Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
To make SDK easier to use builder pattern was implemented in the file
Builder.ts
. From an implementation point of view, the "Builder with a twist" design pattern also known as "Step builder" was specifically chosen because of the large number of mandatory fields. In short this pattern uses the common builder pattern, but adds a twist by using interface to force which value to set next and thereby solving the problem with many mandatory values.Builder pattern supports following use cases:
To use Builder API, developer first imports a
Builder
function as follows:import { Builder } from '@paraspell/sdk'
Builder function accepts one parameter of type
ApiPromise
. Then, other functions can be chained depending on the use case. In the case of initiating a token transfer the Builder class decides which scenario to use depending on thefrom()
andto()
methods. Depending on the scenario different mandatory fields are required. Every step of the build process is properly typed and therefore invalid object cannot be created. After providing all required parameters thebuild()
method executes the transaction or open/closes a channel. Mandatory fields must be specified in the same order as they are shown in the example otherwise typescript error will be generated.Developer experience
When developing, the developer is guided by the typescript and thus knows which parameter can be added as next. This increases the developer experience and makes SDK easier to use.
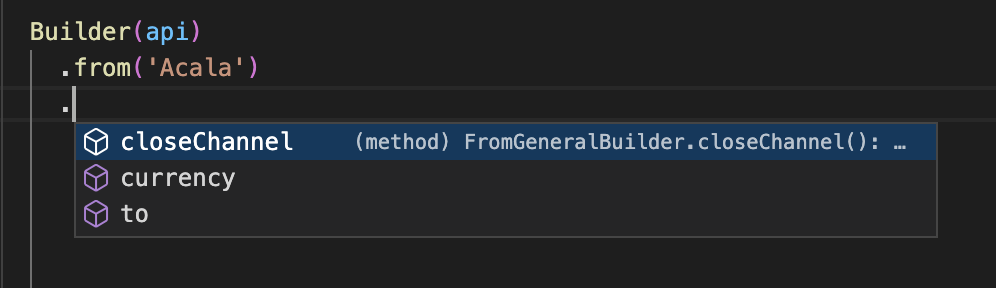
Other changes:
Builder.test.ts
. unit tests are designed in such a way that they check the parameters of the target function, which each case of using the builder pattern calls whenbuild()
method is executed. This is achieved by the spy function from vitest.Examples of all use cases
Transfer tokens from parachain to parachain
Both
from
andto
parameters are provided, thus the parachain to parachain scenario will be used.Transfer tokens from relaychain to parachain
Only
from
parameter is provided, thus the relaychain to parachain scenario will be used.Transfer tokens from parachain to relaychain
Only
to
parameter is provided, thus the parachain to relaychain scenario will be used.Open a channel
When opening a new channel the operation has to be specified by calling
openChannel()
method and then providingmaxSize
andmaxMessageSize
parameters.Close a channel
When closing channels the operation has to be specified by calling
closeChannel()
method and then providinginbound
andoutbound
parameters.