-
Notifications
You must be signed in to change notification settings - Fork 212
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: add sql query to obtain balance (#3446)
Description --- - Replaced the `get_balance` function containing multiple sql queries with a single raw sql query. - Removed redundant code (`fn fetch_pending_outgoing_outputs`) as a result of this change. - Added more test points for time-locked balance. - **Note:** To properly test transaction validation in `async fn test_txo_validation()`, access to the backend to obtain pending incoming transactions is needed via ` fn fetch_pending_incoming_outputs`, although that function is not used in production code anymore. If it is also removed other methods will have to be added to the backend to obtain the data for testing. Retaining the current function was chosen in lieu of adding other code. Motivation and Context --- Get balance used a lot of RAM and was really slow due to multiple database interactions. Comparison of old vs. new query time performance for a wallet with 251,000 UTXOs in the database shown below: - **Full scale** 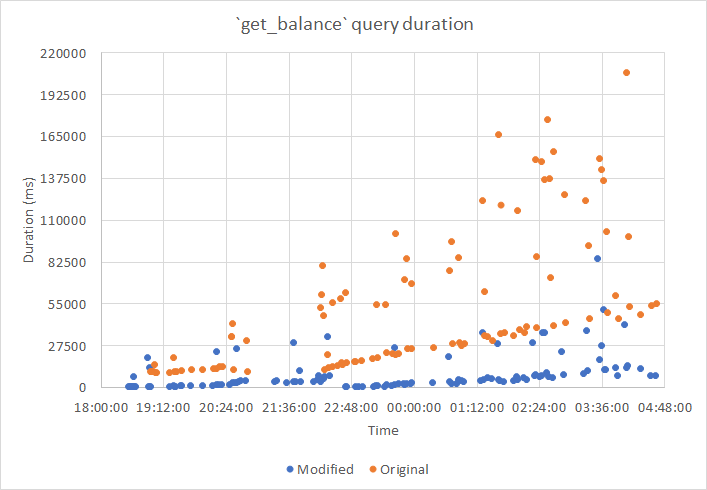 - **Y-axis zoomed in** 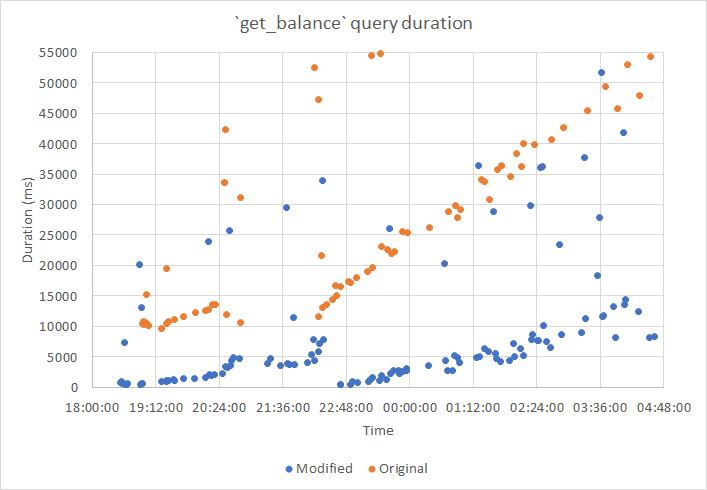 How Has This Been Tested? --- - Unit tests - System level tests
- Loading branch information
1 parent
43b2033
commit e23ceec
Showing
4 changed files
with
135 additions
and
83 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters