In app chat
Find more info about Live chat product on Infobip docs.
- Intro
- Example application
- Display In-app chat view
- Customize In-app chat view
- Handle notification taps
- In-app chat events
- Sending attachments
- Attachments preview
- Supported attachment types
- Unread chat push messages counter
- Changing localization
- Sending Contextual Data
- Multiple chat threads
- Authenticated chat
- Chat and push notifications
- Example application
- Troubleshooting
In-app chat is the mobile component for connecting and interacting with Infobip's LiveChat product. In-app chat is built on top of Mobile Messaging SDK and that's why Mobile Messaging should be included into your application and properly configured. In-app chat requires from you a careful setup, both in your mobile app and in Infobip's portal. The following steps must be prepared in order to ensure the chat communication:
-
Include and setup Mobile Messaging SDK plugin in your application. If you haven't already, please follow its Quick start guide carefully. Only by being able to receive a
pushRegistrationId
in your device, you'll be able to successfully connect to In-app chat in the next steps. -
Create a LiveChat Widget and link Mobile Messaging application profile you created in step 1 to the widget configuration.
-
Setup In-app chat for Mobile Messaging SDK plugin, put
inAppChatEnabled: true
in configuration:
InfobipMobilemessaging.init(Configuration(
...
inAppChatEnabled: true,
...
));
By default in-app chat screen will be displayed with toolbar and back button at the left corner for closing the screen.
InfobipMobilemessaging.showChat()
![]() |
InfobipMobilemessaging.showChat(shouldBePresentedModallyIOS: true);
You can define your own custom appearance for chat view: It is part of Configuration
object passed to InfobipMobilemessaging.init()
. If it is undefined, its settings will be taken from web widget configuration (as defined in Infobip Portal).
We offer an example on chat customization in the example application.
- Top bar:
-
toolbarTitle
(String) -- title for the top bar -
toolbarTitleColor
(String) -- in hex format, color of top bar title text -
toolbarTintColor
(String) -- in hex format, tint color of top bar -
toolbarBackgroundColor
(String) -- in hex format, background of top bar
-
- Chat:
-
widgetTheme
(String) -- name of the Livechat widget theme, you can read more about Livechat widget themes -
chatBackgroundColor
(String) -- in hex format, chat background color -
noConnectionAlertTextColor
(String) -- in hex format, text color at no internet connection alert -
noConnectionAlertBackgroundColor
(String) -- in hex format, background color in no internet connection alert
-
- Input:
-
chatInputSeparatorVisible
(Bool) -- controls visibility of separator line between chat and chat input -
attachmentButtonIcon
(String) -- path to file, icon which represent add attachment button in chat input -
sendButtonIcon
(String) -- path to file, icon which represent send button in chat input -
sendButtonTintColor
(String) -- in hex format, tint color of chat input send button -
chatInputPlaceholderColor
(String) -- in hex format, color of chat input placeholder -
chatInputCursorColor
(String) -- in hex format, color of chat input cursor -
chatInputBackgroundColor
(String) -- in hex format, background color of chat input
-
-
attachmentPreviewBarsColor
(String) -- in hex format, changes color of background and navigation bar when previewing an attachment -
attachmentPreviewItemsColor
(Double) -- in hex format, changes tint color of loading indicator and error alert when previewing an attachment -
textContainerTopMargin
(Double) -- top margin of text container -
textContainerLeftPadding
(Double) -- left padding of text container -
textContainerCornerRadius
(Double) -- corner radius of text container -
textViewTopMargin
(Double) -- text view top margin -
placeholderHeight
(Double) -- height of placeholder -
placeholderSideMargin
(Double) -- placeholder left and right margins -
buttonHeight
(Double) -- height of chat input buttons -
buttonTouchableOverlap
(Double) -- change touchable area of a send button -
buttonRightMargin
(Double) -- right margin of a send button -
utilityButtonWidth
(Double) -- width of an attachment button -
utilityButtonBottomMargin
(Double) -- bottom margin of an attachment button -
initialHeight
(Double) -- initial height of a chat input -
mainFont
(String) -- font name without extension, changes text font in: placeholder, chat input, and no connection alert views -
charCountFont
(String) -- font name without extension, changes text font in character counter view
- Status bar:
-
chatStatusBarColorLight
(Bool) -- whether icons in status bar should be light, dark otherwise -
chatStatusBarBackgroundColor
(String) -- in hex format, color of the status bar
-
- Top bar:
-
chatNavigationIcon
(String) -- path to file, top bar navigation icon -
chatNavigationIconTint
(String) -- in hex format, color of a navigation icon -
chatSubtitleText;
(String) -- text of the top bar subtitle -
chatSubtitleTextColor
(String) -- in hex format, color of subtitle in the top bar -
chatSubtitleTextAppearanceRes
(String) -- id of text appearance from android styles used for the top bar subtitle, e.g.,: TextAppearance_AppCompat_Subtitle -
chatSubtitleCentered
(Bool) -- whether the top bar subtitle should be centered horizontally -
chatTitleTextAppearanceRes
(String) -- id of text appearance from android styles used for the top bar title -
chatTitleCentered
(Bool) -- whether the top bar title should be centered horizontally -
chatMenuItemsIconTint
(String) -- in hex format, color of top bar menu items icon -
chatMenuItemSaveAttachmentIcon
(String) -- path to file, top bar save menu item icon in attachment preview screen
-
- Chat:
-
chatProgressBarColor
(String) -- in hex format, color of a loading indicator -
chatNetworkConnectionErrorText
(String) -- text of a no connection alert -
chatNetworkConnectionErrorTextAppearanceRes
(String) -- id of text appearance from android styles used for a no connection alert
-
- Input:
-
chatInputSeparatorLineColor
(String) -- in hex format, color of a separator between the chat and the chat input -
chatInputHintText
(String) -- text of a chat input placeholder -
chatInputTextColor
(String) -- in hex format, color of text in input field -
chatInputTextAppearance
(String) -- id of text appearance from android styles used for a chat input text -
chatInputAttachmentIconTint
(String) -- in hex format, color of the add attachment icon in the input view -
chatInputAttachmentBackgroundColor
(String) -- in hex format, background color of the attachment icon in the input view -
chatInputAttachmentBackgroundDrawable
(String) -- path to file, icon which is used as background for attachment button in chat input -
chatInputSendIconTint
(String) -- in hex format, color of the send icon in the input view -
chatInputSendBackgroundColor
(String) -- in hex format, background color of the send icon in the input view -
chatInputSendBackgroundDrawable
(String) -- path to file, icon which is used as background for send button in chat input
-
Note: Same style can be achieved in an xml format of android styles. Define your own theme.
Livechat widget themes are defined on the Live chat widget setup page on Infobip Portal. You can define multiple custom themes and customize various widget attributes, read Livechat documentation to get information about all possible widget customization options.
To set Livechat theme in In-app chat you must:
- Define the name and values of your theme(s) in a JSON format, under widget section Theme -> Advanced Customization, in Infobip Portal.
- Once you know the names of your themes, you can use them in
Configuration
.
Mobile Messaging Plugin has notificationTapped
event, which will be sent when user opens the app by tapping on the notification alert. Note that chat messages may be recognised by chat
attribute:
InfobipMobilemessaging.on(
LibraryEvent.notificationTapped,
(Message message) => {
if (message.chat) {print('Chat message tapped')}
});
In-app chat library supports all core SDK library events plus following chat specific events: All In-app chat events are currently supported only in Android.
Event | Parameters | Description |
---|---|---|
unreadMessageCounterUpdated |
Unread messages count | Triggered when number of unread messages is changed. |
chatViewStateChanged |
In-app chat view name: LOADING , THREAD_LIST , LOADING_THREAD , THREAD , CLOSED_THREAD , SINGLE_MODE_THREAD , UNKNOWN
|
Triggered when when view in InAppChat is changed. |
chatConfigurationSynced |
Triggered when chat configuration is synchronized. (Note: Android only) | |
chatLivechatRegistrationIdUpdated |
Livechat registration id | Triggered when livechat registration id is updated. (Note: Android only) |
Library events can be subscribed on using the following code snippet:
import 'package:infobip_mobilemessaging/models/library_event.dart';
InfobipMobilemessaging.on(
LibraryEvent.'<event name>',
(event) => {
print('Event received: ' + event.toString());
});
We've added sending attachments support, more info in iOS SDK docs, Android SDK docs
For saving attachments to photo library you will need to add additional permissions
An API is available to get and reset current unread chat push messages counter. The counter increments each time the application receives chat push message (this usually happens when chat screen is inactive or the application is in background/terminated state). In order to get current counter value use following API:
int counter = await InfobipMobilemessaging.getMessageCounter();
MobileMessaging SDK automatically resets the counter to 0 whenever user opens the chat screen. However, use the following API in case you need to manually reset the counter:
InfobipMobilemessaging.resetMessageCounter();
You can register to the event unreadMessageCounterUpdated
with inAppChat.unreadMessageCounterUpdated
value, in order to get updates of the counter in runtime.
The predefined messages prompted within the In-app chat (such as status updates, button titles, input field prompt) by default are localized using system locale setting, but can be easily changed providing your locale string with the following formats: "es_ES", "es-ES", "es".
InfobipMobilemessaging.setLanguage("es");
It is possible to send contextual data / metadata to Infobip’s Conversations via mobile messaging SDK's chat. Data can be sent anytime, several times, with only one restriction: the chat must be already loaded and presented, and the communication should have started (meaning, there are messages visible and not the initial “Start the chat” button). Sent data will be automatically linked to the conversationId and accountId internally.
There are two parameters:
- The mandatory data, sent as string, in the format of Javascript objects and values (for guidance, it must be accepted by JSON.stringify())
- And optionally, an "all multithread strategy" that can be left empty, and will use
false
(ACTIVE) as default. Possible values are: metadata sent to "ACTIVE" conversation for the widget (withfalse
), or to "ALL" non closed conversations for the widget (withtrue
).
Usage:
InfobipMobilemessaging.sendContextualData("{ exampleKey: 'InAppChat Metadata Value Example' }", false);
Default LiveChat widget works with single chat thread, one customer can only have one single open conversation. But the LiveChat widget settings page offers option to enable multiple chat threads.

When the setting above is enabled, the In-app chat UI will automatically offer in mobile:
- A list (initially empty) of all the unsolved conversation threads the user has opened.
- A button to "Start new chat" thread.
- A navigation to each specific conversation thread, to open particular chat view tap on conversation thread in the list.
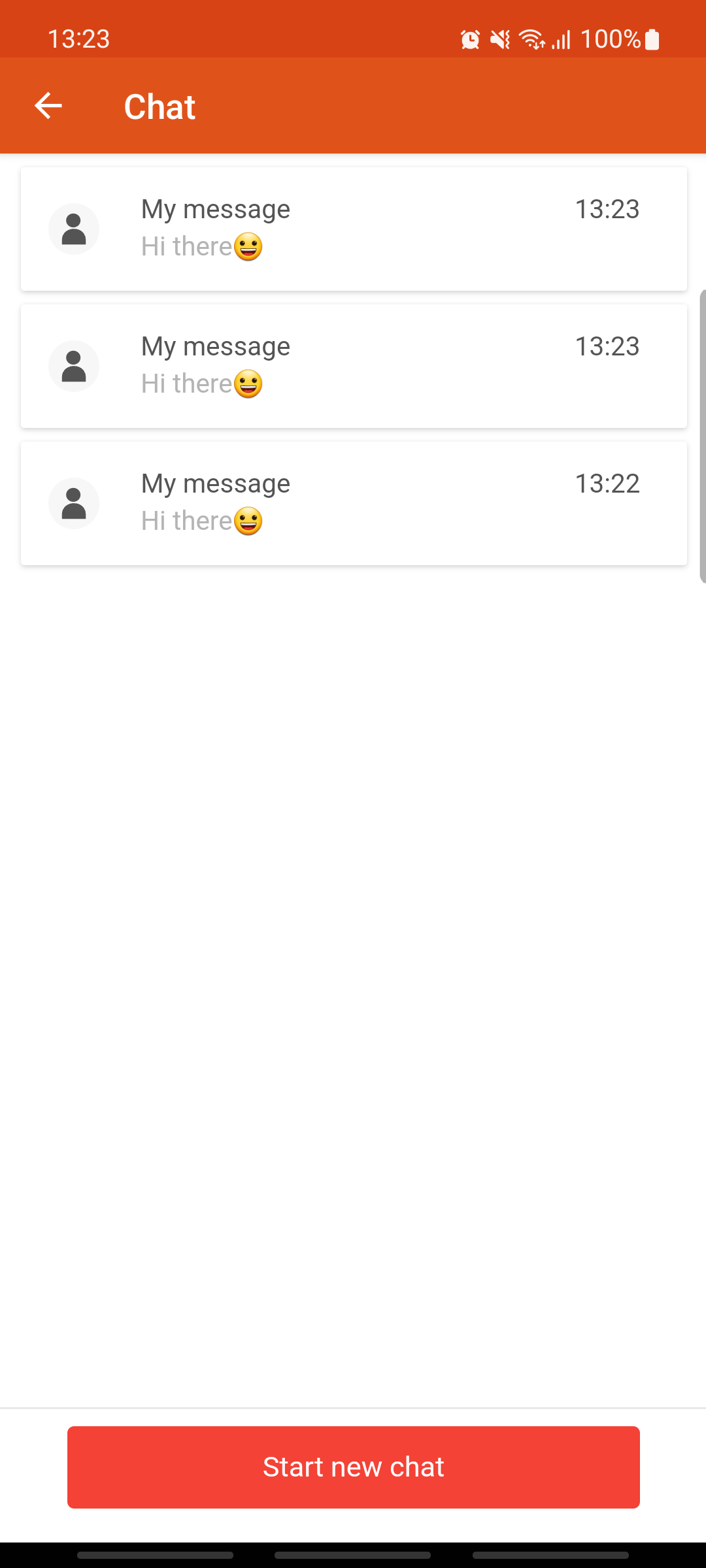
The functionality for multiple chat threads works out of the box: there is no need for extra implementation in the mobile integrator's side.
It is possible to authenticate a user before accessing the In-app chat, given you have enabled this feature in the LiveChat widget.

The authentication is accomplished by combining the Mobile Messaging SDK personalisation method with a JWT (JSON Web Token). The token needs to be generated by your end (see instructions here).
The authentication will use a unique identifier for your user, that can be an email address, a phone number, or an external identifier. It is crucial for this identifier to be valid, and to match the identity defined in the UserIdentity
you passed in Mobile Messaging SDK personalisation call - there will be an authentication error otherwise.
Mobile messaging provides setJwt()
method to give In-app chat the ability to authenticate users. In Android, provided JWT can be used multiple times during In-app chat lifetime, due to various
events like screen orientation change, internet re-connection and others. You must ensure JWT expiration time is more than In-app chat lifetime.
/*
1 - The customer authenticate in your system, and you recognise his/her unique identifier
2 - You call personalise (InfobipMobilemessaging.personalize()) with this unique identifier (and optionally, with other attributes such as first name, second name, etc)
3 - Now you can display the chat as an authenticated user by doing the following:
*/
InfobipMobilemessaging.setJwt("your JWT");
InfobipMobilemessaging.showChat();
In-app chat decides automatically when to send push notifications for incoming messages events based on the following rules:
- If In-app chat is not loaded/disconnected => push notifications will be sent.
- If your app is in the background or a device is locked regardless of its connection status => push notifications will be sent.
- If the app is in the foreground and In-app chat is loaded/connected => push notification will NOT be sent.
In Android In-app chat connection is established and stopped based on component lifecycle. Chat connection is active only when Lifecycle.State
is at least Lifecycle.State.STARTED
. Chat connection is stopped when Lifecycle.State
is below Lifecycle.State.STARTED
.
In iOS chat could be loaded/connected but your app may have it hidden (e.g. behind another view) we provide you with the flexibility to control its connection. You can still receive push notifications in the foreground with these methods: stopConnection
and restartConnection
. Usage example:
InfobipMobilemessaging.showChat();
// Hide the chat, e.g., by displaying a new modal on top of it
InfobipMobilemessaging.stopConnection();
// Push notifications for new message events will start coming to the device
// When you detect that a chat is visible again to a user, you can reload the chat and stop push notifications by invoking:
InfobipMobilemessaging.restartConnection();
If you face any issue using the chat, specially on the first time integration, we encourage to try your application code/certificates in our Example application, as this may give you a hint of potential mistakes. An example of the most common issues our integrators face:
- I get in the logs an error about no push registration id.
Please re-check the quick start guide and the steps mentioned above. For iOS, specially the part about the difference between Sandbox and Production environments, and how they need different p12 files uploaded to Infobip's web portal for Apple push notification's to work.
- When a chat push notification is tapped, the app is invoked, and remains wherever it previously was - but I want it to display the chat related to the push notification I tapped.
InAppChat cannot present itself: if needs the parent application (your app) to present it. So, when a push notification is tapped, your app needs to recognise that event, and present the chat if you wish so. You can detect when a push notification is tapped, its nature and content, by listening to our library-events. For this case, the event you are looking for is called 'notificationTapped'.
- The chat content appears blank, and the text input fields is disabled.
There are many reasons for the chat not working: from incorrect codes/ids in your setup, to badly defined livechat widget in Infobip's web portal. Usually, the console logs (if you enabled debug logs in our SDK) will give you a hint of the issue, and re-checking this guide, or comparing with our Example, should be enough to successfully integrate InAppChat. But if the issue continues, don't exitate to contact our support or ask for help here in our repository's Issues section.
- I have all the setup correct, but the chat is still blank and disabled.
Please confirm, within the security and authentication (last) section of your widget setup (in Infobip's web portal), if "Mobile app customer authentication - Authenticate users on mobile using JSON Web Token" is enabled. If you enabled it by mistake, disable it. If you want to authenticate mobile users, make sure you are sending a correct JSON Web Token (otherwise, similar to the point #1, the chat will remain disabled).
- Library events
- Server errors
- User profile
- Messages and notifications management
- Inbox
- Privacy settings
- In-app chat
- WebRTC Calls and UI
- Migration Guides