You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Previews spam "Unsupported format" Exceptions if the uploaded file is not a PDF file.
I already fixed it and replaced the ConvertPdf in the web.xml with my custom implementation.
My converter also considers existing "[VERSION]-conversion.pdf" files.
I placed some conversion.pdf files in the repository and everything works fine:
So here is my code, you are free to integrate it into logicaldoc, but without warranty:
import static com.logicaldoc.web.util.ServletUtil.downloadDocument;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.logicaldoc.core.document.Document;
import com.logicaldoc.core.document.Version;
import com.logicaldoc.core.document.dao.DocumentDAO;
import com.logicaldoc.core.document.dao.VersionDAO;
import com.logicaldoc.core.store.Storer;
import com.logicaldoc.util.Context;
import com.logicaldoc.web.util.ServletUtil;
/**
* This servlet simply downloads the document PDF.
*/
public class ConvertPdf extends HttpServlet {
private static final String VERSION = "version";
private static final String DOCUMENT_ID = "docId";
private static final long serialVersionUID = 1L;
protected static Logger log = LoggerFactory.getLogger(ConvertPdf.class);
@Override
public void doPost(final HttpServletRequest request, final HttpServletResponse response) {
try {
doGet(request, response);
} catch (Throwable th) {
throw new RuntimeException(th);
}
}
@Override
public void doGet(final HttpServletRequest request, final HttpServletResponse response) {
try {
var session = ServletUtil.validateSession(request);
var docDao = (DocumentDAO) Context.get().getBean(DocumentDAO.class);
var versionDao = (VersionDAO) Context.get().getBean(VersionDAO.class);
var docId = Long.parseLong(request.getParameter(DOCUMENT_ID));
var document = findDocument(docDao, docId);
var version = getDocumentVersion(request, versionDao, docId, document);
var suffix = getConversionSuffix(document);
if (documentResourceExists(docId, version, suffix)) {
downloadDocument(request, response, null, document.getId(), version.getFileVersion(), null, suffix, session.getUser());
} else {
downloadNotAvailablePdf(request, response);
}
} catch (Throwable th) {
log.error(th.getMessage(), th);
downloadNotAvailablePdf(request, response);
}
}
private Document findDocument(DocumentDAO docDao, long docId) {
var document = docDao.findById(docId);
if (document.getDocRef() != null) {
document = docDao.findById(document.getDocRef());
}
return document;
}
private Version getDocumentVersion(final HttpServletRequest request, VersionDAO versionDao, long docId, Document document) {
var ver = document.getVersion();
if (StringUtils.isNotEmpty(request.getParameter(VERSION))) {
ver = request.getParameter(VERSION);
}
return versionDao.findByVersion(docId, ver);
}
private String getConversionSuffix(Document document) {
if (isPdfDocument(document)) {
return null;
} else {
return "conversion.pdf";
}
}
private boolean documentResourceExists(long docId, Version version, String suffix) {
var storer = (Storer) Context.get().getBean(Storer.class);
var resource = storer.getResourceName(docId, version.getFileVersion(), suffix);
return storer.exists(docId, resource);
}
private void downloadNotAvailablePdf(final HttpServletRequest request, final HttpServletResponse response) {
int letter = 0;
try (InputStream is = ConvertPdf.class.getResourceAsStream("/pdf/notavailable.pdf");
OutputStream os = response.getOutputStream();) {
ServletUtil.setContentDisposition(request, response, "notavailable.pdf");
while ((letter = is.read()) != -1) {
os.write(letter);
}
} catch (Throwable e) {
log.warn(e.getMessage());
}
}
private boolean isPdfDocument(Document document) {
return StringUtils.endsWithIgnoreCase(document.getFileName(), ".pdf");
}
}
The text was updated successfully, but these errors were encountered:
Previews spam "Unsupported format" Exceptions if the uploaded file is not a PDF file.
I already fixed it and replaced the ConvertPdf in the web.xml with my custom implementation.
My converter also considers existing "[VERSION]-conversion.pdf" files.
I placed some conversion.pdf files in the repository and everything works fine:
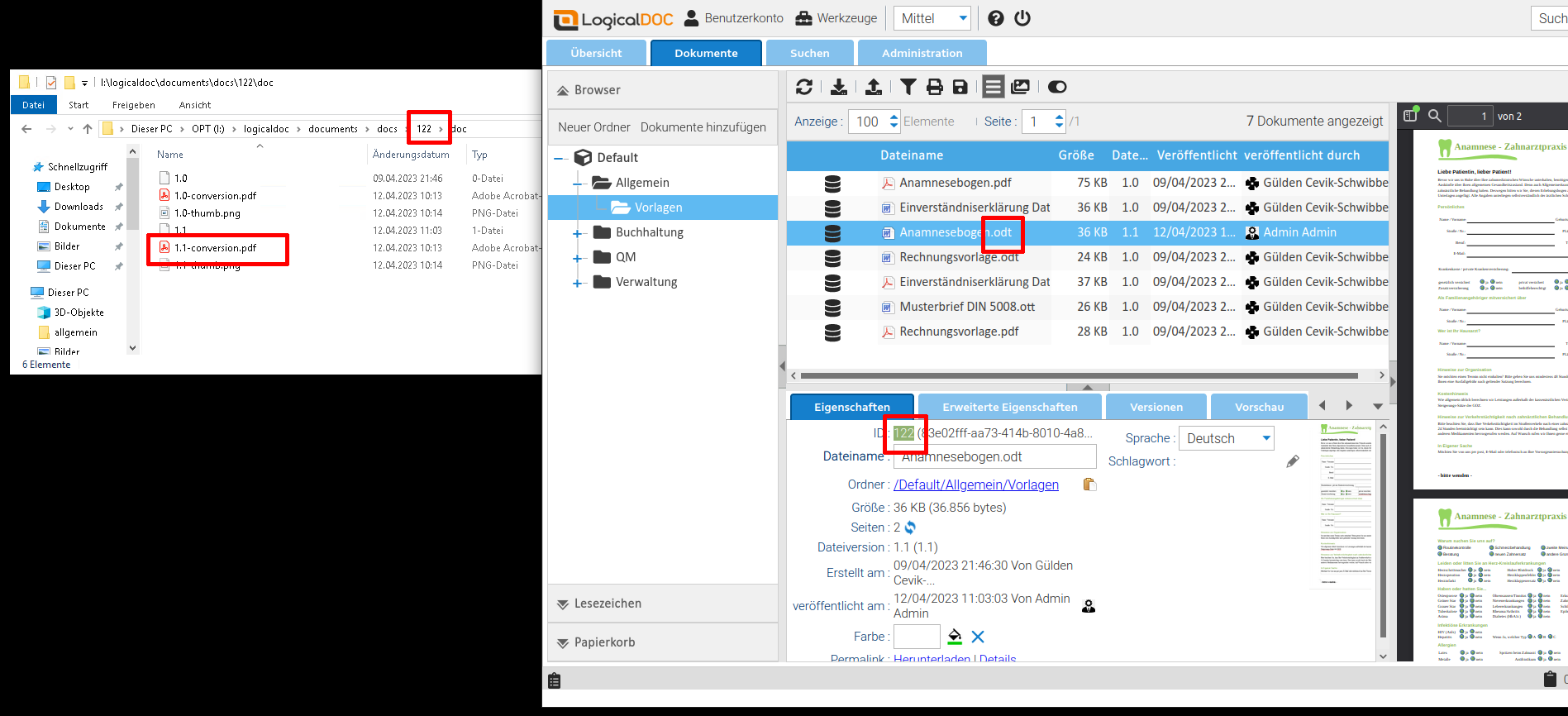
So here is my code, you are free to integrate it into logicaldoc, but without warranty:
The text was updated successfully, but these errors were encountered: