-
Notifications
You must be signed in to change notification settings - Fork 31
security
- Overview
-
Categories
- A01:2021-Broken Access Control
- A02:2021-Cryptographic Failures
- A03:2021-Injection
- A04:2021-Insecure Design
- A05:2021-Security Misconfiguration
- A06:2021-Vulnerable and Outdated Components
- A07:2021-Identification and Authentication Failures
- A08:2021-Software and Data Integrity Failures
- A09:2021-Security Logging and Monitoring Failures
- A10:2021-Server-Side Request Forgery
- SonarQube
- References
The OWASP Top 10 is a standard awareness document for web application security and developers. It reflects widespread agreement on the most serious security threats to web applications.
In the Top 10 for 2021, there are three new categories, four with nomenclature and scoping modifications, and some consolidation.
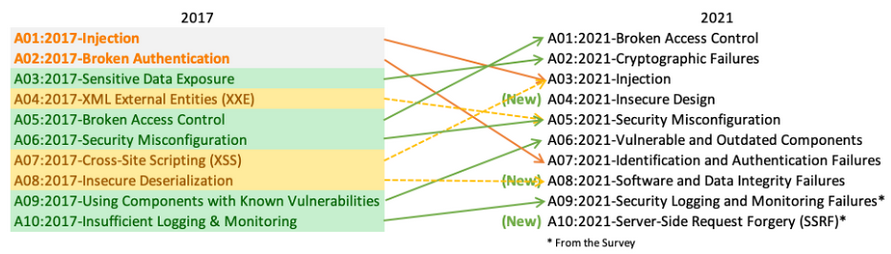
Name |
Description |
A01:2021-Broken Access Control |
Access control ensures that users do not exceed their intended permissions. |
A02:2021-Cryptographic Failures |
Cryptographic failures (or lack thereof), which frequently result in the exposing of sensitive data |
A03:2021-Injection |
This category includes |
A04:2021-Insecure Design |
Missing or ineffective control design. |
A05:2021-Security Misconfiguration |
Including unnecessary features enabled or installed, default accounts and their passwords enabled and unchanged, among other vulnerabilities |
A06:2021-Vulnerable and Outdated Components |
Every corporation must have a continuous process in place for monitoring, triaging, and implementing updates or configuration changes during the life of the application. |
A07:2021-Identification and Authentication Failures |
To prevent against authentication-related threats, user identity verification, authentication, and session management are crucial. |
A08:2021-Software and Data Integrity Failures |
Failures in software and data integrity are caused by code and infrastructure that do not prevent against integrity violations. |
A09:2021-Security Logging and Monitoring Failures |
Breach detection is unachievable without logging and monitoring. |
A10:2021-Server-Side Request Forgery |
SSRF issues occur when a web application retrieves a remote resource without verifying the user-supplied URL. |
Access control, also commonly referred to as authorization, is a set of mechanisms and policies that manage access over resources. Usually, once the server has determined your credentials using an authentication mechanism, it will then grant or restrict what resources you can access in the system.
Access control is only effective in trusted server-side code or server-less API, where the attacker cannot modify the access control check or metadata.
-
Except for public resources, deny by default.
-
Implement access control mechanisms once and re-use them throughout the application, including minimizing Cross-Origin Resource Sharing (CORS) usage.
-
Model access controls should enforce record ownership rather than accepting that the user can create, read, update, or delete any record.
-
Unique application business limit requirements should be enforced by domain models.
-
Disable web server directory listing and ensure file metadata (e.g., .git) and backup files are not present within web roots.
-
Log access control failures, alert admins when appropriate (e.g., repeated failures).
-
Rate limit API and controller access to minimize the harm from automated attack tooling.
-
Stateful session identifiers should be invalidated on the server after logout. Stateless JWT tokens should rather be short-lived so that the window of opportunity for an attacker is minimized. For longer lived JWTs it’s highly recommended to follow the OAuth standards to revoke access.
In devon4net we have the Devon4Net.Infrastructure.JWT
component available. This component allows developers restrict access to different functionalities using a Json Web Token. This token has encrypted information, a time-to-live period, and can be verified, refreshed and discarded using cryptographic functions.
This JWT verification occurs in the backend of the application so it cannot be bypassed easily.
In addition, a developer that uses this component will have to come up with a secure sign in method that provides this token to the application client.
Devon implements Devon4Net.Infrastructure.CORS
, which allows control over cross-origin resources.
The user can set it to allow the required hosts.
CORS policies can also be defined by method, class, or globally.
There is a pre-configured policy that accepts any origins by default; the program will alert you about it, and it is not recommended in production environments.
The first step is to identify the security requirements for data in transit and at rest. Passwords, credit card numbers, health records, personal information, and business secrets, for example, require additional safeguards, particularly if the data is subject to privacy regulations.
Do the following, at a minimum, and consult the references:
-
Classify data processed, stored, or transmitted by an application. Identify which data is sensitive according to privacy laws, regulatory requirements, or business needs.
-
Don’t store sensitive data unnecessarily. Discard it as soon as possible or use PCI DSS compliant tokenization or even truncation. Data that is not retained cannot be stolen.
-
Make sure to encrypt all sensitive data at rest.
-
Ensure up-to-date and strong standard algorithms, protocols, and keys are in place; use proper key management.
-
Encrypt all data in transit with secure protocols such as TLS with forward secrecy (FS) ciphers, cipher prioritization by the server, and secure parameters. Enforce encryption using directives like HTTP Strict Transport Security (HSTS).
-
Disable caching for response that contain sensitive data.
-
Apply required security controls as per the data classification.
-
Do not use legacy protocols such as FTP and SMTP for transporting sensitive data.
-
Store passwords using strong adaptive and salted hashing functions with a work factor (delay factor), such as Argon2, scrypt, bcrypt or PBKDF2.
-
Initialization vectors must be chosen appropriate for the mode of operation. For many modes, this means using a CSPRNG (cryptographically secure pseudo random number generator). For modes that require a nonce, then the initialization vector (IV) does not need a CSPRNG. In all cases, the IV should never be used twice for a fixed key.
-
Always use authenticated encryption instead of just encryption.
-
Keys should be generated cryptographically randomly and stored in memory as byte arrays. If a password is used, then it must be converted to a key via an appropriate password base key derivation function.
-
Ensure that cryptographic randomness is used where appropriate, and that it has not been seeded in a predictable way or with low entropy. Most modern APIs do not require the developer to seed the CSPRNG to get security.
-
Avoid deprecated cryptographic functions and padding schemes, such as MD5, SHA1, PKCS number 1 v1.5 .
-
Verify independently the effectiveness of configuration and settings.
Although HTTP communication protocol can be used between the server and the client, HTTPS is highly recommended. In devon4net you can easily configure certificates for secure communication with latest public key cryptographic algorithms.
Some of the more common injections are SQL, NoSQL, OS command, Object Relational Mapping (ORM), LDAP, and Expression Language (EL) or Object Graph Navigation Library (OGNL) injection.
Preventing injection requires keeping data separate from commands and queries:
-
The preferred option is to use a safe API, which avoids using the interpreter entirely, provides a parameterized interface, or migrates to Object Relational Mapping Tools (ORMs).
NoteEven when parameterized, stored procedures can still introduce SQL injection if PL/SQL or T-SQL concatenates queries and data or executes hostile data with EXECUTE IMMEDIATE or exec(). -
Use positive server-side input validation. This is not a complete defense as many applications require special characters, such as text areas or APIs for mobile applications.
-
For any residual dynamic queries, escape special characters using the specific escape syntax for that interpreter.
NoteSQL structures such as table names, column names, and so on cannot be escaped, and thus user-supplied structure names are dangerous. This is a common issue in report-writing software. -
Use LIMIT and other SQL controls within queries to prevent mass disclosure of records in case of SQL injection.
Entity Framework Core is a modern object-database mapper for .NET. It supports LINQ queries, change tracking, updates, and schema migrations. By using EntityFramework we are using parametrized queries, which are a big protection against SQL injection attacks for example.
Insecure design is a broad category representing different weaknesses, expressed as “missing or ineffective control design.” One factor that contributes to insecure design is a lack of business risk profile inherent in the software or system being produced, which results in a failure to decide what level of security design is necessary.
-
Establish and use a secure development lifecycle with AppSec professionals to help evaluate and design security and privacy-related controls
-
Establish and use a library of secure design patterns or paved road ready to use components
-
Use threat modeling for critical authentication, access control, business logic, and key flows
-
Integrate security language and controls into user stories
-
Integrate plausibility checks at each tier of your application (from frontend to backend)
-
Write unit and integration tests to validate that all critical flows are resistant to the threat model. Compile use-cases and misuse-cases for each tier of your application.
-
Segregate tier layers on the system and network layers depending on the exposure and protection needs
-
Segregate tenants robustly by design throughout all tiers
-
Limit resource consumption by user or service
Secure installation processes should be implemented, including:
-
A repeatable hardening process makes it fast and easy to deploy another environment that is appropriately locked down. Development, QA, and production environments should all be configured identically, with different credentials used in each environment. This process should be automated to minimize the effort required to set up a new secure environment.
-
A minimal platform without any unnecessary features, components, documentation, and samples. Remove or do not install unused features and frameworks.
-
A task to review and update the configurations appropriate to all security notes, updates, and patches as part of the patch management process (see A06:2021-Vulnerable and Outdated Components). Review cloud storage permissions (e.g., S3 bucket permissions).
-
A segmented application architecture provides effective and secure separation between components or tenants, with segmentation, containerization, or cloud security groups (ACLs).
-
Sending security directives to clients, e.g., Security Headers.
-
An automated process to verify the effectiveness of the configurations and settings in all environments.
Devon4net is configurable and has many packages that can be adjusted to your needs through a configuration file.
This file can have variations for each available environment, for example, we can have a configuration file for the production environment appsettings.Production.json
and another for development appsettings.Development.json
, each with different configuration.
Thanks to .Net we can also specify some code for different environments. For example, maybe we want to add a default user if the environment is Development so we can do our testing. We can specify the code for adding this user with the condition of the environment being Development and this code wont be compiled in other enviroment than the specified.
This provides the application with security because we treat each environment differently, increasing security in released versions.
There should be a patch management process in place to:
-
Remove unused dependencies, unnecessary features, components, files, and documentation.
-
Continuously inventory the versions of both client-side and server-side components (e.g., frameworks, libraries) and their dependencies using tools like versions, OWASP Dependency Check, retire.js, etc. Continuously monitor sources like Common Vulnerability and Exposures (CVE) and National Vulnerability Database (NVD) for vulnerabilities in the components. Use software composition analysis tools to automate the process. Subscribe to email alerts for security vulnerabilities related to components you use.
-
Only obtain components from official sources over secure links. Prefer signed packages to reduce the chance of including a modified, malicious component (See A08:2021-Software and Data Integrity Failures).
-
Monitor for libraries and components that are unmaintained or do not create security patches for older versions. If patching is not possible, consider deploying a virtual patch to monitor, detect, or protect against the discovered issue.
In our framework, we keep all of the libraries and components up to date, constantly evaluating and monitoring all of our libraries searching for vulnerabilities and updated versions, and we don’t have any unnecessary dependencies. Every time we modify our code, we use SonarQube to check the code quality and vulnerabilities.
Confirmation of the user’s identity, authentication, and session management is critical to protect against authentication-related attacks.
-
Where possible, implement multi-factor authentication to prevent automated credential stuffing, brute force, and stolen credential reuse attacks.
-
Do not ship or deploy with any default credentials, particularly for admin users.
-
Implement weak password checks, such as testing new or changed passwords against the top 10,000 worst passwords list.
-
Align password length, complexity, and rotation policies with National Institute of Standards and Technology (NIST) 800-63b’s guidelines in section 5.1.1 for Memorized Secrets or other modern, evidence-based password policies.
-
Ensure registration, credential recovery, and API pathways are hardened against account enumeration attacks by using the same messages for all outcomes.
-
Limit or increasingly delay failed login attempts, but be careful not to create a denial of service scenario. Log all failures and alert administrators when credential stuffing, brute force, or other attacks are detected.
-
Use a server-side, secure, built-in session manager that generates a new random session ID with high entropy after login. Session identifier should not be in the URL, be securely stored, and invalidated after logout, idle, and absolute timeouts.
Software and data integrity failures relate to code and infrastructure that does not protect against integrity violations. An example of this is where an application relies upon plugins, libraries, or modules from untrusted sources, repositories, and content delivery networks (CDNs).
-
Use digital signatures or similar mechanisms to verify the software or data is from the expected source and has not been altered.
-
Ensure libraries and dependencies, such as npm or Maven, are consuming trusted repositories. If you have a higher risk profile, consider hosting an internal known-good repository that’s vetted.
-
Ensure that a software supply chain security tool, such as OWASP Dependency Check or OWASP CycloneDX, is used to verify that components do not contain known vulnerabilities
-
Ensure that there is a review process for code and configuration changes to minimize the chance that malicious code or configuration could be introduced into your software pipeline.
-
Ensure that your CI/CD pipeline has proper segregation, configuration, and access control to ensure the integrity of the code flowing through the build and deploy processes.
-
Ensure that unsigned or unencrypted serialized data is not sent to untrusted clients without some form of integrity check or digital signature to detect tampering or replay of the serialized data
This category is to help detect, escalate, and respond to active breaches. Without logging and monitoring, breaches cannot be detected.
-
Ensure all login, access control, and server-side input validation failures can be logged with sufficient user context to identify suspicious or malicious accounts and held for enough time to allow delayed forensic analysis.
-
Ensure that logs are generated in a format that log management solutions can easily consume.
-
Ensure log data is encoded correctly to prevent injections or attacks on the logging or monitoring systems.
-
Ensure high-value transactions have an audit trail with integrity controls to prevent tampering or deletion, such as append-only database tables or similar.
-
DevSecOps teams should establish effective monitoring and alerting such that suspicious activities are detected and responded to quickly.
-
Establish or adopt an incident response and recovery plan, such as National Institute of Standards and Technology (NIST) 800-61r2 or later.
In devon we have the Devon4Net.Infrastructure.Logger component that allows developers to log all the information that they need. It can be organiced in different log levels according to its purpose, for example you can log debugging information or critical errors, into a file or a database. Furthermore all the devon4net components have their own logging implemented.
SSRF flaws occur whenever a web application is fetching a remote resource without validating the user-supplied URL. It allows an attacker to coerce the application to send a crafted request to an unexpected destination, even when protected by a firewall, VPN, or another type of network access control list (ACL).
-
From Network layer
-
Segment remote resource access functionality in separate networks to reduce the impact of SSRF
-
Enforce “deny by default” firewall policies or network access control rules to block all but essential intranet traffic.
-
-
From Application layer:
-
Sanitize and validate all client-supplied input data
-
Enforce the URL schema, port, and destination with a positive allow list
-
Do not send raw responses to clients
-
Disable HTTP redirections
-
Be aware of the URL consistency to avoid attacks such as DNS rebinding and “time of check, time of use” (TOCTOU) race conditions
-
SonarQube is a tool for automatically detecting bugs, vulnerabilities, and code smells in your code. It may be integrated into your existing workflow to allow for continuous code analysis across project branches and pull requests.
It covers also security by raising OWASP Top 10-related issues to developers early in the process, SonarQube helps you protect your systems, your data and your users.
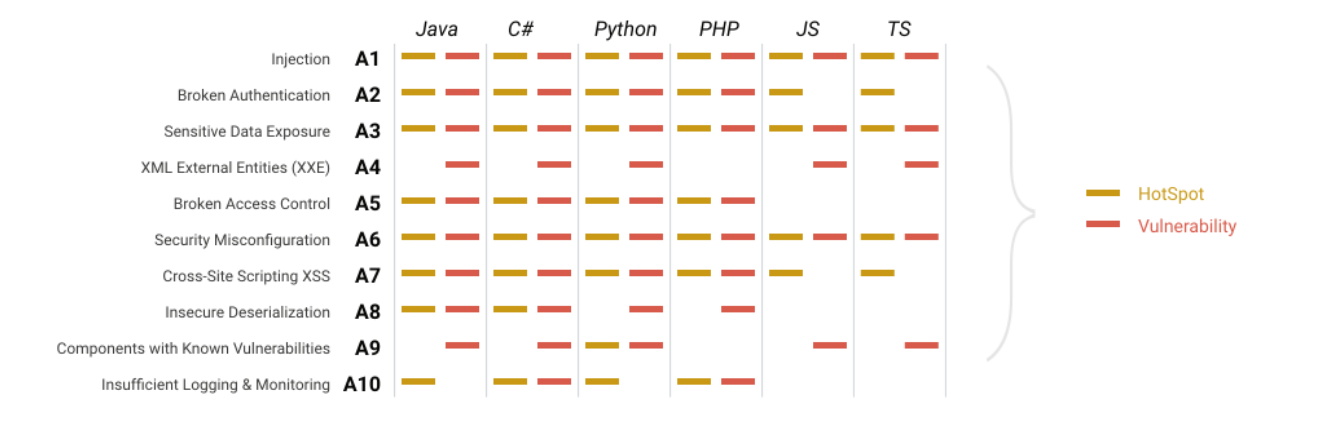
This documentation is licensed under the Creative Commons License (Attribution-NoDerivatives 4.0 International).