-
Notifications
You must be signed in to change notification settings - Fork 12.4k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Can't implement a const Option::unwrap
because of "destructors cannot be evaluated at compile-time" error.
#66753
Comments
This was discussed here in the initial PR for cc @rust-lang/wg-const-eval |
This might need to consider |
So... we have three situations afaict:
So... if even drop elaboration can't figure this out, I'm not sure const qualif should be finding an independent solution. Several solutions come to mind:
cc @eddyb |
I think there's something missing from drop elaboration's (and other analyses'?) dataflow usage: So the The annoying thing is that we also have to be able to check this in const-checking. Hang on... promotion has to run before borrow-checking because it modifies the MIR. |
I'm planning to investigate eddyb's idea early next week (they gave me some instructions to follow over Zulip). This will require a small change to the dataflow analysis API which I'll probably do on top of #65672 just because I'm more familiar with it. Hopefully I can get a proof-of-concept up and running. |
Mark other variants as uninitialized after switch on discriminant During drop elaboration, which builds the drop ladder that handles destruction during stack unwinding, we attempt to remove MIR `Drop` terminators that will never be reached in practice. This reduces the number of basic blocks that are passed to LLVM, which should improve performance. In #66753, a user pointed out that unreachable `Drop` terminators are common in functions like `Option::unwrap`, which move out of an `enum`. While discussing possible remedies for that issue, @eddyb suggested moving const-checking after drop elaboration. This would allow the former, which looks for `Drop` terminators and replicates a small amount of drop elaboration to determine whether a dropped local has been moved out, leverage the work done by the latter. However, it turns out that drop elaboration is not as precise as it could be when it comes to eliminating useless drop terminators. For example, let's look at the code for `unwrap_or`. ```rust fn unwrap_or<T>(opt: Option<T>, default: T) -> T { match opt { Some(inner) => inner, None => default, } } ``` `opt` never needs to be dropped, since it is either moved out of (if it is `Some`) or has no drop glue (if it is `None`), and `default` only needs to be dropped if `opt` is `Some`. This is not reflected in the MIR we currently pass to codegen. 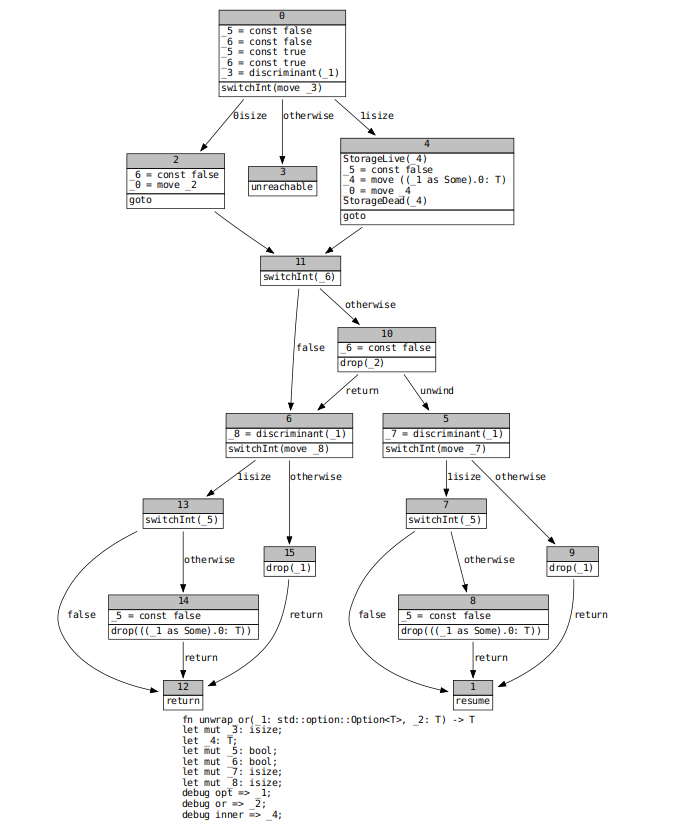 @eddyb also suggested the solution to this problem. When we switch on an enum discriminant, we should be marking all fields in other variants as definitely uninitialized. I implemented this on top of alongside a small optimization (split out into #68943) that suppresses drop terminators for enum variants with no fields (e.g. `Option::None`). This is the resulting MIR for `unwrap_or`. 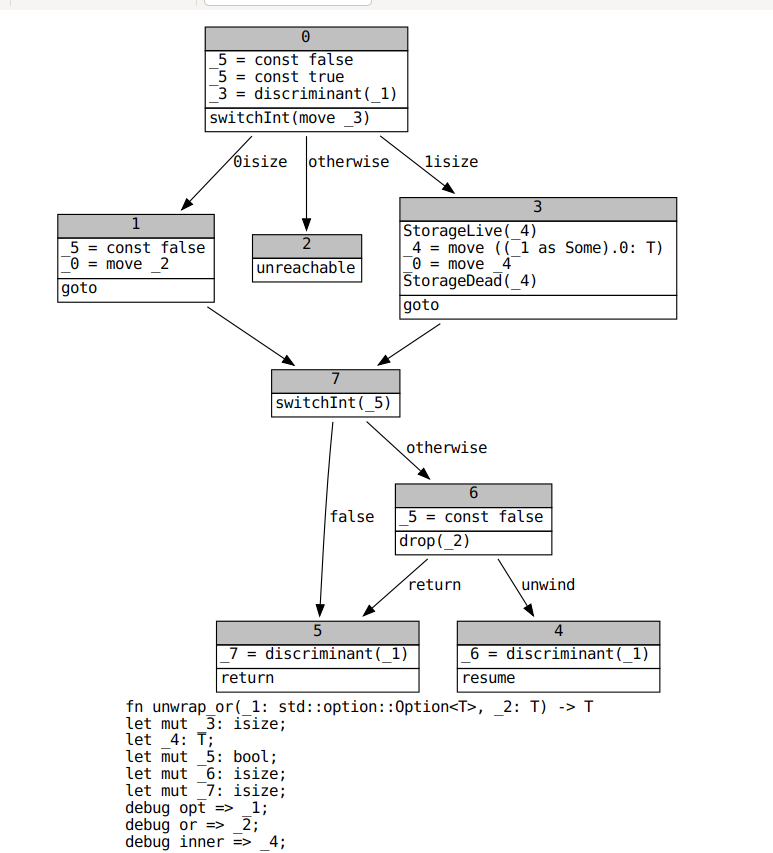 In concert with #68943, this change speeds up many [optimized and debug builds](https://perf.rust-lang.org/compare.html?start=d55f3e9f1da631c636b54a7c22c1caccbe4bf0db&end=0077a7aa11ebc2462851676f9f464d5221b17d6a). We need to carefully investigate whether I have introduced any miscompilations before merging this. Code that never drops anything would be very fast indeed until memory is exhausted.
After #68528 and #68943, the optimized MIR for |
Silly question but - why does this need compiler support? Can't you |
If you mean this: #![feature(const_forget)]
#![feature(const_fn)]
#![feature(const_if_match)]
#![feature(const_panic)]
const fn unwrap<T>(opt:Option<T>)->T{
match opt {
Some(x)=>x,
None=>{
std::mem::forget(opt);
panic!("Trying to unwrap a None")
},
}
} It has no effect on the error message:
Neither does using |
…p-elab, r=oli-obk Check for live drops in constants after drop elaboration Resolves rust-lang#66753. This PR splits the MIR "optimization" pass series in two and introduces a query–`mir_drops_elaborated_and_const_checked`–that holds the result of the `post_borrowck_cleanup` analyses and checks for live drops. This query is invoked in `rustc_interface` for all items requiring const-checking, which means we now do `post_borrowck_cleanup` for items even if they are unused in the crate. As a result, we are now more precise about when drops are live. This is because drop elaboration can e.g. eliminate drops of a local when all its fields are moved from. This does not mean we are doing value-based analysis on move paths, however; Storing a `Some(CustomDropImpl)` into a field of a local will still set the qualifs for that entire local. r? @oli-obk
…p-elab, r=oli-obk Check for live drops in constants after drop elaboration Resolves rust-lang#66753. This PR splits the MIR "optimization" pass series in two and introduces a query–`mir_drops_elaborated_and_const_checked`–that holds the result of the `post_borrowck_cleanup` analyses and checks for live drops. This query is invoked in `rustc_interface` for all items requiring const-checking, which means we now do `post_borrowck_cleanup` for items even if they are unused in the crate. As a result, we are now more precise about when drops are live. This is because drop elaboration can e.g. eliminate drops of a local when all its fields are moved from. This does not mean we are doing value-based analysis on move paths, however; Storing a `Some(CustomDropImpl)` into a field of a local will still set the qualifs for that entire local. r? @oli-obk
…p-elab, r=oli-obk Check for live drops in constants after drop elaboration Resolves rust-lang#66753. This PR splits the MIR "optimization" pass series in two and introduces a query–`mir_drops_elaborated_and_const_checked`–that holds the result of the `post_borrowck_cleanup` analyses and checks for live drops. This query is invoked in `rustc_interface` for all items requiring const-checking, which means we now do `post_borrowck_cleanup` for items even if they are unused in the crate. As a result, we are now more precise about when drops are live. This is because drop elaboration can e.g. eliminate drops of a local when all its fields are moved from. This does not mean we are doing value-based analysis on move paths, however; Storing a `Some(CustomDropImpl)` into a field of a local will still set the qualifs for that entire local. r? @oli-obk
More precise drop elaboration is now behind |
…atch, r=oli-obk Stabilize `#![feature(const_if_match)]` Quoting from the [stabilization report](rust-lang#49146 (comment)): > `if` and `match` expressions as well as the short-circuiting logic operators `&&` and `||` will become legal in all [const contexts](https://doc.rust-lang.org/reference/const_eval.html#const-context). A const context is any of the following: > > - The initializer of a `const`, `static`, `static mut` or enum discriminant. > - The body of a `const fn`. > - The value of a const generic (nightly only). > - The length of an array type (`[u8; 3]`) or an array repeat expression (`[0u8; 3]`). > > Furthermore, the short-circuiting logic operators will no longer be lowered to their bitwise equivalents (`&` and `|` respectively) in `const` and `static` initializers (see rust-lang#57175). As a result, `let` bindings can be used alongside short-circuiting logic in those initializers. Resolves rust-lang#49146. Ideally, we would resolve 🐳 rust-lang#66753 before this lands on stable, so it might be worth pushing this back a release. Also, this means we should get the process started for rust-lang#52000, otherwise people will have no recourse except recursion for iterative `const fn`. r? @oli-obk
…atch, r=oli-obk Stabilize `#![feature(const_if_match)]` Quoting from the [stabilization report](rust-lang#49146 (comment)): > `if` and `match` expressions as well as the short-circuiting logic operators `&&` and `||` will become legal in all [const contexts](https://doc.rust-lang.org/reference/const_eval.html#const-context). A const context is any of the following: > > - The initializer of a `const`, `static`, `static mut` or enum discriminant. > - The body of a `const fn`. > - The value of a const generic (nightly only). > - The length of an array type (`[u8; 3]`) or an array repeat expression (`[0u8; 3]`). > > Furthermore, the short-circuiting logic operators will no longer be lowered to their bitwise equivalents (`&` and `|` respectively) in `const` and `static` initializers (see rust-lang#57175). As a result, `let` bindings can be used alongside short-circuiting logic in those initializers. Resolves rust-lang#49146. Ideally, we would resolve 🐳 rust-lang#66753 before this lands on stable, so it might be worth pushing this back a release. Also, this means we should get the process started for rust-lang#52000, otherwise people will have no recourse except recursion for iterative `const fn`. r? @oli-obk
…ch, r=oli-obk Stabilize `#![feature(const_if_match)]` Quoting from the [stabilization report](rust-lang#49146 (comment)): > `if` and `match` expressions as well as the short-circuiting logic operators `&&` and `||` will become legal in all [const contexts](https://doc.rust-lang.org/reference/const_eval.html#const-context). A const context is any of the following: > > - The initializer of a `const`, `static`, `static mut` or enum discriminant. > - The body of a `const fn`. > - The value of a const generic (nightly only). > - The length of an array type (`[u8; 3]`) or an array repeat expression (`[0u8; 3]`). > > Furthermore, the short-circuiting logic operators will no longer be lowered to their bitwise equivalents (`&` and `|` respectively) in `const` and `static` initializers (see rust-lang#57175). As a result, `let` bindings can be used alongside short-circuiting logic in those initializers. Resolves rust-lang#49146. Ideally, we would resolve 🐳 rust-lang#66753 before this lands on stable, so it might be worth pushing this back a release. Also, this means we should get the process started for rust-lang#52000, otherwise people will have no recourse except recursion for iterative `const fn`. r? @oli-obk
It's not possible to implement
Option::unwrap
as a const fn like:https://play.rust-lang.org/?version=nightly&mode=debug&edition=2018&gist=8ad0c1cdd371ca0488fab9d872bd6ffe
Because the function triggers this error:
The text was updated successfully, but these errors were encountered: