-
Notifications
You must be signed in to change notification settings - Fork 2
09 Cognito Auth
JP Barbosa edited this page Sep 24, 2022
·
8 revisions
code ./stacks/resources/auth.ts
import { Cognito, Bucket, Stack } from "@serverless-stack/resources";
type CreateAuthOptions = { bucket: Bucket };
type CreateAuth = (stack: Stack, options: CreateAuthOptions) => Cognito;
export const createAuth: CreateAuth = (stack, { bucket }) => {
const auth = new Cognito(stack, "auth", {
identityPoolFederation: {
cdk: {
cfnIdentityPool: {
identityPoolName: "identityPool",
allowUnauthenticatedIdentities: true,
},
},
},
});
auth.attachPermissionsForUnauthUsers(auth, [bucket]);
return auth;
};
code ./stacks/resources/site.ts
import {
...
Cognito,
} from "@serverless-stack/resources";
type CreateSiteOptions = {
...
auth: Cognito;
};
type CreateSite = (...) => ViteStaticSite;
export const createSite: CreateSite = (stack, { ..., auth }) =>
new ViteStaticSite(stack, "site", {
path: "frontend",
environment: {
...
VITE_API_IDENTITY_POOL_ID: String(auth.cdk.cfnIdentityPool?.ref),
},
});
code ./stacks/resources/index.ts
...
export * from "./auth";
code ./stacks/MyStack.ts
import { StackContext } from "@serverless-stack/resources";
import {
...
createAuth,
} from "./resources";
export function MyStack({ stack }: StackContext) {
...
const auth = createAuth(stack, { bucket });
const site = createSite(stack, { api, bucket, auth });
...
}
npm start # or press ENTER to redeploy if SST is already running
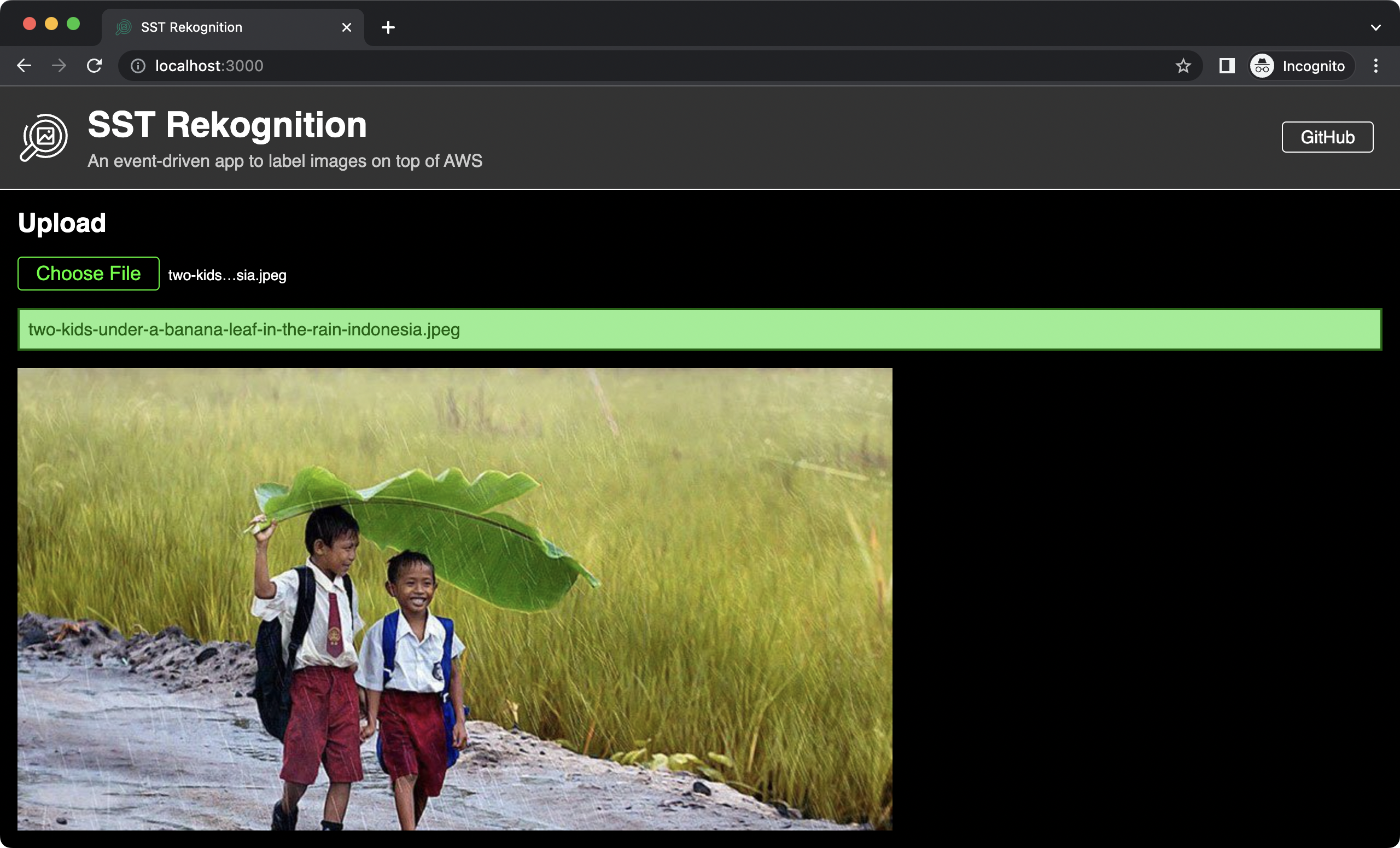
git add .
git commit -m "Cognito Auth"