-
-
Notifications
You must be signed in to change notification settings - Fork 34
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Is this the correct way to implement a hopfield network? #220
Comments
You're absolutely right about it being more complex than just a dense feed-forward network. To my understanding in a hopfield network all nodes connect to all other nodes so implementation-wise this depends on whether the |
but there should be backward pointing connections right ? Like this?
|
Yes exactly, we could create a new connection method that does this. Also, |
Ok, consider renaming it to And we need no additional connection type we can just do:
|
That's true and much more elegant 👍 renaming sounds good also |
We should also have some basic structural tests for architecture to avoid things like this slipping through |
carrot/src/architecture/architect.js
Lines 466 to 498 in bf9ae22
If this is correct than a hopfield network is just a simple perceptron but with STEP activation function.
I found this pics of hopfield networks:
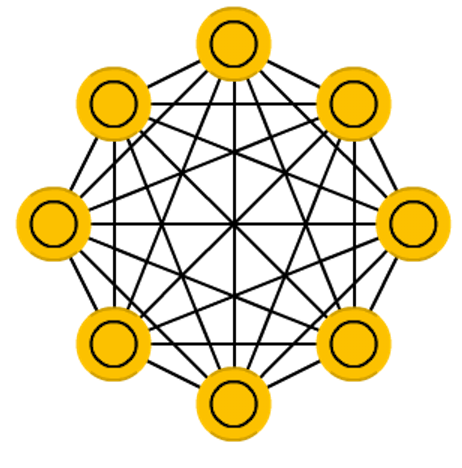
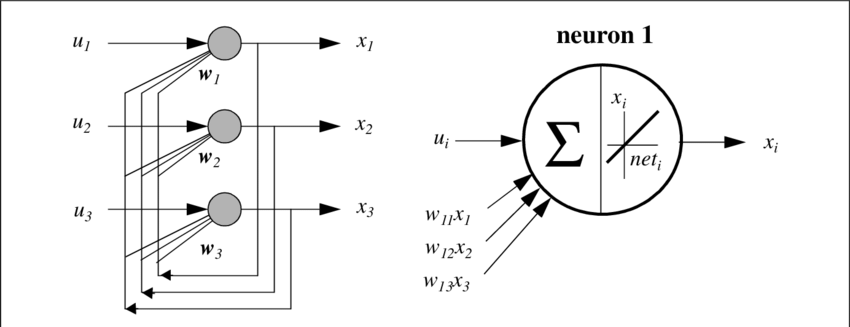
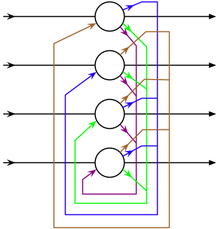
They seem like there is more ongoing then just a dense layer with step activation, or am I wrong?
Also Wikipedia says: "A Hopfield network is a form of recurrent artificial neural network" (Wikipedia). But there are only feedforward components.
The text was updated successfully, but these errors were encountered: