-
Notifications
You must be signed in to change notification settings - Fork 6
Zippie Button
Want to unshackle your dApp from clunky onboarding solutions? Want to have a consistent experience no matter where your users are coming from?
Follow these instructions to include the 'Sign in with Zippie' button
Install the zippie modules from node repostiory
npm install @zippie/vault-api
npm install @zippie/vault-web3-provider
Import the modules into your DApp
import Vault from '@zippie/vault-api'
import * as vaultSecp256k1 from '@zippie/vault-api/src/secp256k1.js'
import * as zippieprovider from '@zippie/vault-web3-provider'
import the button graphics, you get a choice of light or dark themed buttons
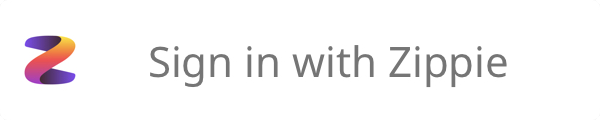

import zippieButtonDark from '@zippie/vault-api/static/img/zippie-button-dark-default.png'
import zippieButtonLight from '@zippie/vault-api/static/img/zippie-button-light-default.png'
you will need something like file-loader to make sure the graphics are packed into your deployment
Add a spot for the button in the HTML
<div id='zippie-button' />
When the button is pressed, the user will be asked to onboard if they haven't already and then be returned to your dApp.
Check for the presence of the zippie login cookie, and automatically initialise the zippie vault and start your dApp
const vault = new Vault({vault_uri: 'https://vault.dev.zippie.org/'})
if (localStorage.getItem('zippie-vault-cookie')) {
vault.setup()
.then(isSignedIn => {
if (isSignedIn) { onSignedIn() }
})
.catch(e => { console.error("Failed to initialize vault:", e) })
}
function onSignedIn () {
let ethereum = zippieprovider.init(vault, vaultSecp256k1, { network: 'kovan' })
window.web3 = new Web3(ethereum)
// Hide button
zippieBtn.style.display = 'none'
}
Handle Zippie signin button clicks. If the user has a Zippie account already registered on the device, it will automatically signin the dapp. Otherwise it will cause a Zippie sign up process and when the user creates a new account, will redirect back to your dapp.
const zippieBtn = document.getElementById('zippie-button')
function doZippieSignin (inhibit_signup) {
return ev => {
vault.setup()
.then(_ => vault.signin({}, inhibit_signup))
.then(onSignedIn)
.catch(e => { console.error("Failed to signin vault:", e) })
}
}
zippieBtn.addEventListener('click', doZippieSignin())
If you would like to inhibit for some reason the signin button to only do sign ins, and not automatically start a signup process. Then it is possible to do this by changing the above button handler registration to:
zippieBtn.addEventListener('click', doZippieSignin(true))
Check out the documentation on github at: