Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
bug #48897 [Console] fix clear of section with question (maxbeckers)
This PR was merged into the 6.2 branch. Discussion ---------- [Console] fix clear of section with question | Q | A | ------------- | --- | Branch? | 6.2 | Bug fix? | yes | New feature? | no | Deprecations? | no | Tickets | Fix #47411 | License | MIT | Doc PR | n/a This PR fixes the problems to clear a section with a question included. Example Code: ``` protected function execute(InputInterface $input, OutputInterface $output) { $section1 = $output->section(); $io = new SymfonyStyle($input, $section1); $output->writeln("foo"); $countdown = 3; while ($countdown > 0) { $section1->clear(); $io->writeln('start ' . $countdown); $io->write('foo'); $io->write(' and bar'.\PHP_EOL); $givenAnswer = $io->ask('Dummy question?'); $section1->write('bar'); $countdown--; } return self::SUCCESS; } ``` Output loop 1: 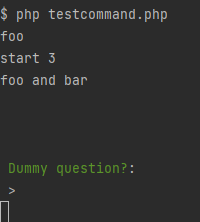 Output loop 1: 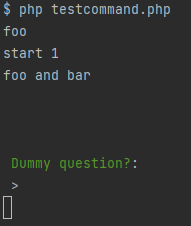 There was already a fix #48089 to be merged in 6.1, but the problem was that there were some changes in 6.2, so it was not possible to merge it into 6.2. So this fix is only working for 6.2, but perhaps we could find a solution as well for the older versions. But because of the changes of console it was not possible to find a solution working for all versions. `@chalasr` this fix is still with the newline always `true` https://github.com/symfony/symfony/blob/4cf9855debc26e4323429ac8d87f02df582e2893/src/Symfony/Component/Console/Output/ConsoleSectionOutput.php#L181 A change of the newline to `$newline` would change the behavior. Maybe we could change that in symfony 7. To make it easier to test is here a zip with 2 testcommands in the root and the changed vendors. [test-48089.zip](https://github.com/symfony/symfony/files/10360423/test-48089.zip) Commits ------- f4c551805b [Console] fix clear of section with question
- Loading branch information