New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Code() in file Code_mobject.py to display code with color highlighted works correctly with updates in text_mobject.py and svg_mobject. #1018
Conversation
|
Solved ! |
now everything is working fine. |
Only changes I would make is 1.)adding CodeMobject to imports.py 2.) changing some of the variable names to more meaningful things I've noticed you used letters alot which may be confusing to someone who may come about this file in the future 3.)also there seems to be an issue on line 125 otherwise it looks good maybe we can try implementing the conversion of the HTML for the user so they don't need to rewrite code and convert it to a readable version for Manim, instead I'm proposing that we allow the user to add any file type to assets/codes and then we convert for them |
updated ! |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Dude awesome work, lets try and get a code converter working to make code_mobject even better!
implementing the conversion of the HTML do you mean user input .cpp file or any other and it automatically converts it to html ? i don't found any better way to do that, i tried pygments but it have only few coding languages options and sometimes don't highlight correctly. do you have any other better option? |
well i can implement that online highlighter in python then it send request to the server and get highlighted html |
change two files "svg_mobjet.py" and "text_mobject.py" |
i think its better if a single PR is solving two problems. |
as you said "delete |
Yeah, that's true. I understand. |
You can create another one if your PR is merged then this PR will automatically updated and don't show up that text_mobject.py or svg_mobject.py changes in files changes. |
@NavpreetDevpuri oops, I think you did a wrong thing, you merge my pull request which in the shaders branch but not master. The pull request of master branch is #1030 which is already merged but not 1031 which is waiting for merged. |
See #1035 |
@eulertour check updates. |
Please do not try to build a text layout engine by yourself. And letting Text receive multiple texts is a good idea, but this change(feature) is already big enough that you should open a single PR specifically focus on this thing. Your PR have 48 commits, honestly I think it's too big to be a single PR. |
Also, SingleStringTextMobject is not a good choice. |
i just make each line as a separate text i don't changing or doing anything with 'the space between the letters' |
Sorry, I said wrong. I mean the space in the front and back of each line. |
no problem |
reopened with only 6 commits |
* Fix x_min, x_max and color of FunctionGraph * Add graphical unit test to FunctionGraph * Apply review suggestions * Pass color as argument instead of updating kwargs and run black
you can use it as follow.
if you put file into "assets/codes" folder then you don't need to specify full file path, just name of file is enough otherwise specify full file path.
if generate_html_file==True
it create a html file with color highlighted and save it to "assets/codes/generated_html_files/"Output will be looks like
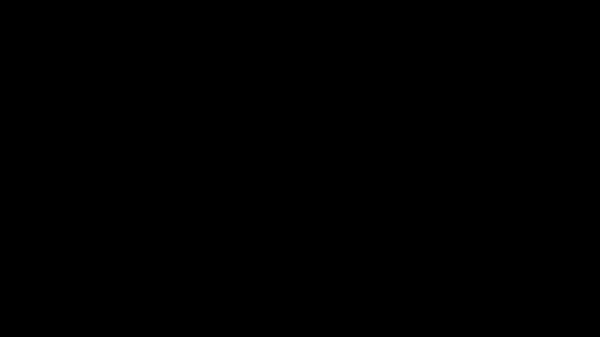
Updated text_mobject() to use Text() as paragraph.
Output
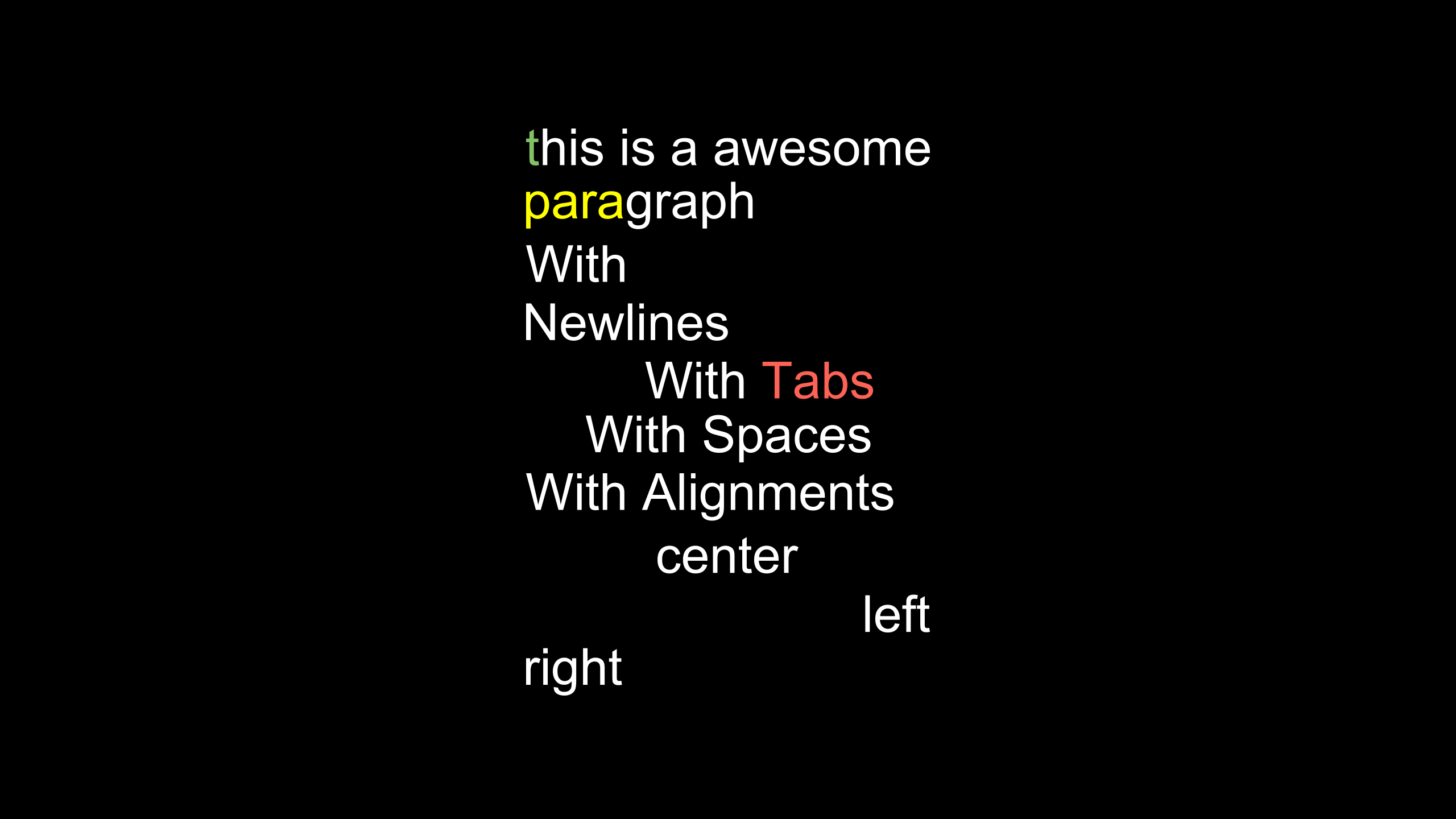
It solves the following problems