We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
适配器模式是指两个互不相关的类或者接口通过适配器进行关联的模式,属于结构型设计模式。 其作用就是让两个互不相关的类或者接口能够互相通信,启到桥梁的作用。主要有类适配器模式、类对象适配器模式和接口对象适配器模式。
//待适配的类
public class Source { public void f1(){ System.out.println("Source-f1"); } }
//目标接口
public interface Target { void f1(); //注意这里的方法签名和待适配类中方法一致 void f2(); }
//适配器(Adapter)类
public class Adapter extends Source implements Target { @Override public void f2() { System.out.println("Adapter-f2"); } }
//调用
Target target = new Adapter(); target.f1(); target.f2();
关键点:
//待适配接口
public interface Source3 { void f1(); void f2(); }
//待适配接口实现类
public class Source3Impl implements Source3 { @Override public void f1() { System.out.println("f1"); } @Override public void f2() { System.out.println("f2"); } }
public interface Target3 { void f1(); void f2(); }
Source2 source2 = new Source2(); Target2 target2 = new Adapter2(source2); target2.f1(); target2.f2();
public class Source2 { public void f1(){ System.out.println("Source2-f1"); } }
public interface Target2 { void f1(); void f2(); }
public class Adapter3 implements Target3 { private Source3 mSource3; public Adapter3(Source3 source3) { this.mSource3 = source3; } @Override public void f1() { mSource3.f1(); } @Override public void f2() { mSource3.f2(); } }
Source3 source3 = new Source3Impl(); Target3 target3 = new Adapter3(source3); target3.f1(); target3.f2();
在java JDK中InputStreamReader和OutputStreamWriter;在Android SDK中ArrayAdapter、BaseAdapter、ListAdapter等都使用了大量的适配器模式。
适配器模式使得两个互不相干的类或接口能进行通信;适配器模式主要有三个要素,确定待适配的对象、目标对象、适配器对象(关联桥梁类)。适配器模式只适用一方访问另一方的情形,即是单向的。
The text was updated successfully, but these errors were encountered:
No branches or pull requests
1. 什么是适配器模式?有什么作用?
适配器模式是指两个互不相关的类或者接口通过适配器进行关联的模式,属于结构型设计模式。
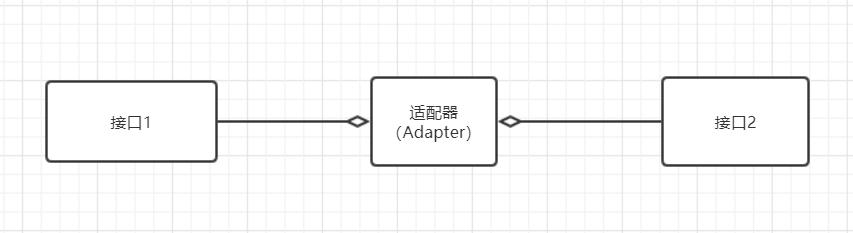
其作用就是让两个互不相关的类或者接口能够互相通信,启到桥梁的作用。主要有类适配器模式、类对象适配器模式和接口对象适配器模式。
2. 如何实现?
2.1 类适配器模式
//待适配的类
//目标接口
//适配器(Adapter)类
//调用
关键点:
2.2 对象适配器模式
//待适配接口
//待适配接口实现类
//目标接口
//适配器(Adapter)类
//调用
关键点:
2.3 接口对象适配器模式
//待适配的类
//目标接口
//适配器(Adapter)类
//调用
关键点:
3. JDK或Android的应用举例
在java JDK中InputStreamReader和OutputStreamWriter;在Android SDK中ArrayAdapter、BaseAdapter、ListAdapter等都使用了大量的适配器模式。
4. 小结
适配器模式使得两个互不相干的类或接口能进行通信;适配器模式主要有三个要素,确定待适配的对象、目标对象、适配器对象(关联桥梁类)。适配器模式只适用一方访问另一方的情形,即是单向的。
The text was updated successfully, but these errors were encountered: