Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Auto merge of #140 - cjpearce:fix/test-race-condition, r=komaeda
Fix intermittent test failure caused by race condition First public pull request 😬 There's an intermittent integration test failure when you use multiple test threads (at least for me on a mac). I narrowed it down to two tests each spawning a process using `Command` which then try to compile the same file at the same time. If the timing doesn't work out, they both try to compile, and then one process runs `clean` before the other can run the executable - causing a panic. 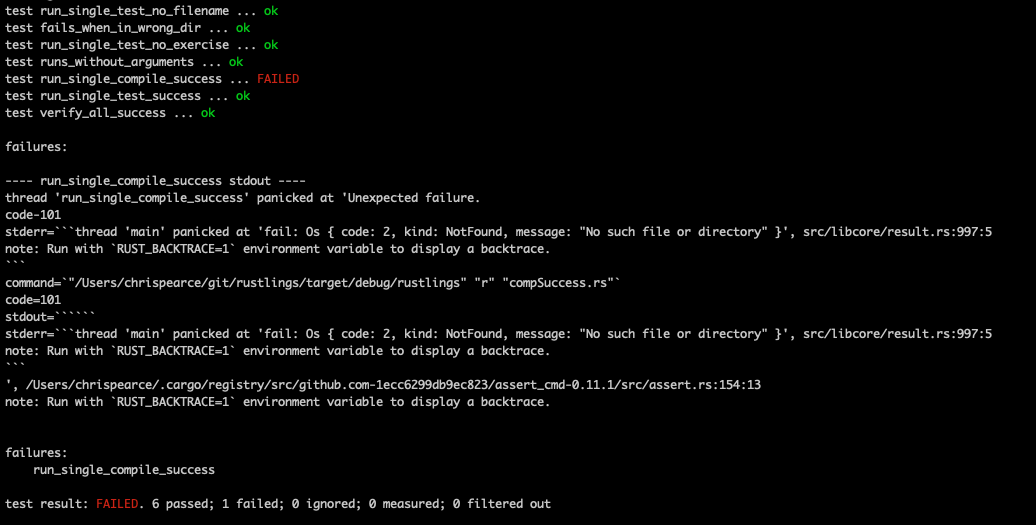 You can prevent it from happening by running with a single thread (`cargo test -- --test-threads=1`), because the `Command` blocks. That's not a particularly good solution though because it's not something you can configure in `Cargo.toml`. I considered making the affected tests just run serially, but it occurred to me that this could also happen if someone accidentally runs rustlings in watch mode in two terminals without realising it. I wound't consider this that unlikely given it's a tool for learning. I fixed it by ensuring that the executables made from separate processes don't conflict by appending a process id to the output executable name. I also extracted the commands into a single file next to `clean` so that we don't have to repeat the generated file name everywhere and risk missing something.
- Loading branch information
Showing
4 changed files
with
49 additions
and
30 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,12 +1,41 @@ | ||
use std::fs::remove_file; | ||
use std::process::{self, Command, Output}; | ||
|
||
const RUSTC_COLOR_ARGS: &[&str] = &["--color", "always"]; | ||
|
||
fn temp_file() -> String { | ||
format!("./temp_{}", process::id()) | ||
} | ||
|
||
pub fn compile_test_cmd(filename: &str) -> Output { | ||
Command::new("rustc") | ||
.args(&["--test", filename, "-o", &temp_file()]) | ||
.args(RUSTC_COLOR_ARGS) | ||
.output() | ||
.expect("failed to compile exercise") | ||
} | ||
|
||
pub fn compile_cmd(filename: &str) -> Output { | ||
Command::new("rustc") | ||
.args(&[filename, "-o", &temp_file()]) | ||
.args(RUSTC_COLOR_ARGS) | ||
.output() | ||
.expect("failed to compile exercise") | ||
} | ||
|
||
pub fn run_cmd() -> Output { | ||
Command::new(&temp_file()) | ||
.output() | ||
.expect("failed to run exercise") | ||
} | ||
|
||
pub fn clean() { | ||
let _ignored = remove_file("temp"); | ||
let _ignored = remove_file(&temp_file()); | ||
} | ||
|
||
#[test] | ||
fn test_clean() { | ||
std::fs::File::create("temp").unwrap(); | ||
std::fs::File::create(&temp_file()).unwrap(); | ||
clean(); | ||
assert!(!std::path::Path::new("temp").exists()); | ||
assert!(!std::path::Path::new(&temp_file()).exists()); | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters