You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
In my web app the timetable controller looses the ability to 'animateTo' whenever there is a change in the eventlist. The timetable keeps working correctly otherwise (I can swipe through the weeks manually, updated events show correctly, onTap keeps working, etc.). I can even use the timetableController to get information about the currentstate (e.g. currently displayed week). The only thing that seems to stop working is 'AnimateTo'. If I reload the widget through "popAndPushNamed", it works again, until there is a change in a event. Not a 100% sure this is a bug or an issue with my code, but since everything else seems to work correctly I'm posting it here..
I'm not sure that this is causing the issue, but the TimetableController is supposed to be instantiated only once (usually in initState) and then get reused across invocations of build. You can then use EventProvider.simpleStream(Stream<List<E>> eventStream) with a StreamController to change the displayed events after initState has been executed. Though I'm a bit confused that you can still read information from the controller, so the cause may be something else…
In my web app the timetable controller looses the ability to 'animateTo' whenever there is a change in the eventlist. The timetable keeps working correctly otherwise (I can swipe through the weeks manually, updated events show correctly, onTap keeps working, etc.). I can even use the timetableController to get information about the currentstate (e.g. currently displayed week). The only thing that seems to stop working is 'AnimateTo'. If I reload the widget through "popAndPushNamed", it works again, until there is a change in a event. Not a 100% sure this is a bug or an issue with my code, but since everything else seems to work correctly I'm posting it here..
Screenshot:
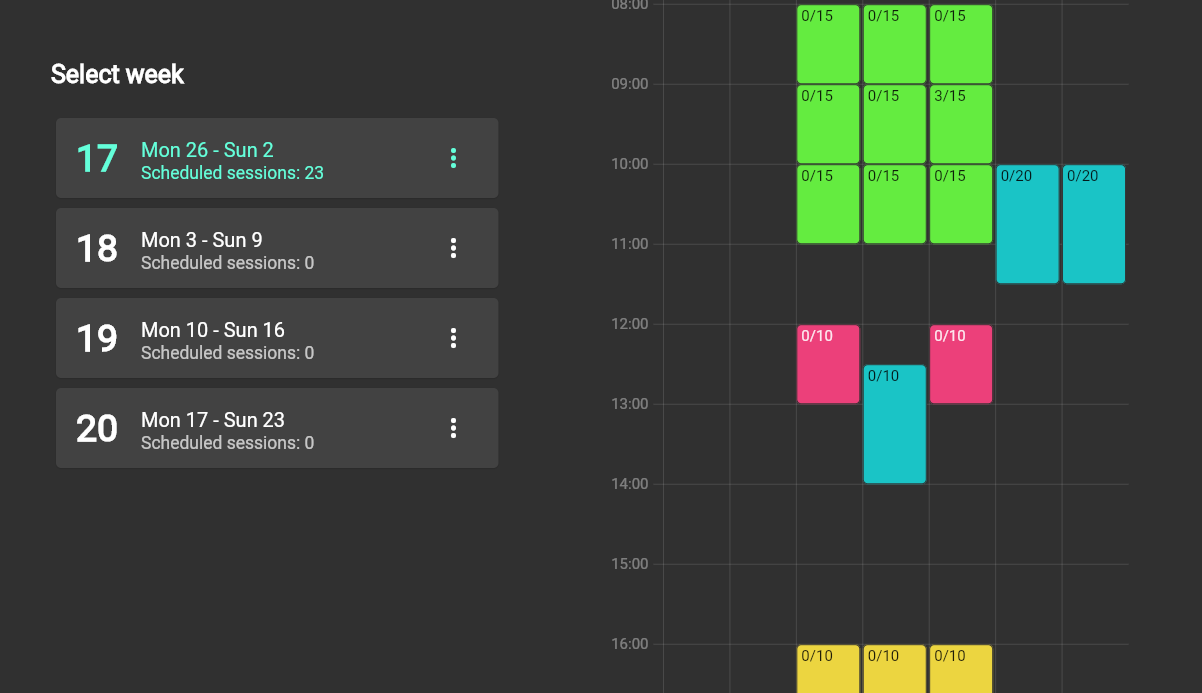
Environment:
Code that displays the calendar:
Child widget with the list of weeks:
The text was updated successfully, but these errors were encountered: