Add tcp_no_delay parameter to sensor models #211
Merged
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
This adds the
tcp_no_delay
ROS parameter to all sensor models. This parameter is equivalent to the~[sensor]_nodelay
parameter in https://github.com/cra-ros-pkg/robot_localization/blob/melodic-devel/doc/state_estimation_nodes.rst#sensor_nodelay.You can easily see the impact of enabling
TCP_NODELAY
withrostopic delay --tcpnodelay <topic>
, which can be compared againstrostopic delay <topic>
, e.g.:I've used this simple Python node to generate dummy messages:
I've also computed the delay in the
fuse_models::Odometry2D::process
method, with this changes wrt this PR:With this I can easily plot the delay and see the impact of
TCP_NODELAY
:tcp_no_delay: false
(default):tcp_no_delay: true
:The next plot shows the delay for
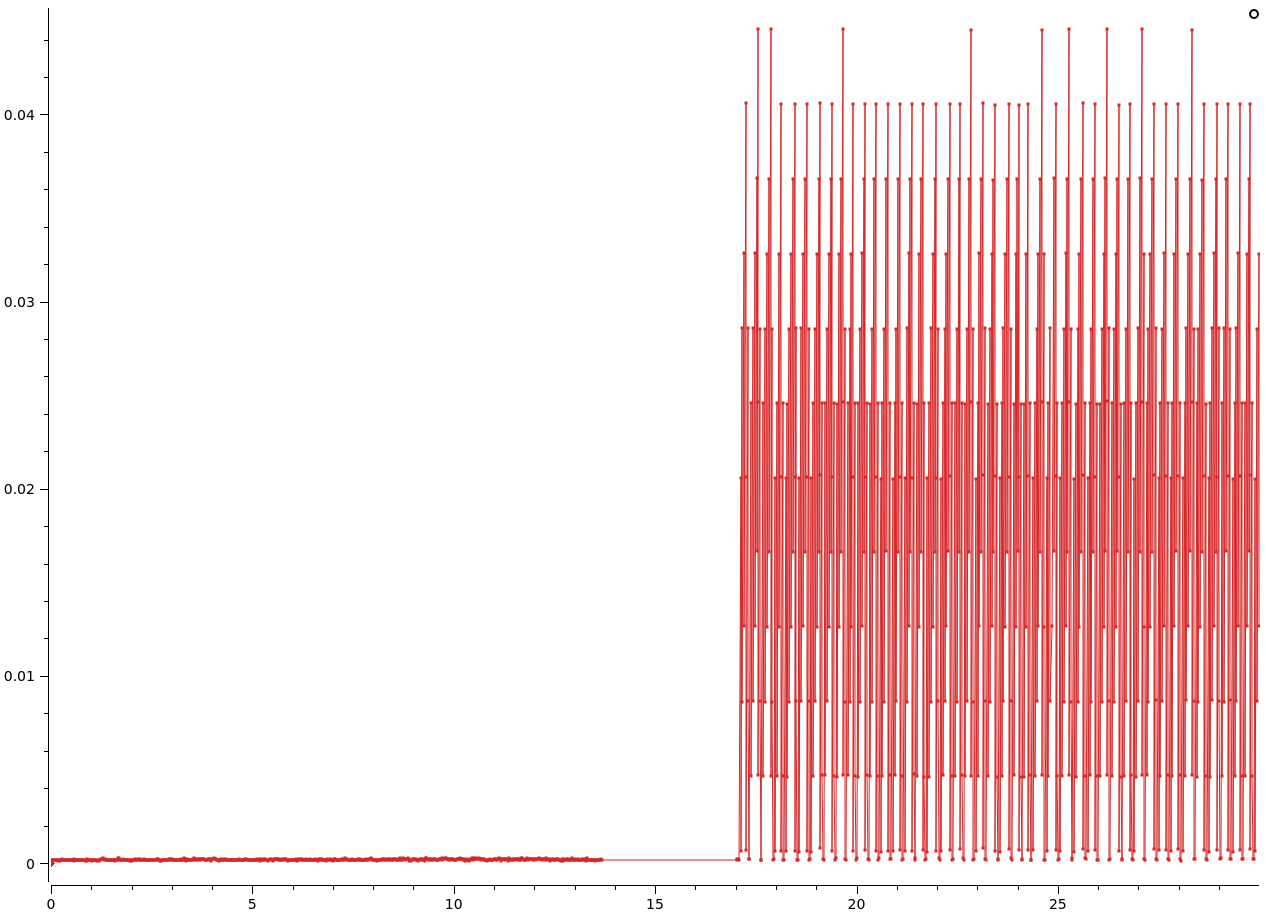
tcp_no_delay: true
andtcp_no_delay: false
one after the other, so it's easier to see the impact, with the same axes scale:We could do the same in simulation, but in that case the delay is computed using the simulated time, not the wall time, so there are some discretization artifacts, e.g. with simulation running at 100Hz:
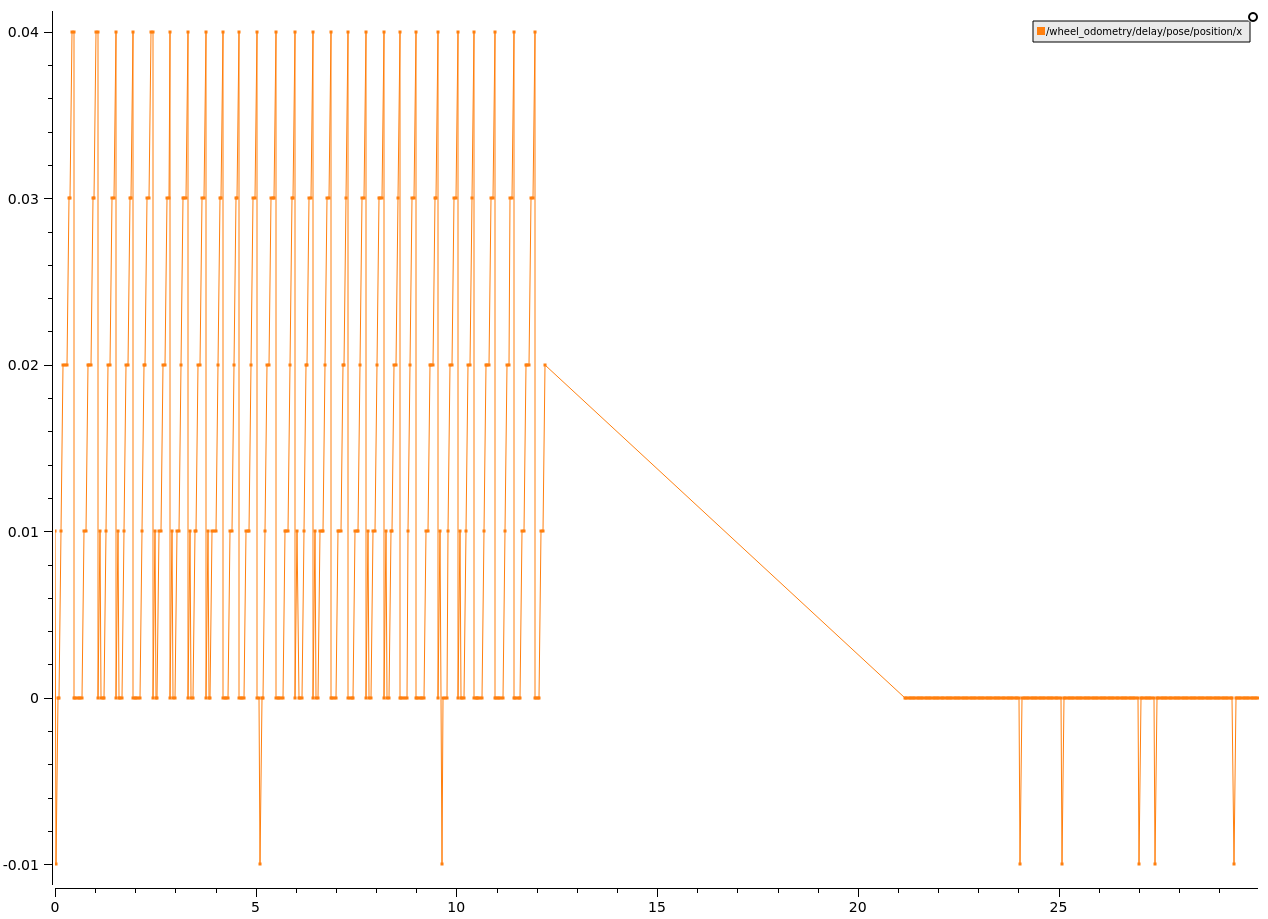
This also allows to see that for
nav_msgs::Odometry
messages, Nagle's algorithm buffers approximately 11-12 messages.More information on
TCP_NODELAY
and other related topics can be found in:https://www.extrahop.com/company/blog/2016/tcp-nodelay-nagle-quickack-best-practices/