We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
链栈的即有一个头结点,然后依次在头结点后面插入节点,形成链栈
基本操作有:
#include <stdio.h> #include <stdlib.h> #include "linkStack.h" int main() { LinkStack S; LinkStack s; char ch[50],e,*p; initStack(&S); printf("请输入进栈的字符;\n"); gets(ch); p = &ch[0]; while(*p) { pushStack(S,*p); p++; } printf("显示当前栈顶元素:\n"); getTop(S,&e); printf("%4c\n",e); printf("输出栈中元素的个数: %d \n",stackLength(S)); printf("元素出栈的序列:\n"); while(!stackEmpty(S)) { popStack(S,&e); printf("%4c\n",e); } printf("\n"); return 0; }
#ifndef LINKSTACK_H_INCLUDED #define LINKSTACK_H_INCLUDED struct node { char data; struct node *next; }; typedef struct node LStackNode,*LinkStack; // 初始化链栈 void initStack(LinkStack *top) { if((*top = (LinkStack)malloc(sizeof(LStackNode)))==NULL) exit(-1); (*top)->next = NULL; } // 判断链栈是否为空 int stackEmpty(LinkStack top) { if(top->next==NULL) return 1; return 0; } // 进栈 int pushStack(LinkStack top,char e) { LinkStack p; if((p=(LinkStack)malloc(sizeof(LStackNode)))==NULL) // 节点指针 { printf("内存分配失败"); exit(-1); } p->data = e; p->next = top->next; top->next = p; return 1; } // 退栈 int popStack(LinkStack top,char *e) { LinkStack p; p=top->next; if(!p) { printf("栈为空"); return 0; } top->next=p->next; *e = p->data; free(p); return 1; } // 取栈顶元素 int getTop(LinkStack top,char *e) { LinkStack p; p = top->next; if(!p) { printf("栈为空"); return 0; } *e = p->data; return 1; } // 获取栈中元素长度 int stackLength(LinkStack top) { LinkStack p; int count=0; p = top->next; while(p!=NULL) { count+=1; p = p->next; } return count; } // 销毁链栈 void destortStack(LinkStack top) { LinkStack p,q; p = top; while(p) { q=p; p = p->next; free(q); } } #endif // LINKSTACK_H_INCLUDED
The text was updated successfully, but these errors were encountered:
No branches or pull requests
链栈的即有一个头结点,然后依次在头结点后面插入节点,形成链栈
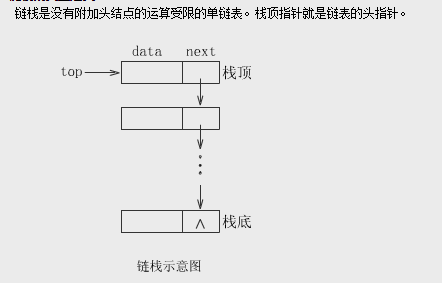
基本操作有:
主文件
头文件
结果
The text was updated successfully, but these errors were encountered: