-
-
Notifications
You must be signed in to change notification settings - Fork 1.3k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
## Description I added the possibility to use one instance of `useAnimationStyle()` for many components. `AnimationStyle` is connected with view by ViewDescriptor object. I changed this to a set of `ViewDescriptors` and now `AnimationStyle` can be shared between many components. Fixes #1263 ## Example of code <details> <summary>code</summary> ```Js import Animated, { useSharedValue, withTiming, useAnimatedStyle, Easing, } from 'react-native-reanimated'; import { Button, ScrollView } from 'react-native'; import React from 'react'; export default function SharedAnimatedStyleUpdateExample(props) { const randomWidth = useSharedValue(10); const config = { duration: 500, easing: Easing.bezier(0.5, 0.01, 0, 1), }; const style = useAnimatedStyle(() => { return { width: withTiming(randomWidth.value, config), }; }); const renderList = () => { const items = []; for (let i = 0; i < 100; i++) { items.push( <Animated.View key={i} style={[ { width: 100, height: 5, backgroundColor: 'black', margin: 1 }, style, ]} /> ); } return items; }; return ( <ScrollView style={{ flex: 1, flexDirection: 'column', }}> <Button title="toggle" onPress={() => { randomWidth.value = Math.random() * 350; }} /> {renderList()} </ScrollView> ); } ``` </details> <details> <summary>code2</summary> ```Js import Animated, { useSharedValue, withTiming, useAnimatedStyle, Easing, } from 'react-native-reanimated'; import { View, Button } from 'react-native'; import React, { useState } from 'react'; export default function AnimatedStyleUpdateExample(props) { const randomWidth = useSharedValue(10); const [counter, setCounter] = useState(0); const [counter2, setCounter2] = useState(0); const [itemList, setItemList] = useState([]); const [toggleState, setToggleState] = useState(false); const config = { duration: 500, easing: Easing.bezier(0.5, 0.01, 0, 1), }; const style = useAnimatedStyle(() => { return { width: withTiming(randomWidth.value, config), }; }); const staticObject = <Animated.View style={[ { width: 100, height: 8, backgroundColor: 'black', margin: 1 }, style, ]} /> const renderItems = () => { let output = [] for(let i = 0; i < counter; i++) { output.push( <Animated.View key={i + 'a'} style={[ { width: 100, height: 8, backgroundColor: 'blue', margin: 1 }, style, ]} /> ) } return output } return ( <View style={{ flex: 1, flexDirection: 'column', }}> <Button title="animate" onPress={() => { randomWidth.value = Math.random() * 350; }} /> <Button title="increment counter" onPress={() => { setCounter(counter + 1) }} /> <Button title="add item to static lists" onPress={() => { setCounter2(counter2 + 1) setItemList([...itemList, <Animated.View key={counter2 + 'b'} style={[ { width: 100, height: 8, backgroundColor: 'green', margin: 1 }, style, ]} />]) }} /> <Button title="toggle state" onPress={() => { setToggleState(!toggleState) }} /> <Animated.View style={[ { width: 100, height: 8, backgroundColor: 'orange', margin: 1 }, style, ]} /> {toggleState && <Animated.View style={[ { width: 100, height: 8, backgroundColor: 'black', margin: 1 }, style, ]} />} {toggleState && staticObject} {renderItems()} {itemList} </View> ); } ``` </details> ## Change before changes: 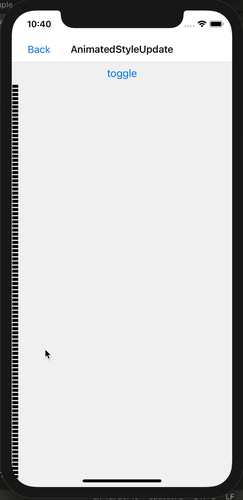 final result: 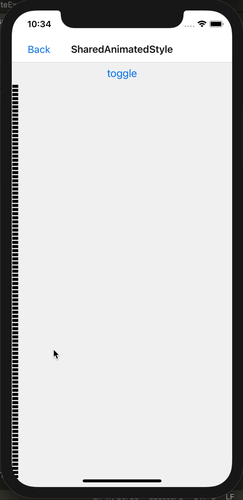
- Loading branch information
Showing
15 changed files
with
248 additions
and
112 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.