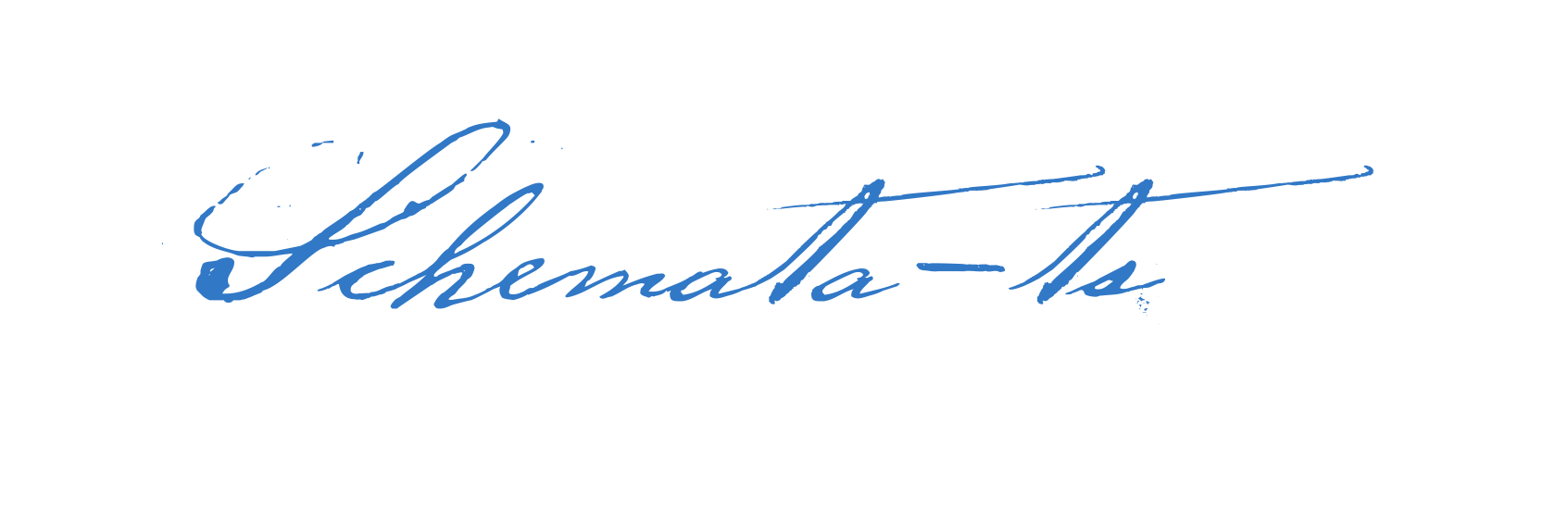
An all-inclusive schema engine featuring schemata inspired by io-ts and validators.js. Written for TypeScript with fp-ts
Schemata-ts
is an unofficial continuation of io-ts
v2 built from the ground up using the highly extensible Schemable
/Schema
API. Schemata also comes with a suite of string validation schemas and branded types β powered by Kuvio β all inspired by io-ts-types and validators.js.
Features | |
---|---|
β | Validation, Parsing, and Serialization |
β | Type Guards |
β | Json-Schema Draft 07, 2019-09, and 2020-12 |
β | Fast-Check Arbitraries |
β | and more |
yarn add schemata-ts
npm install schemata-ts
pnpm add schemata-ts
A note on fast-check: Schemata lists fast-check
as a peer dependency. As a result, it doesn't need to be installed for schemata to work. It is recommended to install fast-check
as a dev dependency which will satisfy the peer dependency requirement. To avoid fast-check being bundled in a front-end application, only import from the Arbitrary
module in test files.
A Schema
is a simple function that's architected using service-oriented principles. It's a function that takes a Schemable
which is an interface of capabilities that produce a particular data-type. Schemas aren't intended to be constructed by users of the library but instead are pre-constructed and exported from the root directory.
The following import will give you access to all of the pre-constructed schemas.
import * as S from 'schemata-ts'
In addition to "primitive" schemas like S.String(params?)
, S.Int(params?)
, S.Boolean
, schemata-ts also exports schema combinators from the root directory. 'Combinator' is a fancy word for a function that takes one or more schemas and returns a new schema. For example, S.Array(S.String())
is a schema over an array of strings, and S.Struct({ foo: S.String() })
is a schema over an object with a single property foo
which is a string.
import * as S from 'schemata-ts'
export const PersonSchema = S.Struct({
name: S.String(),
age: S.Int({ min: 0, max: 120 }),
isCool: S.Boolean,
favoriteColors: S.Array(S.String()),
})
There are three ways to transform a schema in schemata-ts
: 'combinators' which are functions that take schemas as parameters and return new schemas, 'transformers' which are specific to particular schemata, and Imap
which applies an invariant transformation to the underlying data-type.
Aside from Guard
, all data-types derivable from schemas are invariant functors. This means that supplying mapping functions to
and from
to Imap
adjusts the Output
of that particular data type. Because Guard
is not invariant, you must supply the resulting Guard
to the invariant map. The combinator version of this is Imap
. In version 3.0
(coming late 2023/early 2024) it will be possible to .imap()
any schema.
