-
-
Notifications
You must be signed in to change notification settings - Fork 44
Can't query for or fire events on mocked components #17
Comments
Hey thanks for the detailed report, and we're happy you checked the library out! Just like dom-testing-library, this library won't accept events on implementations like your own components. In your repro, the real Snapshots output in 2.0 isn't great, which is something we're addressing in the upcoming 3.0 release that should be landing any day now. I'm just putting some finishing touches on it and polishing up the docs to get it out the door. That said, the 3.0 release does dig in more on testing only the native components, not implementation components. I think it's much more aligned to the guiding principles and you'll love it once it's out Sort of related, as a general rule of thumb, in the testing-library world, testIDs aren't a preferred way to search . Check out this page or this page for more info about that. Usually there's a better user-centric query that will help you isolate an event target. All of that said, this is what I'd recommend for your tests be:
These pass, other than the fact buttonHandler just console logs, but it can't pass because it's not a Good luck to you, and keep an eye out for 3.0! |
Responded on #12 as well now that 3.0 is released, and wanted to show you what things would be like using the latest. Given these tests: import React from 'react';
import { render, fireEvent } from 'native-testing-library';
import { buttonHandler } from './buttonHandler';
import { ComponentUnderTest } from './ComponentUnderTest';
it('should match snapshot', () => {
const { container } = render(<ComponentUnderTest />);
expect(container.children[0]).toMatchSnapshot();
});
it('should press button', () => {
const { getByText } = render(<ComponentUnderTest />);
fireEvent.press(getByText('Main Content'));
expect(buttonHandler).toHaveBeenCalled();
}); this is the snapshot output:
Much more reasonable, huh? import React from 'react';
import { render, fireEvent } from 'native-testing-library';
import { buttonHandler } from './buttonHandler';
import { ComponentUnderTest } from './ComponentUnderTest';
jest.mock('./ButtonWrapper', () => ({
ButtonWrapper: ({ children, ...props }) =>
require('react').createElement('ButtonWrapper', props),
}));
it('should match snapshot', () => {
const { container } = render(<ComponentUnderTest />);
expect(container.children[0]).toMatchSnapshot();
}); The important thing is not passing children to your mock, otherwise it's just a regular deep render with a wasted mock. It's not really good for anything other than getting a shallow snapshot, honestly. Here's the output:
Hope it helps, good luck on the transition from Enzyme with your team! |
Thanks for the detailed responses 😃 I'll address your specific responses before getting back to the bigger question. Ya we have a huge codebase of enzyme shallow tests... Diving through HOCs is annoying but maybe that'll get better with hooks (if enzyme ever supports them). Deep rendering and then mocking everything below the component under test seemed like a good way to approximate a shallow test. I'm just starting to slowly convert things to TypeScript, Hooks, GraphQL, and some testing library that supports those. Rewriting tests that have been focused on implementation detail is going to be an undertaking. I'm just trying to figure out how to best get started. It'll be a process over time. Hooks make it harder to write tests that depend on the component instance anyway which you can't get at with hooks and functional components. Thanks for the work on improving the snapshots and the 3.0 release! I'll have to try it sometime soon. Ya the testIDs here were just for the example. But I think I'm reaching for them cuz I'm still trying to interact with the implementation components, not the native components in some cases. The middle test was just me proving to myself that I could query for children INSIDE a mocked component. In reality the snapshot would cover testing for that. I wasn't really sure what my issue was at the beginning. Hopefully an improved rendered output in the unable to find element error message makes it clearer what you can and can't query. When I was mocking a child component, I was interested in rendering the children since they are IN my component since they won't get tested anywhere else. That's a good way to toss the children though if I need to. Returning a string is a super simple way to mock a component but still get the children. Your comment in the other issue about firing a scroll event on any child of the FlatList makes sense and will be helpful. I was wondering where it was possible to fire that... I guess I'm struggling with the testing-library world forcing me out of writing unit tests and into writing integration tests, especially in light of the existing codebase. I've also got a co-worker who believes very strongly in writing unit tests for everything and having them only interact with the unit and not anything else outside. I think I was coming here with a solution/bug fix in mind instead of presenting the complete problem 😬 Starting a conversation about the merits of the testing-library approach is probably not the most appropriate in an issue in a library for one specific implementation but I'll fire off one message and maybe you can point me to the best place to ask about it haha (Stack Overflow/other repo/chat/etc). I'm also trying to convince myself strongly enough of this pattern to be able to convince a coworker :) So I guess my concerns are:
All that to say, I think you resolved my initial question and the issue with "that doesn't fit our guiding principles". So feel free to close the issue :) But I would like to continue this conversation/learn more. I'm not opposed to any of the guiding principles and do see the value in them, I'm just trying to figure out how to best apply them to a large codebase. At this point, I'm not convinced that I can test what I want to in a sane way without some amount of mocking, in which case maybe I should stick with I suppose I could make mock components that would, say, hook up the Thanks for reading all this lol. I've appreciated your thoughts so far 👍 |
Edit: sorry I closed this early, clicked the button on accident while I was writing my comment lol. Still going to close just for housekeeping’s sake, since the original concern is addressed. I totally get where you’re coming from, so I’ll try to address a few of the biggest concerns.
Hopefully that helps, I definitely felt the pain points as well going through a transition from Enzyme. Biggest thing to keep in mind, it’s a completely different mentality and tests you currently have likely won’t map 1:1 to testing library; there’s probably a lot of tests you could/should get rid of in favor of something that’s more focused around user actions and outcomes. I won’t try to convince you to use NTL over RNTL because I’ve always believed there’s merits to both. I think it’s ultimately about what helps your team be confident your app is working. I can tell you there will be trade offs, but if you’ve not run into any issues with it and you’re generally happier with it, no complaints here, and I’m happy it’s working for you guys 👍 the only alternative I could give you to consider (just for the sake of helping you make a decision, not to convince you) is using NTL for most tests and importing the react-test-renderer directly to shallow render for snapshots, which is totally possible to ease a transition. If you make a custom utility like I linked earlier, you could even export shallow from there so most of the team wouldn’t even care where the shallow renderer was coming from. Hope this helps, and best of luck to you and your team in your huge overhaul! |
One thing I'd add here to what Brandon said - I'd encourage you to read Martin Fowler's article on mocking and stubbing, and specifically his discussion about the difference between "classical" and "mockist" testing. Component tests in testing-library world tend to still be unit tests, but they're unit tests under the classical philosophy. That is, they test a single "unit" (a component), but they allow that unit to exercise its full behavior and then verify the state of things after it does so. One dumb example I like to point to for this: Imagine you have an function calculate() {
return add(3, 5);
}
function add(x, y) {
return x + y;
} or function calculate() {
return 3 + 5;
} These two implementations are equivalent in terms of how they behave. On the naive version of the mockist testing approach, though, you'd actually write different tests for each! (Viz., for the first you'd mock out the add function, but for the latter you wouldn't). That means that the tests you write for a feature are tightly coupled to the implementation, then (since the only difference here is a difference in implementation). On the classical testing philosophy, the tests for |
react-native
orexpo
: Both. My project isreact-native
but the repro isexpo
. Everything below is for the repro.native-testing-library
version:2.1.0
react-native
version: https://github.com/expo/react-native/archive/sdk-32.0.0.tar.gznode
version:12.1.0
yarn
version:1.15.2
Relevant code or config:
Component:
Test:
What you did:
Tried to use
getByTestId
to find the mocked component.What happened:
Test output:
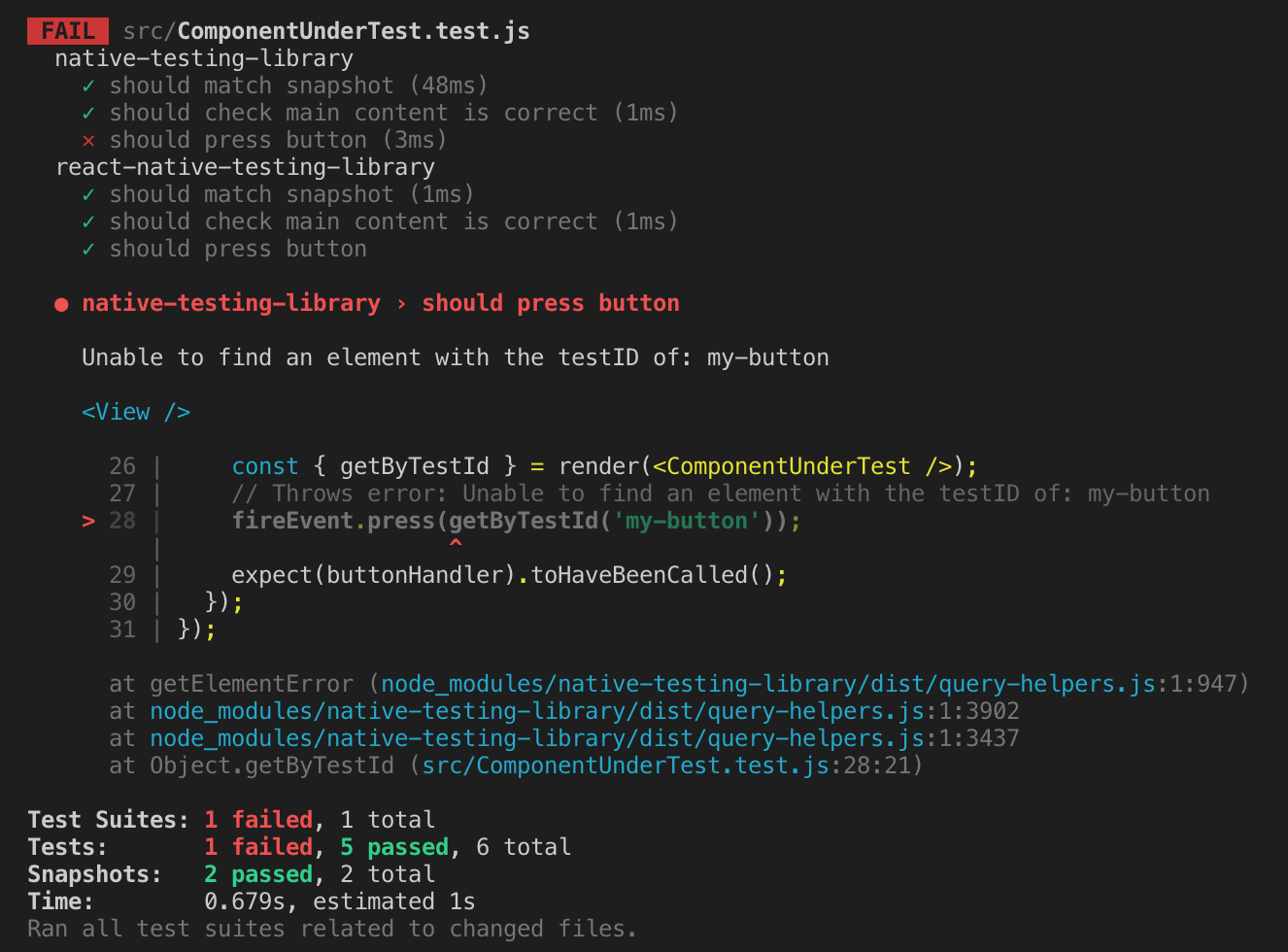
Reproduction:
https://github.com/OzzieOrca/repro-native-testing-library-mock-wrapper
Problem description:
Our repo is filled with wrappers around native components and complicated child components. Being able to mock these makes the snapshot output much cleaner and prevents us from having to mock everything needed to render a child component.
react-native-testing-library
works fine for finding and firing events on these mocked components.native-testing-library
doesn't seem to be able to query for these components.Suggested solution:
<View />
(hopefully that's what it's meant to be) which doesn't match the snapshot or the output ofdebug()
. I spent a while trying to figure out why my component wasn't rendering anything more than just the root element before realizing it actually was. This error is misleading. If you have a typo in the test id, it won't show you the rendered output with the correct ID and could lead you down a wild goose chase before finding the real issue.Thanks for your help with this! Just trying to figure out the best way to test modern React Native components and your library seems promising :)
The text was updated successfully, but these errors were encountered: