-
Notifications
You must be signed in to change notification settings - Fork 49
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Linked modulators never process #133
Comments
ok, further digging reveals that the duplicate
I removed the instrument-level modulators and made them global preset modulators so only 2 modulators are used. |
ok, when testing with the above soundfont that contains only 2 global modulators (and no instrument-level modulators)
Then those 2 get linked in the lines that follow inside THEN when this line is called:
it doesn't matter that there are zero |
Here is a patch. The only problem with it is that the Modulator that is using another Modulator as its source needs to normalize the value passed to it from the other modulator. otherwise you'll end up with a huge
|
I'd like to add that the normalization needs to take into account that multiple modulators can be input to the same target modulator. They need to be summed up and the normalization needs to take the whole range of the sum into account. |
Looking at the code it seems like it is currently not prepared to handle normalization of linked modulators. I see here
that the value from one modulator is simply sent on to another. What is needed is to expand this to send the min and max for normalization, too, e.g.
and setInput() will need to sum up min and max in addition to result. Finally when the result is evaluated it will need to be normalized as (X-sum(min))/(sum(max)-sum(min)) to bring it into the 0 to 1.0 range. It's probably easiest to handle that directly in setInput()
like so
Regarding min and max for a modulator I'd like to mention that currently polyphone always prints "Add From:0 To:0" when the target is a linked modulator when instead it should be computed as an unadjusted number (basically like "Tuning(cents)" without the "cents"). |
@matkatmusic, @mbenkmann thank you very much for your analyze. It's been very helpful |
I created a simple soundfont to test out the modulation system.
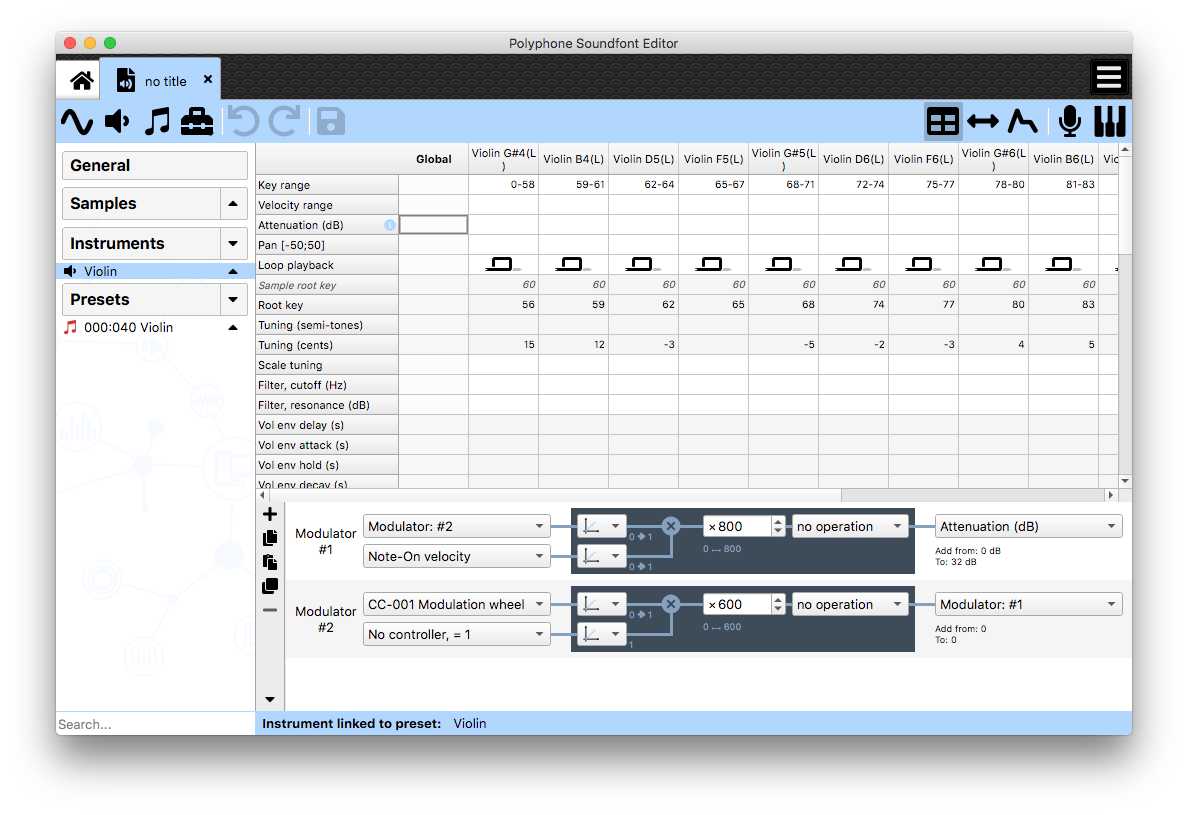
it has 2 modulators, and the 2nd modulator is linked to the first.
in this loop,
ok
always ends up false when the loop exits. This is easily verified by addingbool result = ok;
and a breakpoint:Somewhere
_inputNumber
is being set to2
in theParameterModulator
class, which prevents this line:from ever returning
true.
it seems that this loop in
ModulatorGroup::loadModulators()
is causingmodulator->setOutput(otherMod);
to be called twice for 'modulator'if you inspect
modulator
you'll see that itsinputNumber
gets set to2
for the attached soundFont.ModulationTest.sf2.zip
The text was updated successfully, but these errors were encountered: