.rs
, they are not picked up.It's
dev-dependency
, shouldn't it be fine? I definitely liketrybuild
, it generates really helpful error messages and allows to structure tests in more easy to understand / maintainable way.For example, this is what a reported failure looks like:
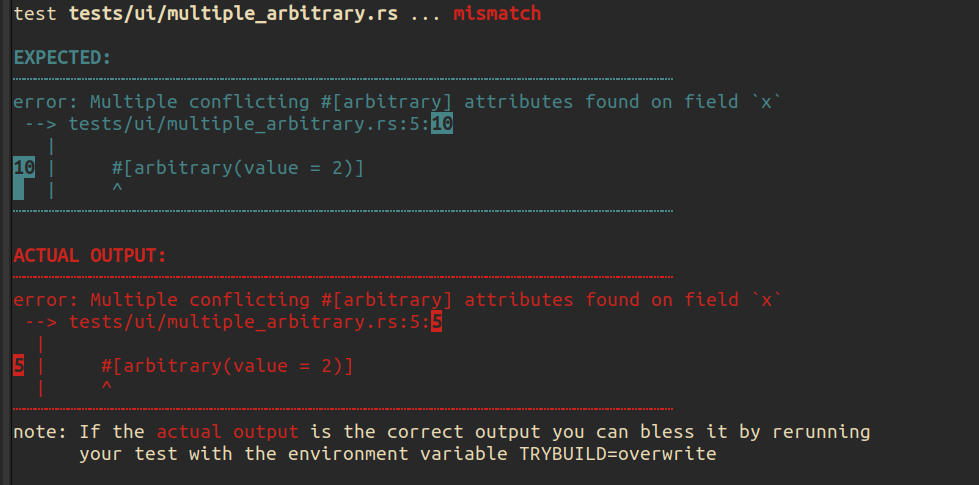
This way we actually test, the compiler fails in the way we expect it to fail. Doc tests may give false positives if something in the code gets mistyped.
I would see the cost of using
trybuild
is rather potential failures ifrustc
changes the way it prints errors.But still I think pros of
trybuild
outweights its cons.Please let me know, if you still insist on using doc tests.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I think if the test is in
src/lib.rs
rather thanderive/src/lib.rs
it should work? I'd really want to avoid failing tests on nightly but not stable unless as an absolute last resort.