FlutterQuill is a rich text editor and a Quill component for Flutter.
This library is a WYSIWYG editor built for the modern mobile platform, with web compatibility under development. You can join our Slack Group for discussion.
Demo App: https://bulletjournal.us/home/index.html
Pub: https://pub.dev/packages/flutter_quill
See the example
directory for a minimal example of how to use FlutterQuill. You typically just need to instantiate a controller:
QuillController _controller = QuillController.basic();
and then embed the toolbar and the editor, within your app. For example:
Column(
children: [
QuillToolbar.basic(controller: _controller),
Expanded(
child: Container(
child: QuillEditor.basic(
controller: _controller,
readOnly: false, // true for view only mode
),
),
)
],
)
Check out Sample Page for advanced usage.
This library uses Quill as an internal data format.
- Use
_controller.document.toDelta()
to extract the deltas. - Use
_controller.document.toPlainText()
to extract plain text.
FlutterQuill provides some JSON serialisation support, so that you can save and open documents. To save a document as JSON, do something like the following:
var json = jsonEncode(_controller.document.toDelta().toJson());
You can then write this to storage.
To open a FlutterQuill editor with an existing JSON representation that you've previously stored, you can do something like this:
var myJSON = jsonDecode(incomingJSONText);
_controller = QuillController(
document: Document.fromJson(myJSON),
selection: TextSelection.collapsed(offset: 0));
The QuillToolbar
class lets you customise which formatting options are available.
Sample Page provides sample code for advanced usage and configuration.
For web development, use flutter config --enable-web
for flutter and use ReactQuill for React.
It is required to provide EmbedBuilder
, e.g. defaultEmbedBuilderWeb.
Also it is required to provide webImagePickImpl
, e.g. Sample Page.
It is required to provide filePickImpl
for toolbar image button, e.g. Sample Page.
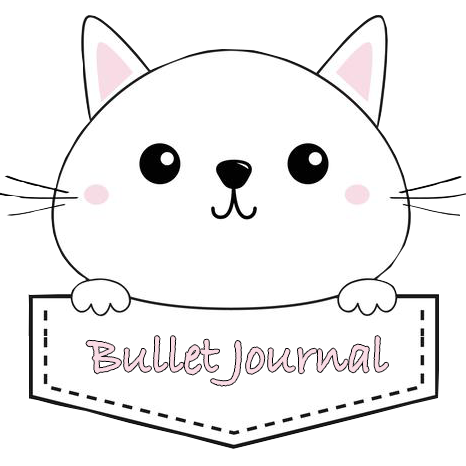