-
Notifications
You must be signed in to change notification settings - Fork 12.3k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Rollup of 10 pull requests #78197
Closed
Closed
Rollup of 10 pull requests #78197
Conversation
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This resolves issues where the cross-build of LLVM fails because it tries to link to the host's system libraries instead of the target's system libraries.
Writing any fmt::Arguments would trigger the inclusion of usize formatting and padding code in the resulting binary, because indexing used in fmt::write would generate code using panic_bounds_check, which prints the index and length. These bounds checks are not necessary, as fmt::Arguments never contains any out-of-bounds indexes. This change replaces them with unsafe get_unchecked, to reduce the amount of generated code, which is especially important for embedded targets.
In this instance, we can just pass a &str slice and save an allocation.
.into() guarantees safety of the conversion. Furthermore, the minimum value of all uints is known to be 0.
Since having enabled the download-ci-llvm option, and having rebased on top of f05b47c, I've noticed that I had to update the llvm-project submodule manually if it was checked out. Orignally, the submodule update logic was introduced to reduce the friction for contributors to manage the submodules, or in other words, to prevent getting PRs that have unwanted submodule rollbacks because the contributors didn't run git submodule update. This commit adds logic to ensure there is no inadvertent LLVM submodule rollback in a PR if download-ci-llvm (or llvm-config) is enabled. It will detect whether the llvm-project submodule is initialized, and if so, update it in any case. If it is not initialized, behaviour is kept to not do any update/initialization. An alternative to the chosen implementation would be to not pass the --init command line arg to `git submodule update` for the src/llvm-project submodule. This would show a confusing error message however on all builds with an uninitialized repo. We could pass the --silent param, but we still want it to print something if it is initialized and has to update something. So we just do a manual check for whether the submodule is initialized.
It checks that fmt::write by itself doesn't pull in any panicking or or display code.
Closes #77062
Mark inout asm! operands as used in liveness pass Variables used in `inout` operands in inline assembly (that is, they're used as both input and output to some arbitrary assembly instruction) are being marked as read and written, but are not marked as being used in the RWU table during the liveness pass. This can result in such expressions triggering an unused variable lint warning. This is incorrect behavior- reads without uses are currently only used for compound assignments. We conservatively assume that an `inout` operand is being read and used in the context of the assembly instruction. Closes #77915
Haiku: explicitly set CMAKE_SYSTEM_NAME when cross-compiling This resolves issues where the cross-build of LLVM fails because it tries to link to the host's system libraries instead of the target's system libraries.
…514,Nemo157 Greatly improve display for small mobile devices screens Fixes #78014. The biggest change being the "search bar". Instead of having everything on one line, I decided to move the search input on its own: 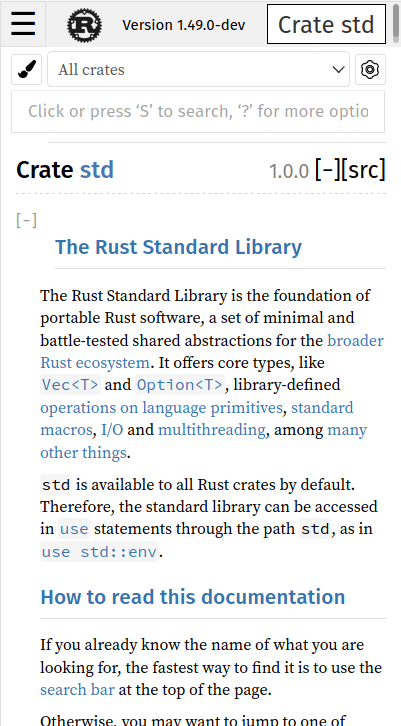 Another change is that now, we "break words" in the listing so that they don't grow too big: 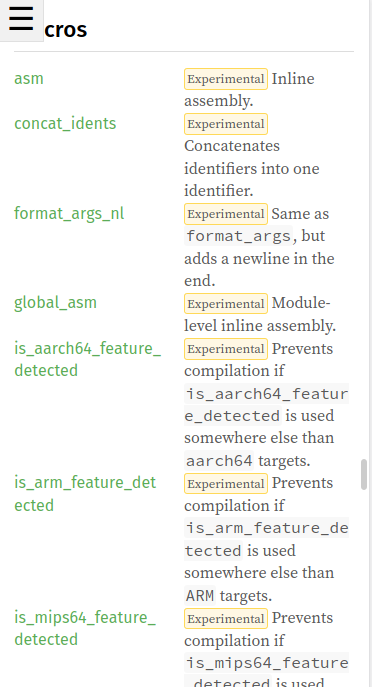 r? @jyn514
…eck, r=Mark-Simulacrum Avoid panic_bounds_check in fmt::write. Writing any fmt::Arguments would trigger the inclusion of usize formatting and padding code in the resulting binary, because indexing used in fmt::write would generate code using panic_bounds_check, which prints the index and length. These bounds checks are not necessary, as fmt::Arguments never contains any out-of-bounds indexes. This change replaces them with unsafe get_unchecked, to reduce the amount of generated code, which is especially important for embedded targets. --- Demonstration of the size of and the symbols in a 'hello world' no_std binary: <details> <summary>Source code</summary> ```rust #![feature(lang_items)] #![feature(start)] #![no_std] use core::fmt; use core::fmt::Write; #[link(name = "c")] extern "C" { #[allow(improper_ctypes)] fn write(fd: i32, s: &str) -> isize; fn exit(code: i32) -> !; } struct Stdout; impl fmt::Write for Stdout { fn write_str(&mut self, s: &str) -> fmt::Result { unsafe { write(1, s) }; Ok(()) } } #[start] fn main(_argc: isize, _argv: *const *const u8) -> isize { let _ = writeln!(Stdout, "Hello World"); 0 } #[lang = "eh_personality"] fn eh_personality() {} #[panic_handler] fn panic(_: &core::panic::PanicInfo) -> ! { unsafe { exit(1) }; } ``` </details> Before: ``` text data bss dec hex filename 6059 736 8 6803 1a93 before ``` ``` 0000000000001e00 T <T as core::any::Any>::type_id 0000000000003dd0 D core::fmt::num::DEC_DIGITS_LUT 0000000000001ce0 T core::fmt::num::imp::<impl core::fmt::Display for u64>::fmt 0000000000001ce0 T core::fmt::num::imp::<impl core::fmt::Display for usize>::fmt 0000000000001370 T core::fmt::write 0000000000001b30 t core::fmt::Formatter::pad_integral::write_prefix 0000000000001660 T core::fmt::Formatter::pad_integral 0000000000001350 T core::ops::function::FnOnce::call_once 0000000000001b80 t core::ptr::drop_in_place 0000000000001120 t core::ptr::drop_in_place 0000000000001c50 t core::iter::adapters::zip::Zip<A,B>::new 0000000000001c90 t core::iter::adapters::zip::Zip<A,B>::new 0000000000001b90 T core::panicking::panic_bounds_check 0000000000001c10 T core::panicking::panic_fmt 0000000000001130 t <&mut W as core::fmt::Write>::write_char 0000000000001200 t <&mut W as core::fmt::Write>::write_fmt 0000000000001250 t <&mut W as core::fmt::Write>::write_str ``` After: ``` text data bss dec hex filename 3068 600 8 3676 e5c after ``` ``` 0000000000001360 T core::fmt::write 0000000000001340 T core::ops::function::FnOnce::call_once 0000000000001120 t core::ptr::drop_in_place 0000000000001620 t core::iter::adapters::zip::Zip<A,B>::new 0000000000001660 t core::iter::adapters::zip::Zip<A,B>::new 0000000000001130 t <&mut W as core::fmt::Write>::write_char 0000000000001200 t <&mut W as core::fmt::Write>::write_fmt 0000000000001250 t <&mut W as core::fmt::Write>::write_str ```
…ulacrum Sync LLVM submodule if it has been initialized Since having enabled the download-ci-llvm option, and having rebased on top of #76864, I've noticed that I had to update the llvm-project submodule manually if it was checked out. Orignally, the submodule update logic was introduced to reduce the friction for contributors to manage the submodules, or in other words, to prevent getting PRs that have unwanted submodule rollbacks because the contributors didn't run git submodule update. This commit adds logic to ensure there is no inadvertent LLVM submodule rollback in a PR if download-ci-llvm (or llvm-config) is enabled. It will detect whether the llvm-project submodule is initialized, and if so, update it in any case. If it is not initialized, behaviour is kept to not do any update/initialization. An alternative to the chosen implementation would be to not pass the --init command line arg to `git submodule update` for the src/llvm-project submodule. This would show a confusing error message however on all builds with an uninitialized repo. We could pass the --silent param, but we still want it to print something if it is initialized and has to update something. So we just do a manual check for whether the submodule is initialized.
Fix two small issues in compiler/rustc_lint/src/types.rs Two small improvements of `compiler/rustc_lint/src/types.rs`
Update cargo 3 commits in 79b397d72c557eb6444a2ba0dc00a211a226a35a..dd83ae55c871d94f060524656abab62ec40b4c40 2020-10-15 14:41:21 +0000 to 2020-10-20 19:31:26 +0000 - Support glob patterns for package/target selection (rust-lang/cargo#8752) - Update env_logger requirement from 0.7.0 to 0.8.1 (rust-lang/cargo#8795) - Fix man page links inside `option` blocks. (rust-lang/cargo#8793)
📌 Commit 891f3f7 has been approved by |
⌛ Testing commit 891f3f7 with merge bc72d45d477acd26de0acf9a24a4504326b086b3... |
💔 Test failed - checks-actions |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Labels
rollup
A PR which is a rollup
S-waiting-on-review
Status: Awaiting review from the assignee but also interested parties.
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
Successful merges:
rustfmt
#78156 (Fixed build failure ofrustfmt
)[(); usize::MAX]
#77062)Failed merges:
r? @ghost