-
Notifications
You must be signed in to change notification settings - Fork 51
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How to correctly parse a JSON #35
Comments
Hi @lbhack !
|
mucha gracias |
I'm sure you can do it yourself, following the example I gave you. |
ok thanks i get it . |
here i finished the mini project with code about Weather Information : /*
*/ /* NAppGUI Weather Info */ #include "nappgui.h" }; /* SetUp arrays and sets for Condition structure / }; struct _app_t static void init_bind()
}
} } /---------------------------------------------------------------------------/ static void i_OnClose(App *app, Event *e) } /---------------------------------------------------------------------------/ static App *i_create(void) /---------------------------------------------------------------------------/ static void i_destroy(App **app) /---------------------------------------------------------------------------/ #include "osmain.h" |
Hi @lbhack ! |
Improved Json documentation: |
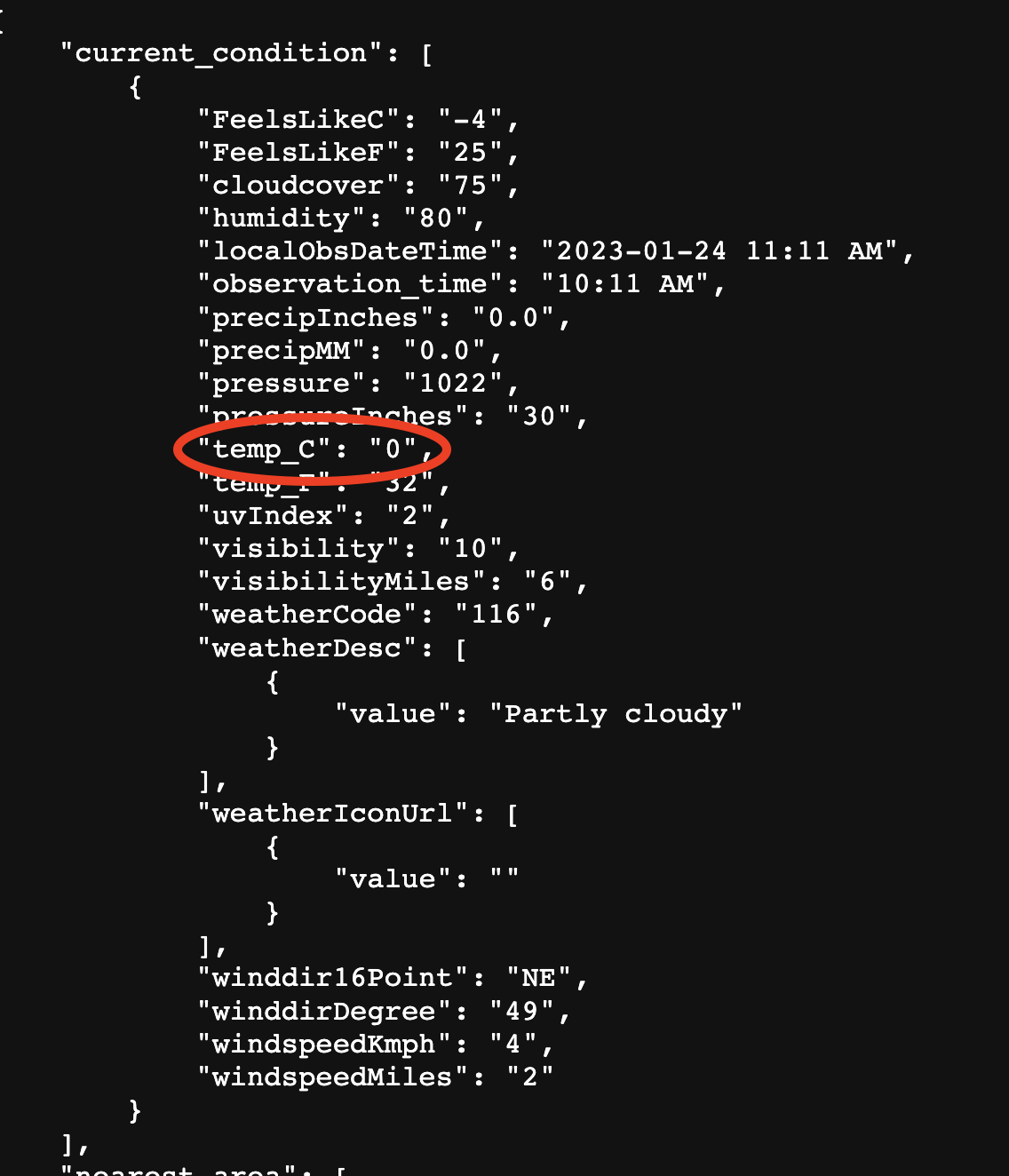
hi i want to access the field temp_C in json always getting empty value request using the code below :/*
*/
/* NAppGUI Hello World */
#include "nappgui.h"
#include "inet.h"
#include "httpreq.h"
#include "json.h"
typedef struct _app_t App;
typedef struct _currentCondition_t CurrentCondition;
typedef struct WeatherDesc {
char_t value[20];
} WeatherDesc;
typedef struct WeatherIconUrl {
char_t value[100];
} WeatherIconUrl;
struct _currentCondition_t {
uint32_t FeelsLikeC;
uint32_t FeelsLikeF;
uint32_t cloudcover;
uint32_t humidity;
char_t localObsDateTime[20];
char_t observation_time[20];
real32_t precipInches;
real32_t precipMM;
uint32_t pressure;
real32_t pressureInches;
uint32_t temp_C;
uint32_t temp_F;
uint32_t uvIndex;
uint32_t visibility;
uint32_t visibilityMiles;
uint32_t weatherCode;
WeatherDesc weatherDesc[1];
WeatherIconUrl weatherIconUrl[1];
char_t winddir16Point[5];
uint32_t winddirDegree;
uint32_t windspeedKmph;
uint32_t windspeedMiles;
};
struct _app_t
{
Window *window;
TextView *text;
};
/---------------------------------------------------------------------------/
/---------------------------------------------------------------------------/
static void i_OnButton(App *app, Event *e)
{
Stream *gdata = NULL;
CurrentCondition *json=NULL;
}
static Panel *i_panel(App *app)
{
Panel *panel = panel_create();
Layout *layout = layout_create(1, 3);
Label *label = label_create();
Button *button = button_push();
TextView *text = textview_create();
app->text = text;
label_text(label, "Hello!, I'm a label");
button_text(button, "Click Me!");
button_OnClick(button, listener(app, i_OnButton, App));
layout_label(layout, label, 0, 0);
layout_button(layout, button, 0, 1);
layout_textview(layout, text, 0, 2);
layout_hsize(layout, 0, 250);
layout_vsize(layout, 2, 200);
//layout_hsize(layout, 2, 200);
layout_margin(layout, 5);
layout_vmargin(layout, 0, 5);
layout_vmargin(layout, 1, 5);
panel_layout(panel, layout);
return panel;
}
/---------------------------------------------------------------------------/
static void i_OnClose(App *app, Event *e)
{
osapp_finish();
unref(app);
unref(e);
}
/---------------------------------------------------------------------------/
static App *i_create(void)
{
App *app = heap_new0(App);
Panel *panel = i_panel(app);
app->window = window_create(ekWINDOW_STD | ekWINDOW_RESIZE);
window_panel(app->window, panel);
window_title(app->window, "Hello, World!");
//window_size(app->window,s2df(800.0,400.0));
window_origin(app->window, v2df(500, 200));
window_OnClose(app->window, listener(app, i_OnClose, App));
window_show(app->window);
return app;
}
/---------------------------------------------------------------------------/
static void i_destroy(App **app)
{
window_destroy(&(*app)->window);
heap_delete(app, App);
}
/---------------------------------------------------------------------------/
#include "osmain.h"
osmain(i_create, i_destroy, "", App)
curl --silent wttr.in/spain?format=j1 | grep -i -o ""temp_c":.*[0-9]" --color=auto | sed 's/"//g'
The text was updated successfully, but these errors were encountered: