You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
// no-default-export-css-modules.jsmodule.exports={meta: {messages: {noDefaultExport: 'Do not use default export of css modules'}},create(context){return{ImportDefaultSpecifier(node){constisCssModule=isCssModulesFile(context,node.parent.source.value)if(isCssModule){context.report({
node,messageId: 'noDefaultImport'})}}}}}
前段时间对项目升级了 webpack5 后,发现 css-loader 中 css-modules 的配置有一些 breakchange。笔者为这些不兼容的语法开发了一个 eslint 插件来校验,源码见 eslint-plugin-css-modules-es
What changes
默认开启了
esModule
。提供
namedExport
配置,值为true
时,exportLocalsConvention
的值只能为camelCaseOnly
。在老版本中,我们使用的是
camelCase: true
配置,我们先来看camelCaseOnly
与camelCase
的区别。举例:
老版本编译出来的对象为:
新版本编译出来的对象为:
可以看出,原先代码中的几个用法,在升级后均会出现问题
再来看 JavaScript 保留字的 breakchange。为什么不能使用保留字作为类目?我们来看下面的例子
在将上述代码编译成 es 模块时,相当于以下代码
在严格模式下,显然都是不行的
How to resolve
我们要先让所有使用
css-modules
默认导入的代码都抛出错误,因此只要自定义一个eslint
规则来校验即可,代码比较简单,这里就直接贴出来了camelCaseOnly
跟默认导入的思路一样,我们可以自定义一个
eslint
规则,这里需要处理具名导入和命名空间导入两种情况如上,解析出来的 AST 对应为
ImportSpecifier
,但要注意导入时可以重命名在 AST 中
delete_icon
对应node.imported
,deleteIcon
对应node.local
,因此我们应该判断node.imported
的值,而非node.local
。核心代码
命名空间导入的校验相对复杂一点,因为我们需要找出所有引用命名空间变量的代码,判断是否有使用非驼峰化的类名。而且需要注意的是,如果原先类命名使用了破折号
-
,那么使用的时候就不是style.a-b
,而是style['a-b']
,因此这两种逻辑都需要处理。(笔者项目上没有使用动态生成类名,如 style[a-${someVariable}
],因此忽略这种情况)核心代码
若使用了保留字,webpack 编译模块的时候就会报错,因此就不需要自定义额外的 eslint 规则来校验。
运行结果:
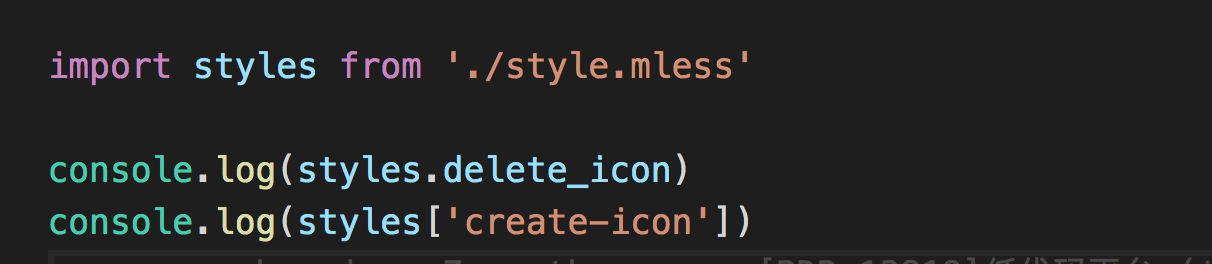

结语
笔者第一次实践自定义 eslint,还是蛮有趣的。目前插件还比较简陋,没有实现自动修复功能,感兴趣的读者可以试试,欢迎贡献 pr。
后文
The text was updated successfully, but these errors were encountered: