-
Notifications
You must be signed in to change notification settings - Fork 2
04 DynamoDB
JP Barbosa edited this page Sep 25, 2022
·
1 revision
code ./stacks/resources/table.ts
import { Table, Stack } from "@serverless-stack/resources";
export const createTable = (stack: Stack) =>
new Table(stack, "table", {
fields: {
id: "string",
},
primaryIndex: { partitionKey: "id" },
});
code ./stacks/resources/queue.ts
import { ..., Table } from "@serverless-stack/resources";
...
type CreateQueueOptions = { bucket: Bucket; table: Table };
...
export const createQueue: CreateQueue = (stack, { bucket, table }) => {
...
const queue = new Queue(stack, "queue", {
consumer: {
function: {
...
environment: {
table: table.tableName,
},
},
},
});
queue.attachPermissions([bucket, table]);
return queue;
};
code ./services/functions/process.ts
...
import { ..., DynamoDB } from "aws-sdk";
...
const dynamoDb = new DynamoDB.DocumentClient();
const getLabels = async (...) => {
...
};
const saveLabels = async (
key: string,
labels?: Rekognition.Types.DetectLabelsResponse,
error?: AWSError
) => {
try {
const params = {
TableName: String(process.env.table),
Item: {
id: key,
createdAt: Date.now(),
labels,
error,
},
};
const result = await dynamoDb.put(params).promise();
console.log("Labels have been saved");
return result;
} catch (error) {
console.log(error);
}
};
const deleteImage = async (...) => {
...
};
export const handler: SQSHandler = async (sqsEvent) => {
sqsEvent.Records.forEach(async (record) => {
...
const { key } = detail.object;
try {
const labels = await getLabels(detail);
await saveLabels(key, labels);
} catch (error) {
const awsError = error as AWSError;
await saveLabels(key, undefined, awsError);
} finally {
await deleteImage(detail);
}
});
};
code ./stacks/resources/index.ts
...
export * from "./table";
code ./stacks/MyStack.ts
...
import {
...
createTable,
} from "./resources";
export function MyStack({ stack }: StackContext) {
const bucket = createBucket(stack);
const table = createTable(stack);
const queue = createQueue(stack, { bucket, table });
const bus = createBus(stack, { bucket, queue });
}
npm start # or press ENTER to redeploy if SST is already running
open https://console.sst.dev/sst-rekognition/sst-jp/local
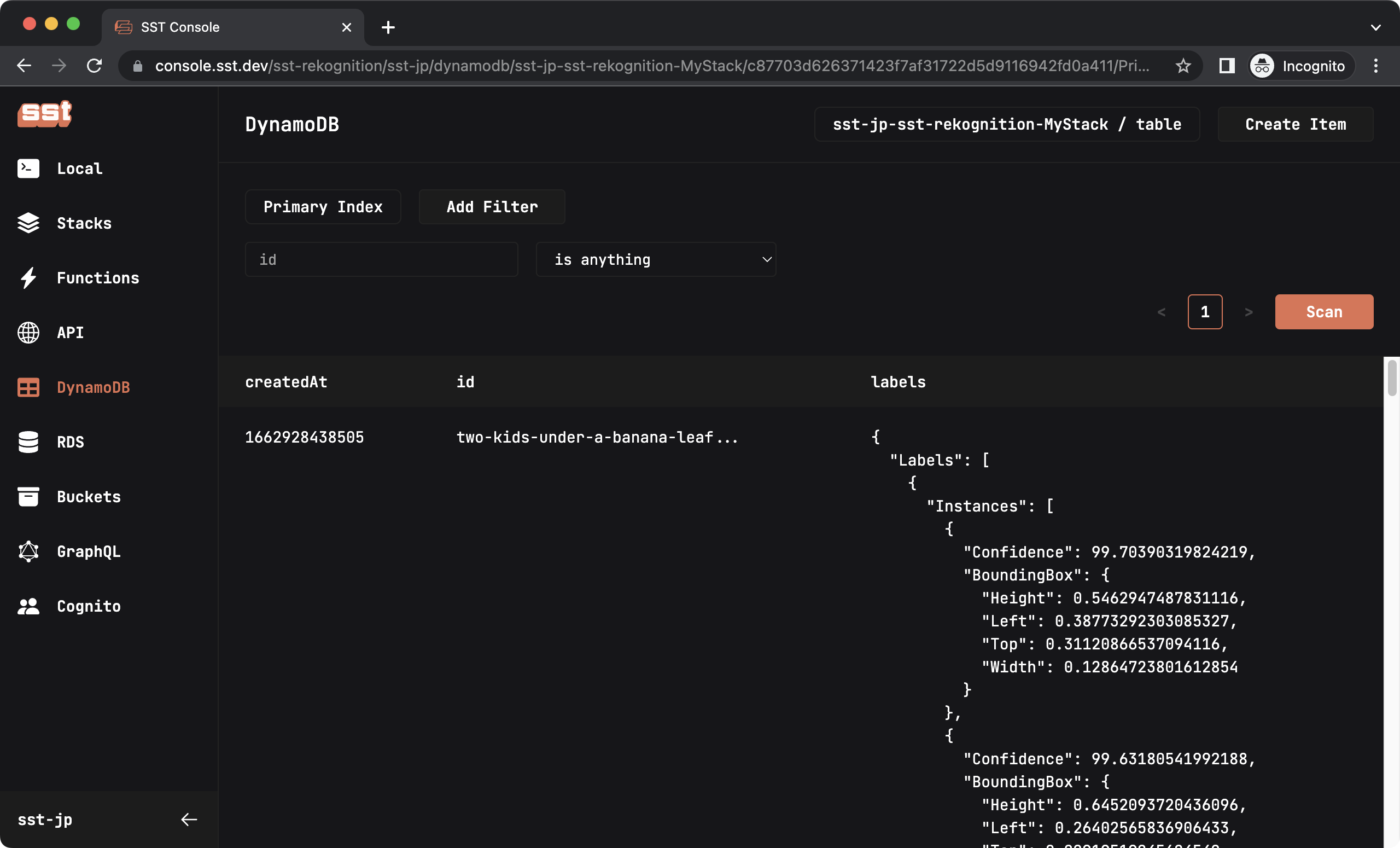
git add .
git commit -m "Dynamo DB"