Schema transformers are classes which extend SchemaImplementation
, and allow adjustment to the underlying schema parameters after it has been declared in an immutable fashion. This is useful for type-specific methods like pick
or omit
for Struct
.
Here are the current transformers and available methods,
- Struct:
pick
,omit
,partial
,partialOption
,readonly
,strict
,addIndexSignature
,extend
,intersect
- Array:
minLength
,maxLength
,nonEmpty
- String:
brand
,minLength
,maxLength
,errorName
- Int:
brand
,min
,max
,errorName
- Float:
brand
,min
,max
,errorName
- Tuple:
append
,prepend
... with more to come!
const SoftwareDeveloperSchema = PersonSchema.omit('isCool')
.extend({
favoriteLanguages: S.Array(S.String()),
favoriteFrameworks: S.Array(S.String()),
})
.strict()
Schemas can be used to extract the underlying TypeScript type to avoid writing the same definition twice and different parts of code getting out of sync.
Schemas have reference to both the input and output type. The input type is more often for usage outside of JavaScript land (such as over the wire in an API request), and the output type is more often for usage within JavaScript land.
export type Person = S.OutputOf<typeof PersonSchema>
export type PersonInput = S.InputOf<typeof PersonSchema>
Schemata-ts
's type-class for validation and parsing is called "Transcoder." Transcoders can be derived from schemas using deriveTranscoder
:
import { deriveTranscoder, type Transcoder } from 'schemata-ts/Transcoder'
const personTranscoder: Transcoder<PersonInput, Person> = deriveTranscoder(PersonSchema)
Transcoders are intended to succeed Decoder
, Encoder
, and Codec
from io-ts
v2. They contain two methods: decode
and encode
. The decode
method takes an unknown value to an fp-ts Either
type where the failure type is a schemata-ts
error tree called TranscodeError
, and the success type is the output type of the schema.
In addition to parsing an unknown value, Transcoder can transform input types. One example is MapFromEntries
which takes an array of key-value pairs and transforms it into a JavaScript Map
type.
import * as Str from 'fp-ts/string'
const PeopleSchema = S.MapFromEntries(Str.Ord, S.String(), PersonSchema)
const peopleTranscoder: Transcoder<
ReadonlyArray<readonly [string, PersonInput]>,
ReadonlyMap<string, Person>
> = deriveTranscoder(PeopleSchema)
Schemas can be turned into printer-parsers using various Parser
schemas, such as:
(De)Serialization from Json String:
const parsePersonTranscoder: Transcoder<S.JsonString, Person> = deriveTranscoder(
S.ParseJsonString(PersonSchema),
)
or, (De)Serialization from a Base-64 encoded Json String:
const parsePersonTranscoder: Transcoder<S.Base64, Person> = deriveTranscoder(
S.ParseBase64Json(PersonSchema),
)
Transcoders can be parallelized using TranscoderPar
which is a typeclass similar to Transcoder but returns TaskEither
s instead of Either
s. This allows for parallelized validation for schemas of multiple values like structs and arrays.
import { deriveTranscoderPar, type TranscoderPar } from 'schemata-ts/TranscoderPar'
const personTranscoderPar: TranscoderPar<PersonInput, Person> =
deriveTranscoderPar(PersonSchema)
Type guards are used by TypeScript to narrow the type of a value to something concrete. Guards can be derived from schemas using deriveGuard
:
import { deriveGuard, type Guard } from 'schemata-ts/Guard'
const guardPerson: Guard<Person> = deriveGuard(PersonSchema)
Json-Schema is a standard for describing JSON data. Schemata-ts can derive Json-Schema from schemas using deriveJsonSchema
for versions Draft-07, 2019-09, and 2020-12.
import { deriveJsonSchema } from 'schemata-ts/JsonSchema'
const personJsonSchemaDraft07 = deriveJsonSchema(PersonSchema, 'Draft-07')
const personJsonSchema2019 = deriveJsonSchema(PersonSchema)
const personJsonSchema2020 = deriveJsonSchema(PersonSchema, '2020-12')
Fast-Check is a property-based testing library for JavaScript. Schemata-ts can derive fast-check arbitraries from schemas using deriveArbitrary
:
import * as fc from 'fast-check'
import { deriveArbitrary } from 'schemata-ts/Arbitrary'
const personArbitrary = deriveArbitrary(PersonSchema).arbitrary(fc)
Schemata has other derivations besides the ones above, below are links to those places in the documentation.
- MergeSemigroup: A customizable schema specific deep-merge (source)
- Eq: A schema-specific equality check (source)
- TypeString: Input / Output type strings (source)
Thanks goes to these wonderful people (emoji key):
Jacob Alford π» |
Dan Minshew π» |
Jonathan Skeate π» |
Peter Krol π» |
golergka π |
This project follows the all-contributors specification. Contributions of any kind welcome!