-
-
Notifications
You must be signed in to change notification settings - Fork 9.4k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #49291 [Serializer] Add methods
getSupportedTypes
to allow …
…better performance (tucksaun, nicolas-grekas) This PR was merged into the 6.3 branch. Discussion ---------- [Serializer] Add methods `getSupportedTypes` to allow better performance | Q | A | ------------- | --- | Branch? | 6.3 | Bug fix? | no | New feature? | yes | Deprecations? | yes (new method in the interfaces, one interface deprecated) | License | MIT | Doc PR | to be written The PRs allows normalizers or denormalizers to expose their supported types and the associated cacheability to the Serializer. With this info, even if the `supports*` call is not cacheable, the Serializer can skip a ton of method calls to `supports*` improving performance substaintially in some cases: 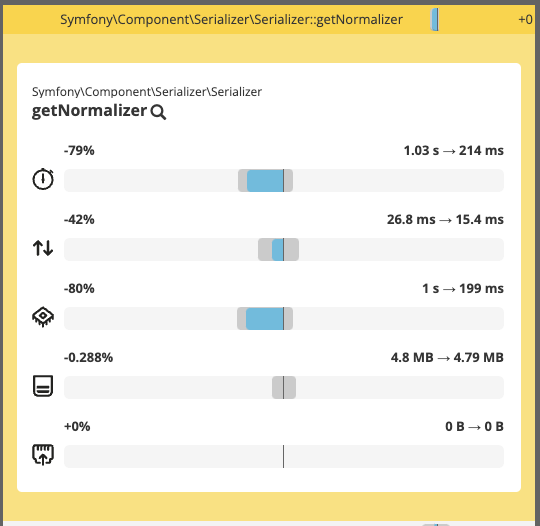 <details> <summary>I found this design while working on a customer project performance (a big app built around API Platform): we reached the point where the slowest part of main application endpoint was `Symfony\Component\Serializer\Serializer::getNormalizer`.</summary> After some digging, we found out we were experiencing the conjunction of two phenomenons: - the application is quite complex and returns deep nested and repeating structures, exposing the underlying bottleneck; - and a lot of custom non-cacheable normalizers. Because most of the normalizers are not cacheable, the Serializer has to call every normalizer over and over again leading `getNormalizer` to account for 20% of the total wall time: 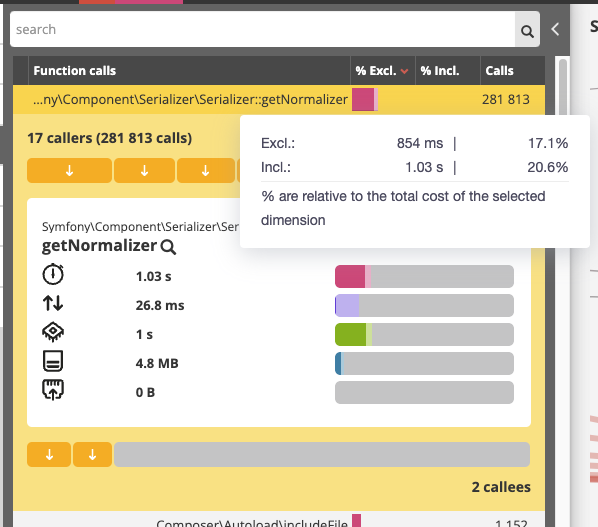 We first tried to improve cacheability based on context without much success, then an approach similar to #45779 with some success but still feeling this could be faster. We then thought that even if the `supportsNormalization` could not be cached (because of the context), maybe we could avoid the calls at the origin by letting the `Normalizers` expose the types they support and came to this PR with pretty good results. </details> The perfornance improvement was only measured by adapting Symfony's normalizers as well as the project ones, proper third party normalizers updates should improve performance even more. This should effectively replaces the `CacheableSupportsMethodInterface` as the cacheability can now be returned by `getSupportedTypes`. Commits ------- e5af24a [Serializer] Add wildcard support to getSupportedTypes() 400685a [Serializer] Add methods `getSupportedTypes` to allow better performance
- Loading branch information
Showing
41 changed files
with
619 additions
and
78 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.