forked from rust-lang/rust
-
Notifications
You must be signed in to change notification settings - Fork 7
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Rollup merge of rust-lang#123797 - amandasystems:better-graphviz, r=oli-obk Better graphviz output for SCCs and NLL constraints This PR modifies the output for `-Z dump-mir-graphviz=yes`. Specifically, it changes the output of the files `.-------.nll.0.regioncx.all.dot` and `nll.0.regioncx.scc.dot` to be easier to read and contain some information that helped me during debugging. In particular: - SCC indices are contracted to `SCC(n)` instead of `ConstraintSccIndex(n)` to compress the nodes - SCC regions are in `{}` rather than `[]` (controversial since they are technically ordered by index, but I figured they're more sets than arrays conceptually since they're equivalence classes). - For regions in other universes than the root, also show the region universe (as ?8/U1) - For regions with external names, show the external name in parenthesis - For the region graph where edges are locations, render the All variant of the enum without the file since it's extremely long and often destroys the rendering - For region graph edge annotations for single locations, remove the wrapping around the Location variant and just add its contents since this can be unambiguously done Example output (from the function `foo()` of `tests/ui/error-codes/E0582.rs`) for an SCC graph:  ...and for the constraints: 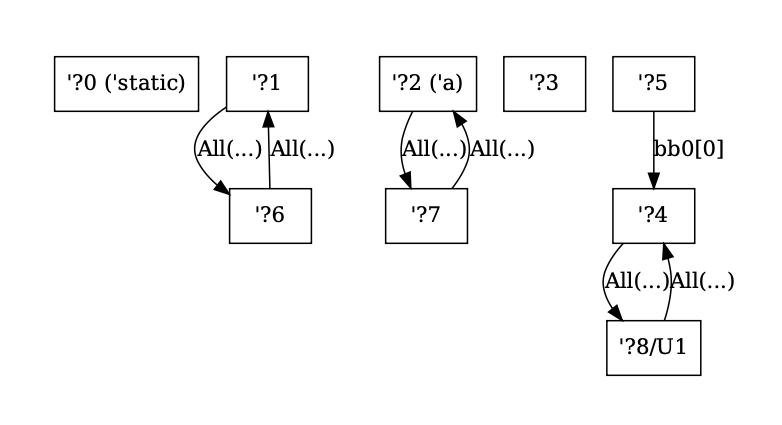 This PR also gives `UniverseIndex`es the `is_root()` method since this is now an operation that happens three times in the borrowck crate.
- Loading branch information
Showing
4 changed files
with
43 additions
and
6 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters