-
Notifications
You must be signed in to change notification settings - Fork 1
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Using p5.js in Node runtime #1
Comments
Example Discord Bot using p5.js: DiscordP5CanvasP.S. There is an issue with using this method of p5, whenever any kind of error is thrown, (undefined variable/invalid syntax, etc) in the sketch, only this error will show in the console:
I don't have any idea on what is causing this error, as I have not checked the JSDOM source code out. |
CodingTrain/Random-Whistle ported to node: here |
@dipamsen Great job! I worked on this over the weekend and got pretty close but I don't think I would have gotten there. Thanks for sharing this. I hope to use this to output p5.js sketches to pixel arrays using a raspberry pi. |
TL;DR: scroll down to the bottom for the templates
After studying the source code of
p5.js
, this is what I have gathered so far:p5 Instance Mode
For making p5 work in instance mode I created this template:
In this way, p5.js can be used in Instance Mode to work with
node-canvas
Click here to see the codes for
saveAsPNG
andsetupWindow
:saveAsPNG
- Saves the canvas Image to filname using fssetupWindow
- Add necessary variables toglobal
for p5 to runGoing further - Global Mode!
This is the template for using p5.js in node with Global Mode:
In this case as well, the functions
saveAsPNG
andsetupWindow
should be appended at the end of the file, with the last two lines ofsetupWindow
uncommented.This is the output of the global mode code
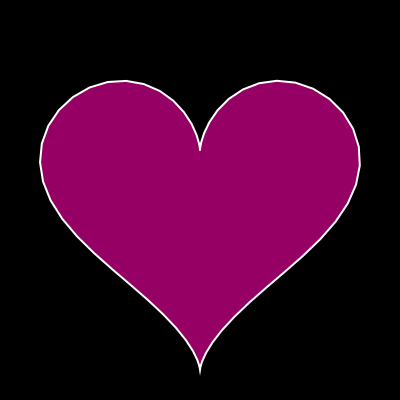
Use these Templates!
To Use these templates, first install these node-modules
npm i p5 canvas jsdom
node-canvas
withp5.js
in global modenode-canvas
withp5.js
in instance modeThe text was updated successfully, but these errors were encountered: