-
Notifications
You must be signed in to change notification settings - Fork 367
Cosmos configuration
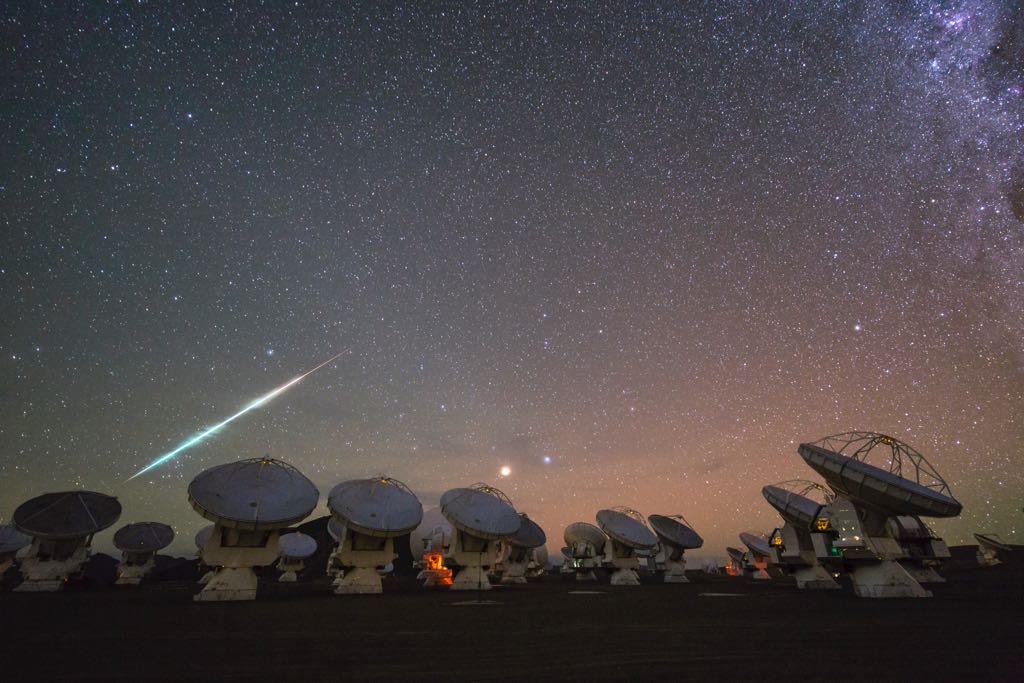
Atacama Large Millimeter Array. The photo was taken by Christoph Malin. Source: European Southern Observatory.
One can configure Cosmos view directly from the storyboard using the attributes inspector (not available with Carthage setup). Alternatively, all the configuration options are accessible through the settings
property of the view object.
// Set the color of a filled star
cosmosView.settings.filledColor = UIColor.orange
Star settings describe appearance of individual stars in the Cosmos.
-
emptyBorderColor: Border color of an empty star. Default:
#FF9500
color. - emptyBorderWidth: Width of the border for empty star. Default: 1 pixel.
- emptyColor: Background color of an empty star. Default: transparent.
-
filledBorderColor: Border color of a filled star. Default:
#FF9500
color. - filledBorderWidth: Width of the border for a filled star. Default: 1 pixel.
-
filledColor: Background color of a filled star. Default:
#FF9500
color. -
fillMode: Defines how the star is filled when the rating value is not a whole integer (
.full
,.half
,.precise
). It can either show full stars, half stars or stars partially filled according to the rating value. Default:.full
. When using in interface builder use these numbers:full
=0
,half
=1
,precise
=2
-
starMargin: Distance between stars. Default:
5
. - starPoints: Array of points for drawing the star with size of 100 by 100 pixels. Supply your points if you need to draw a different shape.
-
starSize: Size of a single star. Default:
20
. -
totalStars: The maximum number of stars to be shown. Default:
5
.
Use the text settings to style the optional text that is shown on the right edge of the Cosmos.
-
textColor: Color of the text. Default:
#7F7F7F
color. -
textFont: Font for the text. Default: dynamic type with
UIFontTextStyleFootnote
style. -
textMargin: Distance between the text and the stars. Default:
5
.
Supply images for the filled and empty stars. By default the star is drawn from the array of points unless an image is supplied.
-
filledImage: Image used for the filled portion of the star. Default:
nil
. -
emptyImage: Image used for the empty portion of the star. Default:
nil
.
Touch settings describe the behaviour of Cosmos when used as in input.
-
minTouchRating: The lowest rating that user can set by touching the stars. Default:
1
. -
passTouchesToSuperview: Set to
false
if you don't want the Cosmos view to pass touches to its superview. Can be useful for table view. Default:true
. -
updateOnTouch: When
true
the star fill level is updated when user touches the Cosmos view. Whenfalse
the Cosmos view only shows the rating and does not act as the input control. Default:true
.
If you have multiple cosmos views that have same appearance, it can be useful to create a CosmosSettings
object and then reuse it:
var settings = CosmosSettings()
settings.starSize = 142
var cosmos1 = CosmosView(settings: settings)
var cosmos2 = CosmosView(settings: settings)
In addition, one can assign settings to cosmos view object:
var settings = CosmosSettings()
...
cosmos.settings = settings