-
Notifications
You must be signed in to change notification settings - Fork 2
11 Labels UI
JP Barbosa edited this page Sep 24, 2022
·
5 revisions
code ./frontend/src/components/Labels.tsx
import { useAppContext } from "../contexts/AppContext";
import { LabelsItem } from "./LabelsItem";
export const Labels: React.FC = () => {
const { selectedItem } = useAppContext();
const renderLabels = () => {
if (!selectedItem) {
return (
<div className="box info">
Select an item from the results list to see its labels.
</div>
);
}
if (selectedItem.error) {
return <div className="box error">{selectedItem.error.message}</div>;
}
return (
<ul className="labels">
{selectedItem.labels?.Labels?.map((label) => (
<LabelsItem key={label.Name} label={label} />
))}
</ul>
);
};
return (
<div id="labels">
<h2>Labels</h2>
<div className="scrollable">{renderLabels()}</div>
</div>
);
};
code ./frontend/src/components/LabelsItem.tsx
import { Rekognition } from "aws-sdk";
export type LabelsItemProps = {
label: Rekognition.Label;
};
export const LabelsItem: React.FC<LabelsItemProps> = ({ label }) => {
const { Name, Confidence, Instances } = label;
const confidence = Confidence ? Math.round(Confidence * 10) / 10 : 0;
const instances = Instances ? Instances.length : 0;
return (
<li>
<div className="bar" style={{ width: `${confidence}%` }}></div>
<div className="label">
<span className="name">{Name}</span>
<span className="info">
{confidence}%{instances ? ` with ${instances} Instances` : ""}
</span>
</div>
</li>
);
};
code ./frontend/src/App.tsx
...
import { Labels } from "./components/Labels";
function App() {
return (
<div id="app">
<AppContextProvider>
<Header />
<div className="content">
<Upload />
<Results />
<Labels />
</div>
</AppContextProvider>
</div>
);
}
export default App;
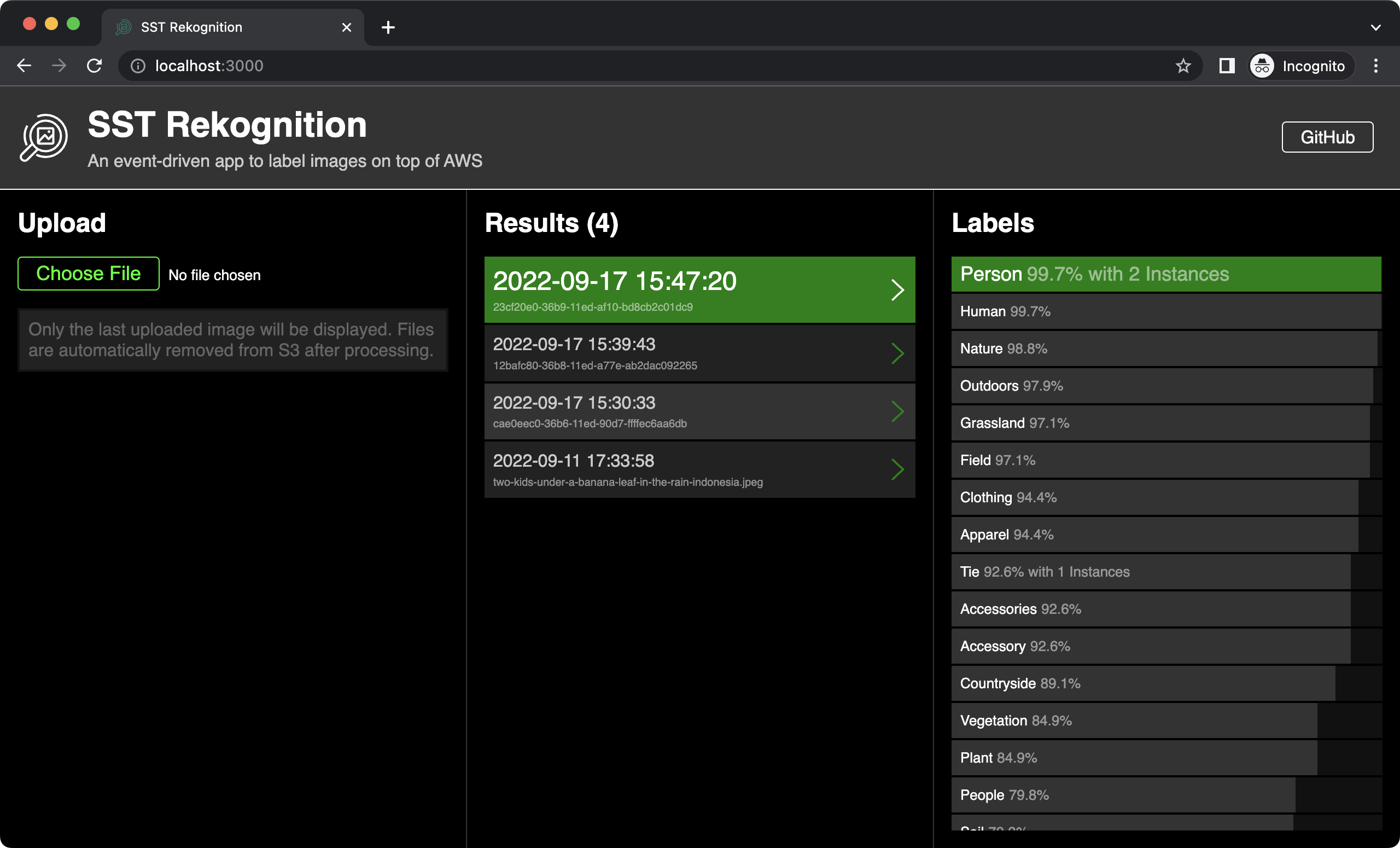
git add .
git commit -m "Labels UI"