You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Hello, I notice that you didn't complete the remove_object in the last of the hw4. I complete it, maybe not perfect.
Below is my code:
def remove_object(image, mask):
"""Remove the object present in the mask.
Returns an output image with same shape as the input image, but without the object in the mask.
Args:
image: numpy array of shape (H, W, 3)
mask: numpy boolean array of shape (H, W)
Returns:
out: numpy array of shape (H, W, 3)
"""
out = np.copy(image)
### YOUR CODE HERE
from skimage import measure
label_image = measure.label(mask)
regions = measure.regionprops(label_image)
region = regions[0]
if len(regions) != 1:
print("Maybe two objects to remove?")
# Find the biggest area of region
for i in regions:
if i.area > region.area:
region = i
transposeImage = False
if region.bbox[2] - region.bbox[0] < region.bbox[3] - region.bbox[1]:
out = np.transpose(out, (1, 0, 2))
mask = np.transpose(mask, (1, 0))
transposeImage = True
count = 0 # count time for all iteration
while not np.all(mask == 0):
energy_image = energy_function(out)
energy_image = energy_image + energy_image * mask * (-1000)
vcost, vpaths = compute_forward_cost(out, energy_image)
end = np.argmin(vcost[-1])
seam = backtrack_seam(vpaths, end)
out = remove_seam(out, seam)
mask = remove_seam(mask, seam)
count += 1
#print("count = ", count)
out = enlarge(out, out.shape[1] + count)
if transposeImage:
out = np.transpose(out, (1, 0, 2))
### END YOUR CODE
return out
The result is:
I notice that the result is not perfect, below is the picture provided by. http://cs.brown.edu/courses/cs129/results/proj3/taox/
In the top right have some subtle change. I don't know how to do better. If you have some ideas
to improve it, we could achieve best result.
The text was updated successfully, but these errors were encountered:
The result is:
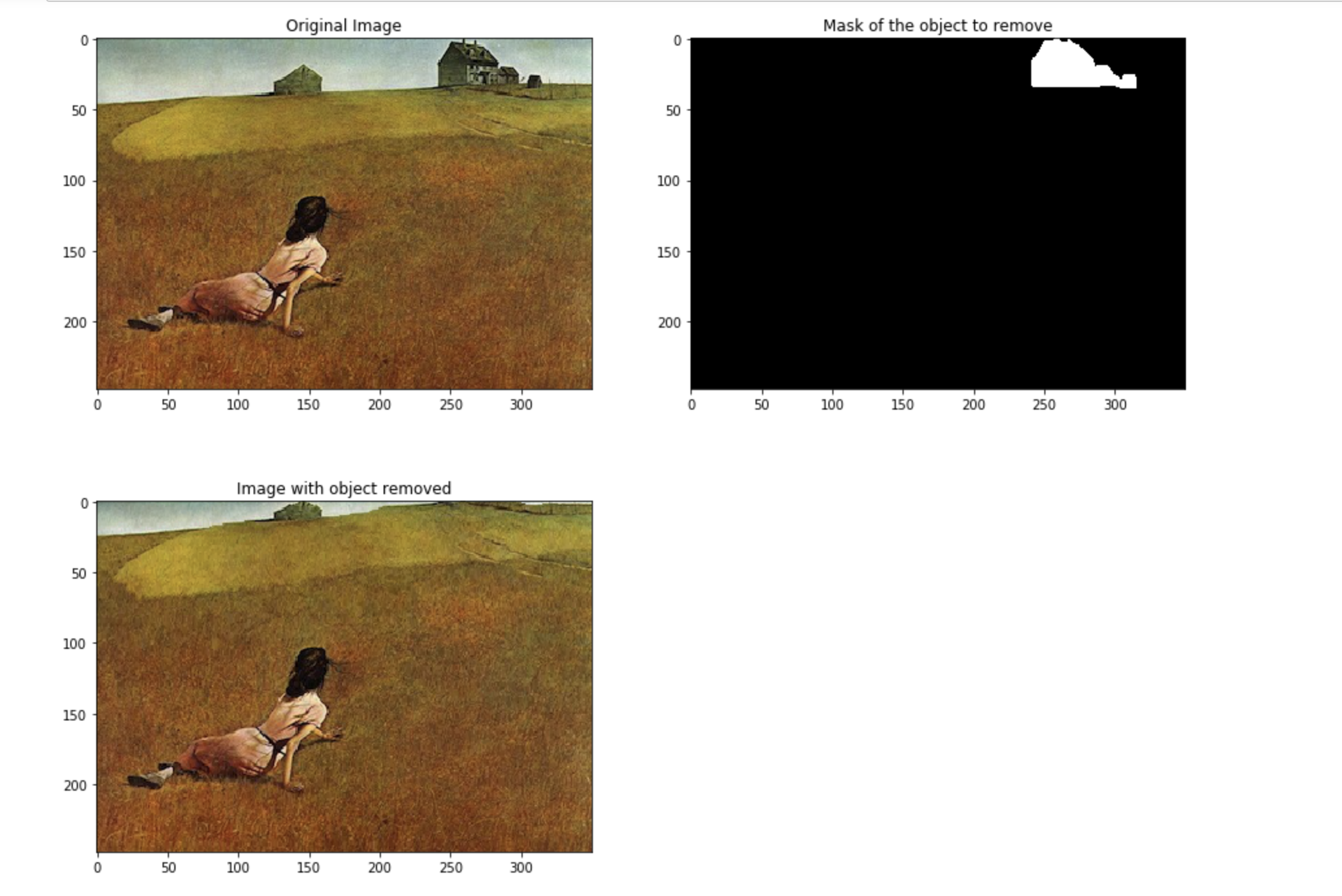
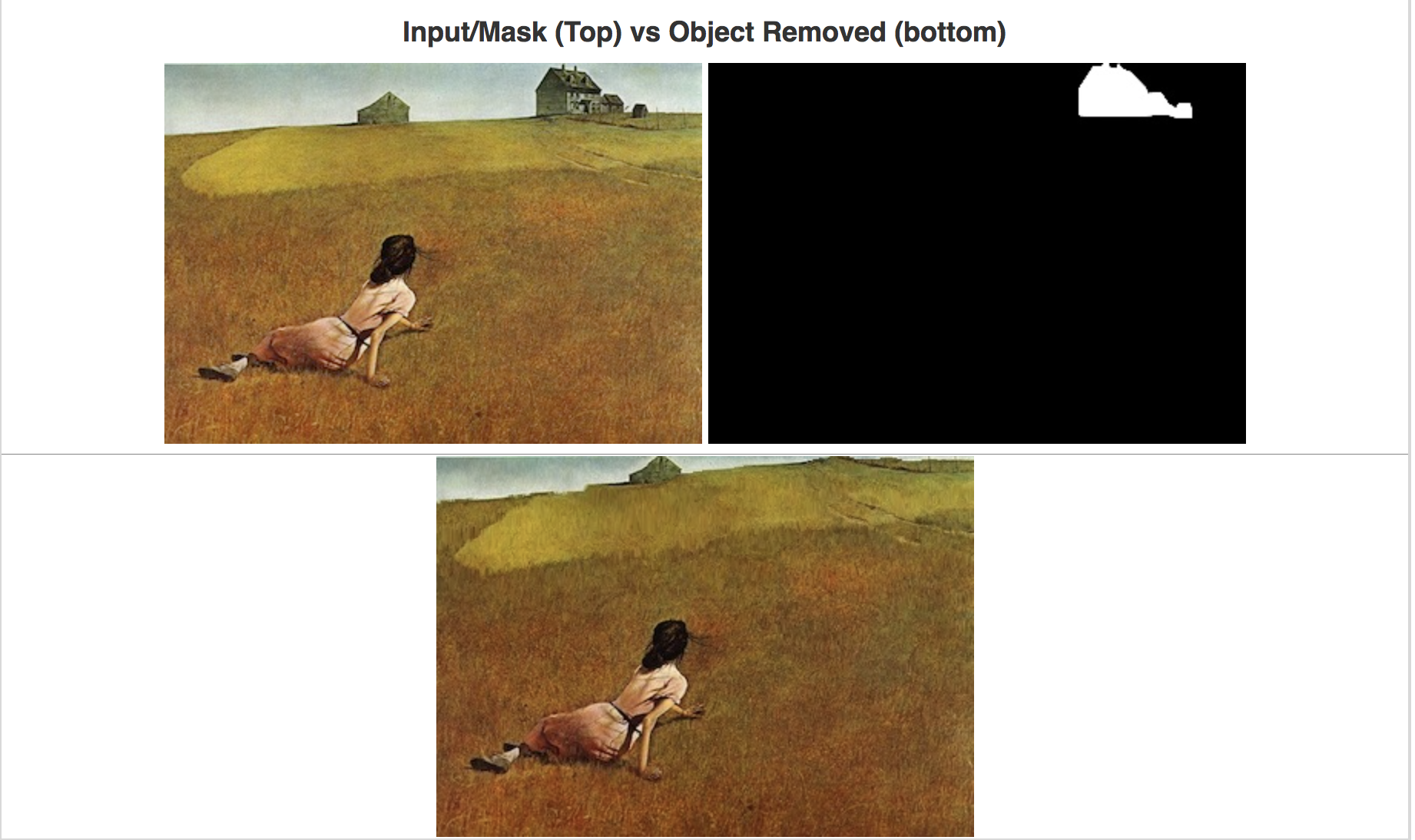
I notice that the result is not perfect, below is the picture provided by.
http://cs.brown.edu/courses/cs129/results/proj3/taox/
In the top right have some subtle change. I don't know how to do better. If you have some ideas
to improve it, we could achieve best result.
The text was updated successfully, but these errors were encountered: