-
-
Notifications
You must be signed in to change notification settings - Fork 1.6k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Problem when switching system language on android #1704
Comments
Hi @uberchilly, I have an example of language switching at the link below, see if it can help you https://gist.github.com/eduardoflorence/9503ca35950559618da71f55a74b6562 |
@eduardoflorence Thank you. I am not trying to switch language by user action, I am trying to make my app follow the system's language like it is the default behavior on Android. Will the code sample above do that? |
Hello, i am irritated too, how do i watch the device locale with getX? When deviceLocale ( I would like to get the minimum sample code for it please |
@uberchilly Do you have a solution so far? |
@jonataslaw can you help please? How to simply watch |
I did it using You can go this way (... |
Maybe related #2019 |
This works for me in getx 4.5.1. I am closing the issue. |
Describe the bug
Switching system language in settings and going back to the app on android doesn't change the app's language
**Reproduction code
example:
To Reproduce
Steps to reproduce the behavior:
Expected behavior
App changes language to fr_FR
Screenshots
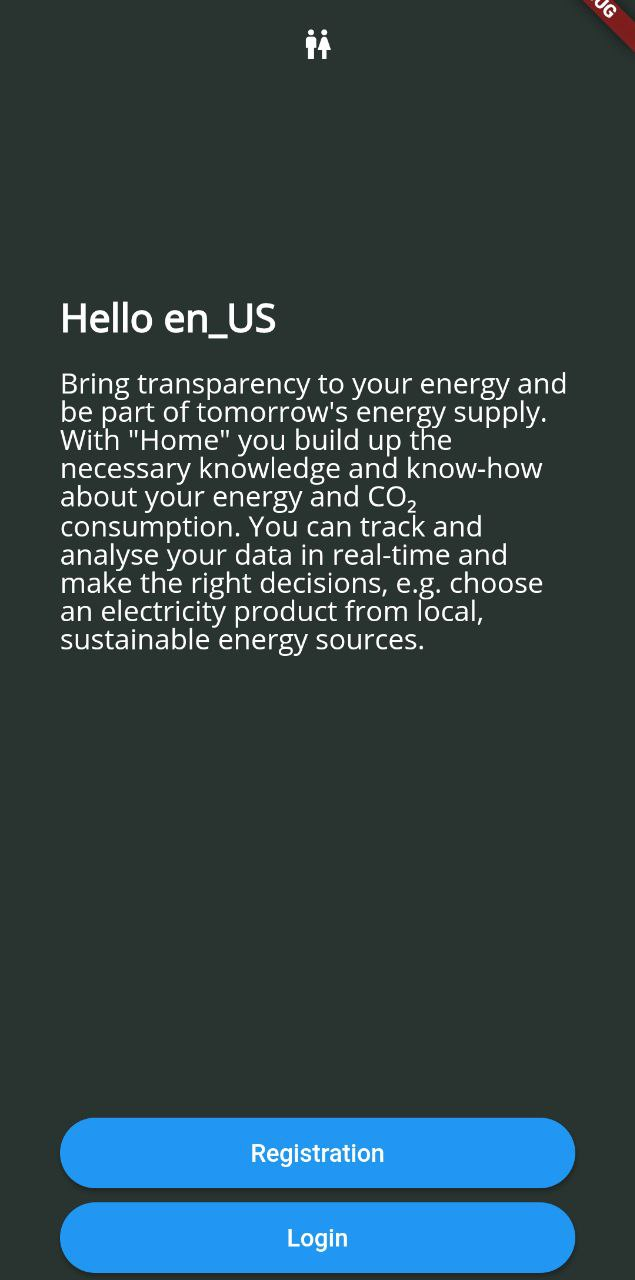
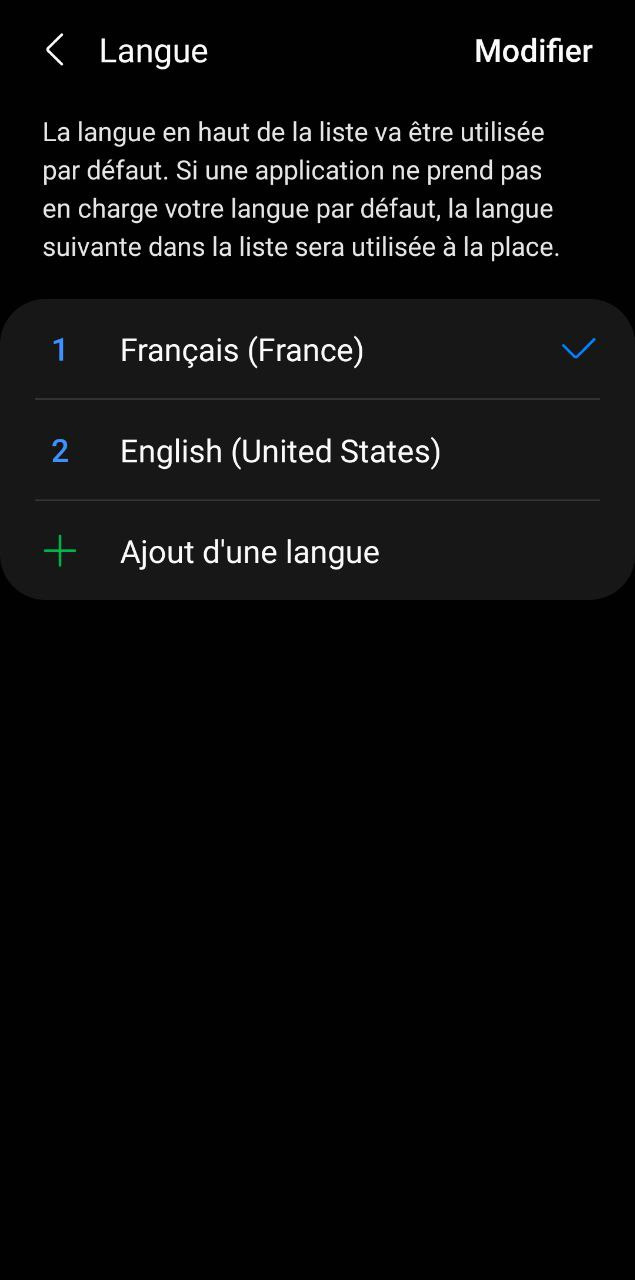
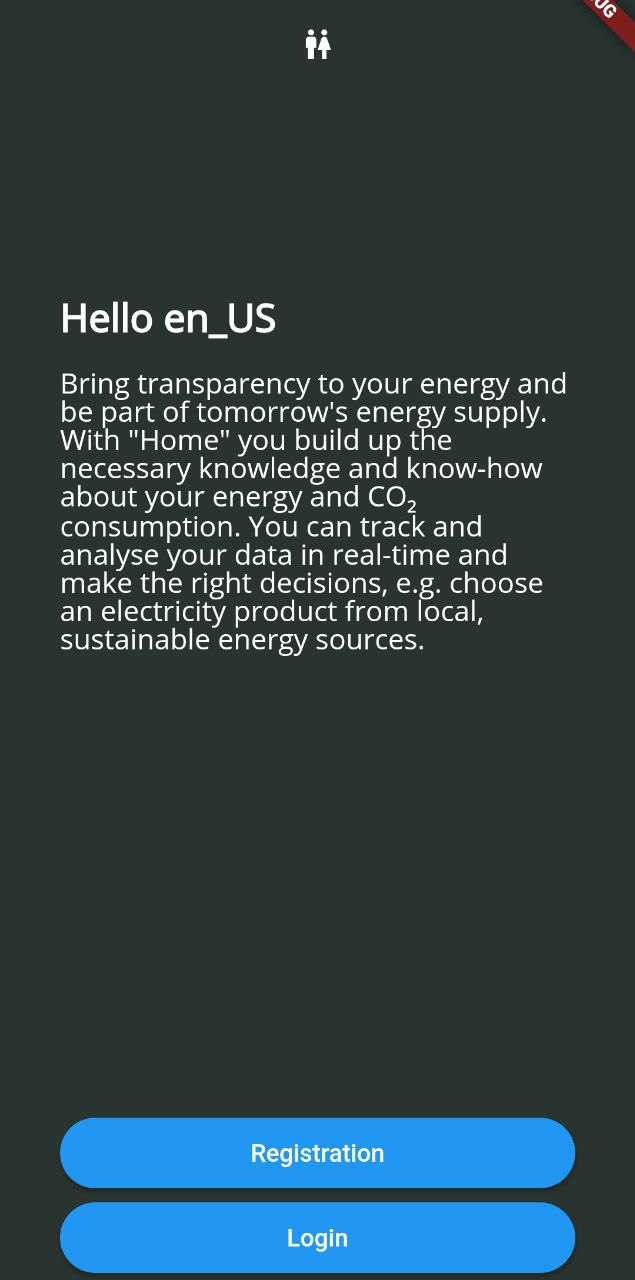
Flutter Version:
Flutter (Channel stable, 2.2.3, on Microsoft Windows [Version 10.0.19042.1110]
Getx Version:
^4.3.0
Describe on which device you found the bug:
Samsung galaxy s21 and every other device and emulator that I have tried
The text was updated successfully, but these errors were encountered: