-
Notifications
You must be signed in to change notification settings - Fork 75
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Feature]: Curp Refactoring #169
Comments
Close #73 |
What‘s the meaning of the relevant context? |
Just miscellanea that will be used in /// Relevant context for Curp
struct Context<C: Command> {
/// Id of the server
id: ServerId,
/// Other server ids and addresses
others: HashSet<ServerId>,
/// Timeout
timeout: Arc<ServerTimeout>,
/// Cmd board for tracking the cmd sync results
cb: CmdBoardRef<C>,
/// Speculative pool
sp: SpecPoolRef<C>,
/// Tx to send leader changes
leader_tx: broadcast::Sender<Option<ServerId>>,
/// Election tick
election_tick: AtomicU8,
/// Heartbeat opt out flag
hb_opt: AtomicBool,
/// Tx to send cmds to execute and do after sync
cmd_tx: Box<dyn CmdExeSenderInterface<C>>,
} |
What's the difference between |
pub(super) struct State {
/* persisted state */
/// Current term
pub(super) term: u64,
/// Candidate id that received vote in current term
pub(super) voted_for: Option<ServerId>,
/* volatile state */
/// Role of the server
pub(super) role: Role,
/// Cached id of the leader.
pub(super) leader_id: Option<ServerId>,
}
pub(super) struct CandidateState<C> {
/// Collected speculative pools, used for recovery
pub(super) sps: HashMap<ServerId, Vec<Arc<C>>>,
/// Votes received in the election
pub(super) votes_received: u64,
}
pub(super) struct LeaderState {
/// For each server, index of the next log entry to send to that server
pub(super) next_index: HashMap<ServerId, usize>,
/// For each server, index of highest log entry known to be replicated on server
pub(super) match_index: HashMap<ServerId, usize>,
} Why Mutex for candidate state?
|
FYI: the pub(super) struct Log<C: Command> {
/// Log entries
pub(super) entries: Vec<LogEntry<C>>,
/// Index of highest log entry known to be committed
pub(super) commit_index: usize,
/// Index of highest log entry applied to state machine
pub(super) last_applied: usize,
} |
move propose logic from curp_node to raw_curp
move propose logic from curp_node to raw_curp
move propose logic from curp_node to raw_curp
move propose logic from curp_node to raw_curp
move propose logic from curp_node to raw_curp
Motivations
Currently, our curp server has the following pain points that might slow down our later developments:
LockGuard
scope issue. Given thatLockGuard
must be released at theawait
point, we must take special care in how we structure the code, which results in opaque and nested code that is difficult both for the developer to write and for reviewers to read. Things would go more smoothly if we could divide our code into two parts: the non-async (update of curp's state) component and the async (transport, io) half.Approach
As a solution, I suggest introducing a new abstraction layer called
RawCurp
.RawCurp
can be viewed as a state machine. It is purely non-async. So the receiving and sending of requests and responses will be handled outside ofRawCurp
.It looks like this:
Before refactoring:
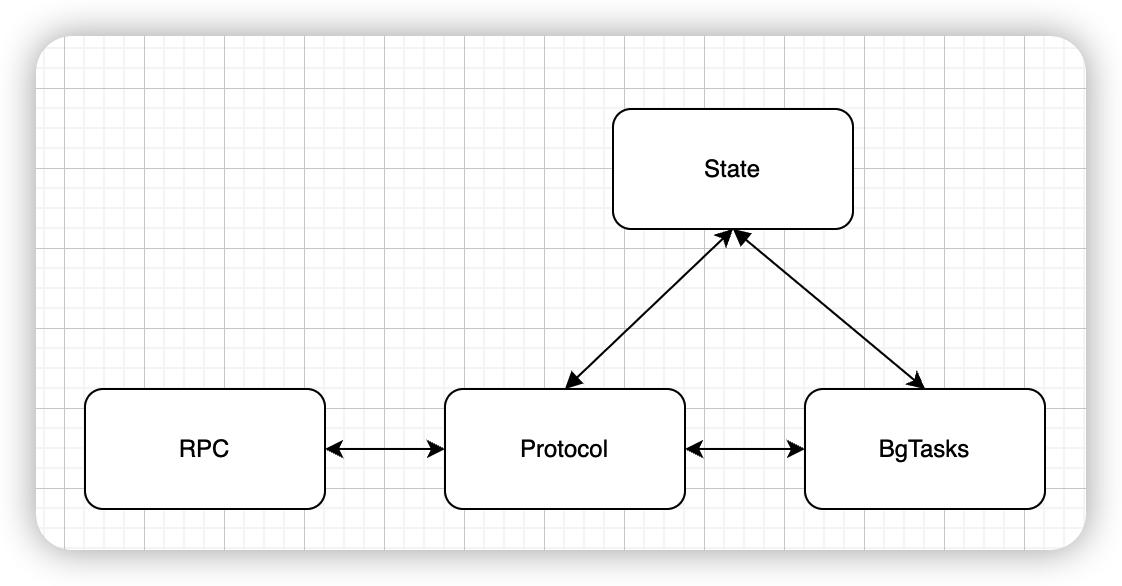
Protocol
,State
,bg_tasks
are messed up togetherAfter refactoring:
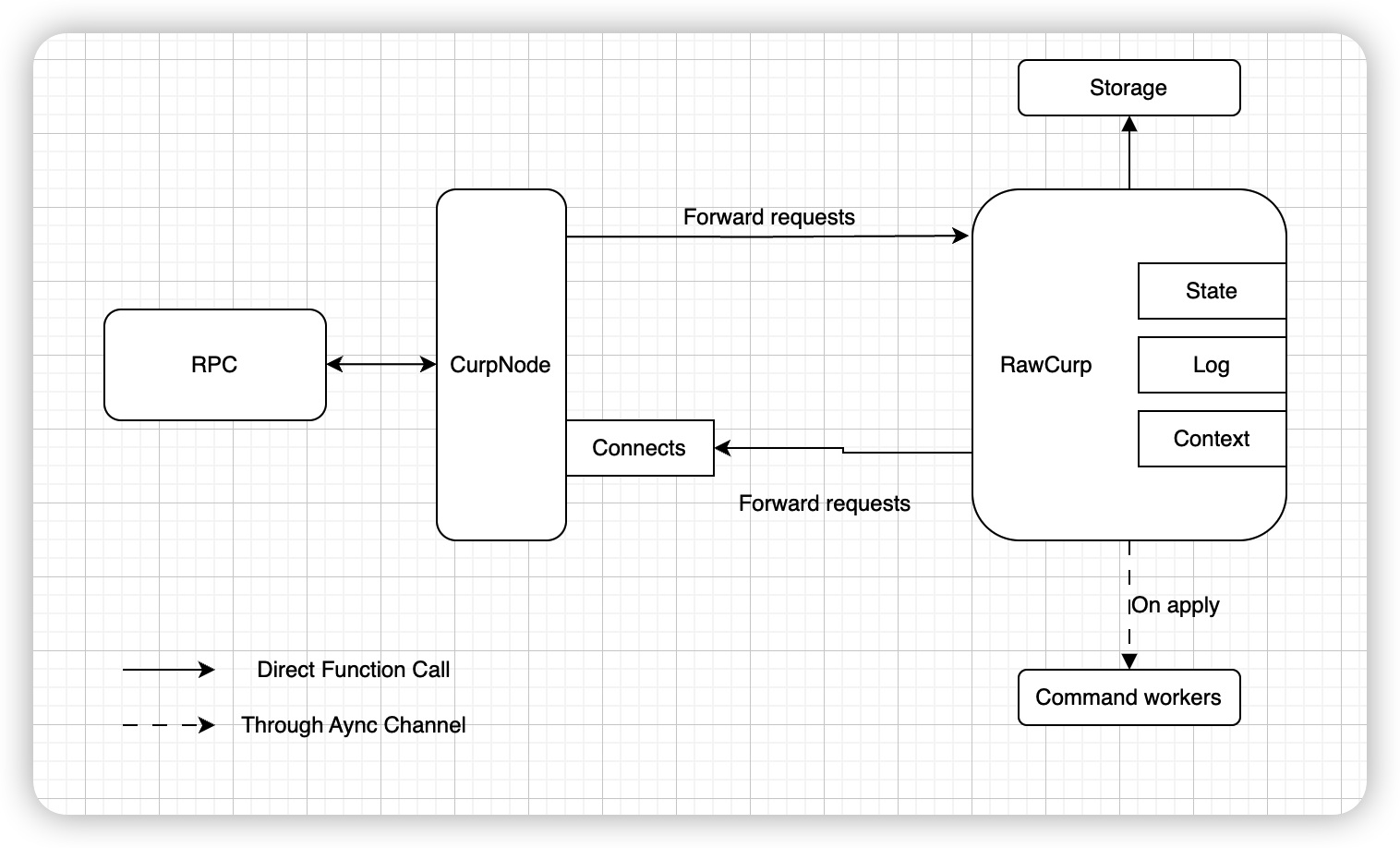
CurpNode
will handle all transport-related functionalities and error handlings, whileRawCurp
only needs to take care of internal structures and their consistencies.I plan to deliver this refactor in 4 prs.
RawCurp
without removing thebg_tasks
: I will try my best to reserve the currentbg_tasks
interface and replace the inner code with theRawCurp
. This will help reduce the burden of reviewers.bg_tasks
; refactorLogEntry
: only one cmd for each log entry and it should contain index; improve error handling.P.S. Although I will remove the
bg_tasks
in the end, it doesn't mean that there will be no background tasks inCurpNode
. In fact, there will still bebg_tick
andbg_leader_calibrate_follower
, but since most logics are handled in thecurp
module, there will be not much code.About Locks in
RawCurp
RawCurp
has 4 locks:Code of Conduct
The text was updated successfully, but these errors were encountered: