-
Notifications
You must be signed in to change notification settings - Fork 2
05 API Gateway
JP Barbosa edited this page Sep 24, 2022
·
9 revisions
code ./stacks/resources/api.ts
import { Api, Stack, Table } from "@serverless-stack/resources";
type CreateApiOptions = { table: Table };
type CreateApi = (stack: Stack, options: CreateApiOptions) => Api;
export const createApi: CreateApi = (stack, { table }) => {
const api = new Api(stack, "api", {
defaults: {
function: {
environment: {
table: table.tableName,
},
},
},
routes: {
"GET /": "functions/results.handler",
},
});
api.attachPermissions([table]);
return api;
};
code ./stacks/resources/index.ts
...
export * from "./api";
code ./stacks/MyStack.ts
...
import {
...
createApi,
} from "./resources";
export function MyStack({ stack }: StackContext) {
...
const api = createApi(stack, { table });
stack.addOutputs({
ApiEndpoint: api.url,
});
}
code ./services/functions/results.ts
import { APIGatewayProxyHandlerV2 } from "aws-lambda";
import { DynamoDB } from "aws-sdk";
const dynamoDb = new DynamoDB.DocumentClient();
export const handler = async (event: APIGatewayProxyHandlerV2) => {
const params = {
TableName: String(process.env.table),
};
try {
const result = await dynamoDb.scan(params).promise();
const sortedResult = {
...result,
Items: result.Items?.sort((a, b) => {
return b.createdAt - a.createdAt;
}),
};
return {
statusCode: 200,
body: JSON.stringify(sortedResult),
};
} catch (error) {
return {
statusCode: 500,
body: JSON.stringify(error),
};
}
};
npm start # or press ENTER to redeploy if SST is already running
open https://console.sst.dev/sst-rekognition/sst-jp/local
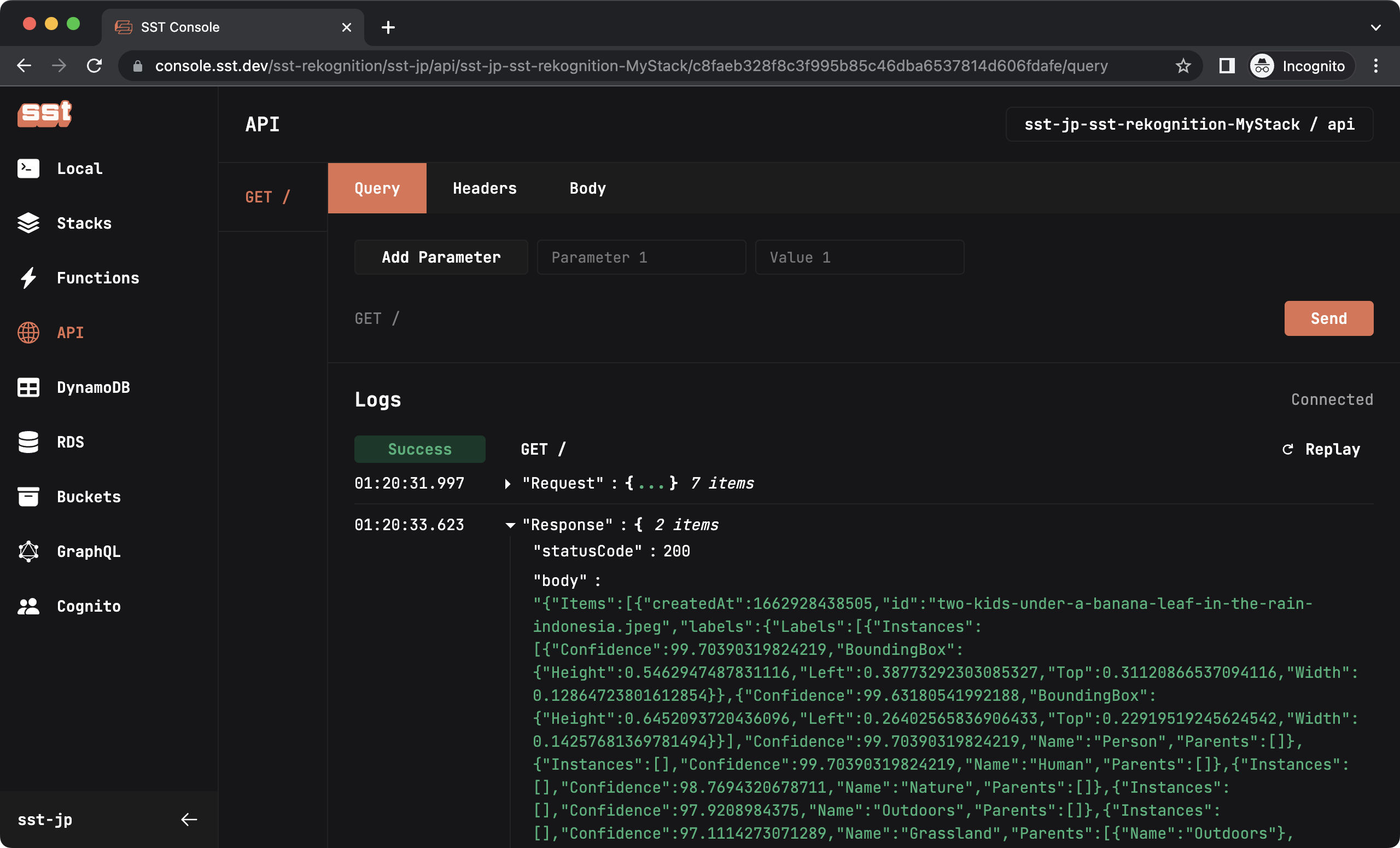
git add .
git commit -m "API Gateway"