Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add tests & slight refactor to TelegramTransport key handling (#528)
This PR: 1. Adds a few additional tests to TelegramTransport 2. Improves TelegramTransport's handling of dynamically set keys (it already had this; this PR makes it a bit more robust) This will enable a few nice things form a user perspective: 1. Users can re-bind an Agent via the Web UI to a new Telegram Bot 2. Users can defer binding their Agent to a Telegram Bot until after they've created an instance Follow-on work is a PR to do for Telegram what we just did for Slack in the web UI: 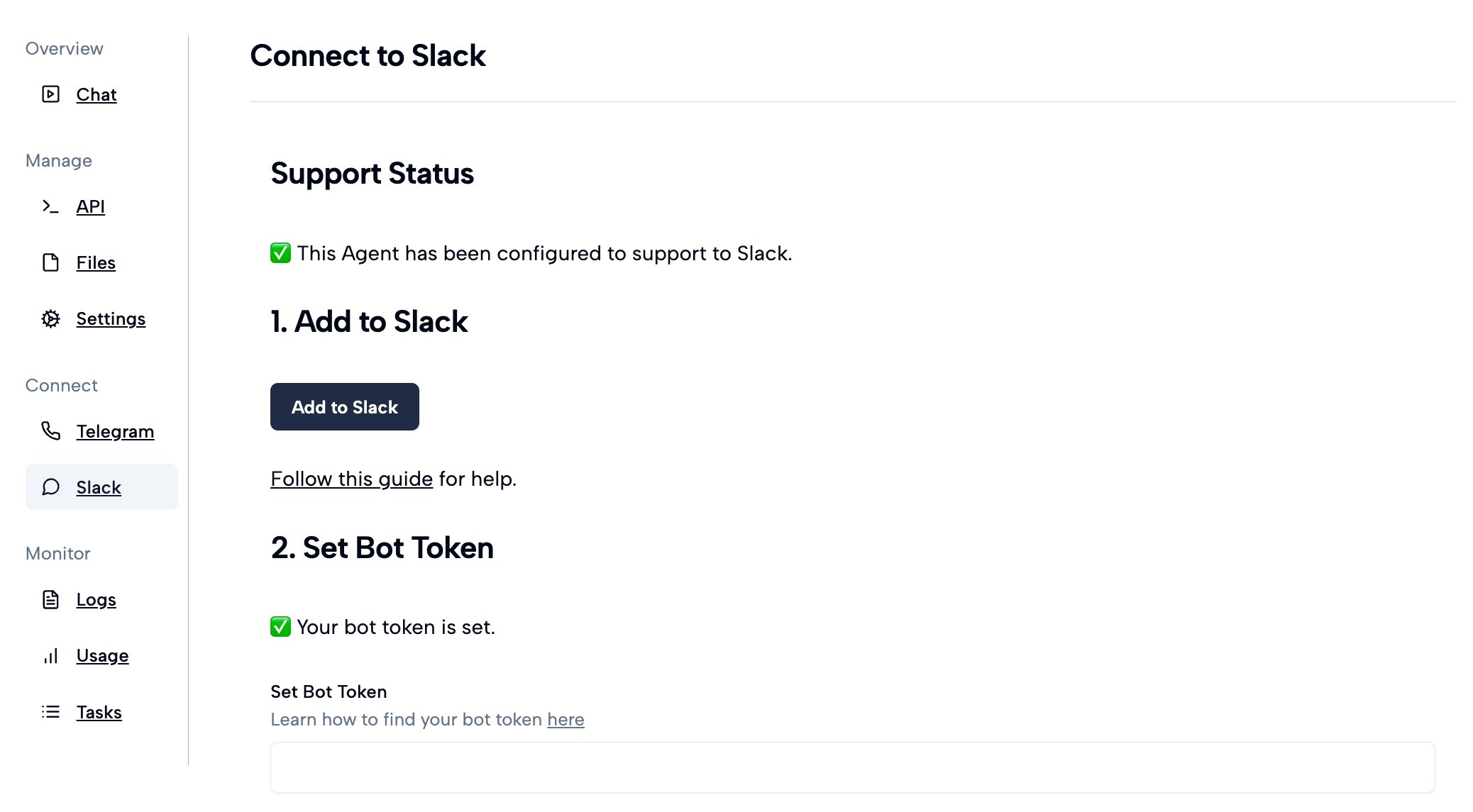
- Loading branch information
Showing
10 changed files
with
264 additions
and
63 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.