-
-
Notifications
You must be signed in to change notification settings - Fork 104
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Bug Fix] Scan Heap Blocks #3
base: master
Are you sure you want to change the base?
Conversation
by the way, if you want to walk over the segment entry list, you have to |
and the fix code only work for |
Very cool. Thanks for that. Do we need to use the xor key to get the size? Can't we just query the base address to get the allocated memory size for that block? |
microsoft seem not provide this function(get the heap block size), but u can use |
In addition to using HeapWalk, you can also use heap32first and heap32next to accomplish this, but it is very slow, and cannot scan 32bit process from 64bit process. heap32next is slow beacause it call RtlQueryProcessDebugInfomation when you call it every time or if you want to faster enum heap, you can implement heap32first and heap32next via RtlQueryProcessDebugInfomation But, there's same problem, it cannot scan 32bit process from 64bit process. I haven't found the solution yet. |
@d1nfinite NtQueryInformationMemory should give the allocated base + size of any address. It might overshoot the amount actually allocated off the heap but it should cover the block it was allocated from |
@CCob beaconeye use
if |
you can see https://github.com/akkuman/EvilEye/blob/e7ca6eec4b52a7aa259d5e0bc8bafecd4e7922a9/beaconeye/beaconeye.go#L260 and https://github.com/akkuman/EvilEye/blob/e7ca6eec4b52a7aa259d5e0bc8bafecd4e7922a9/beaconeye/beaconeye.go#L236 , I did a little work to speed up the program |
In previous fix, you only scan the heap segments, but heap segments contains lots of heap blocks, so, if i create a beacon like this
it can bypass the beaconeye(random, if the beacon config not in first segment block), so, to solve this problem, should scan all heap blocks, but if process alloc large heap memory, it will be slow. (in my case, scan 16gb memory need 5 mins).
but the heap blocks don't exist linkedlist to walk over, so we have to calculate the block address, the block struct like this
in
x64
, the size isSize * 0x10
, so the block address like thisbut windows encrypt the size, so we have to use xor key to decrypt, xor key in
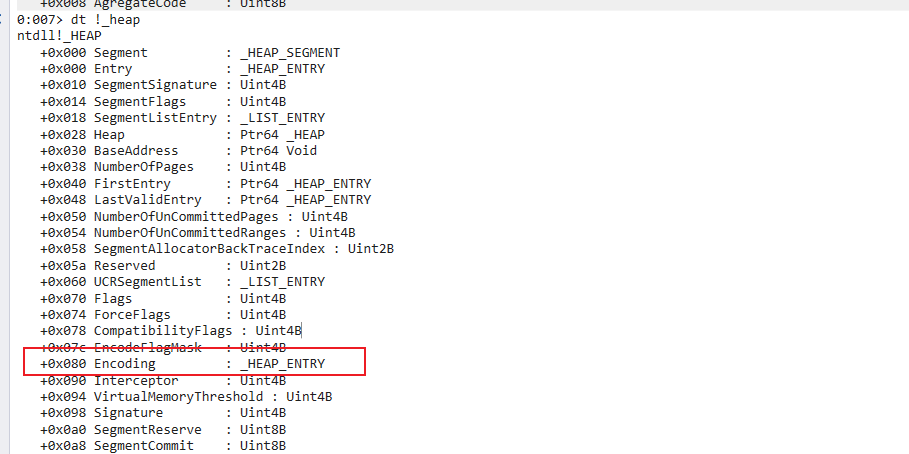
_heap
struct fieldsencoding
so, the fix code is my pr (my english is not good, srry)