You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
#include"MyClass.h"structMyClass::MyInnerClass {
MyInnerClass(bool a, bool b) {
}
};
MyClass::MyClass() {
newMyInnerClass(true, true);
}
This is correct C++, but the constructor which is declared in the .cpp file is not resolved properly:
This happens only if MyInnerClass is instantiated using the new operator, e.g. MyInnerClass inner(true, true) will work fine.
Explaination
I debugged this issue and will try to provide a fix soon. My current findings are best illustrated by the following stack trace: CPPSemantics::resolveAmbiguities tries to find the binding for the constructor, by analyzing all constructors. Constructors which are not declared yet, are filtered out (CPPSemantics::2228).
The constructors are indexed, hence they are IPDOMBindings. CPPSemantics::declaredBefore treats ICPPInternalBindings and IPDOMBindings differently. In the latter case the implementation will use the IIndexFileSet of the IASTTranslationUnit and check if it contains the currently analyzed constructor.
When the constructor is declared in the header file, it will be found in the index file set, but in our case it won't. As a result, the constructor candidate is filtered out and not passed to the following logic.
Fun Fact
When there is another constructor defined in the header file, which has a different signature, but the same number of arguments, the bug disappears:
#ifndef MYCLASS_H_
#defineMYCLASS_H_structMyClass {
MyClass();
structMyInnerClass {
MyInnerClass(int a, int b) {
}
};
};
#endif/* MYCLASS_H_ */
This is due to the fact that CPPSemantics::resolveAmbiguities passes the list of the filtered constructors to CPPSemantics::resolveFunction, which however uses the original (unfiltered list) and only uses the filtered list to exclude constructors by the number of its arguments.
The text was updated successfully, but these errors were encountered:
Bug Description
Consider the following simple example (struct is used for simplicity, the problem also applies to classes):
MyClass.h
MyClass.cpp
This is correct C++, but the constructor which is declared in the .cpp file is not resolved properly:
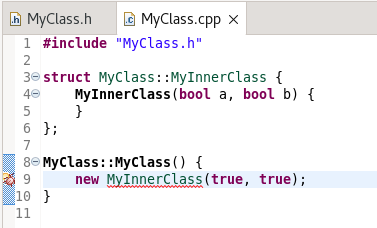
This happens only if
MyInnerClass
is instantiated using thenew
operator, e.g.MyInnerClass inner(true, true)
will work fine.Explaination
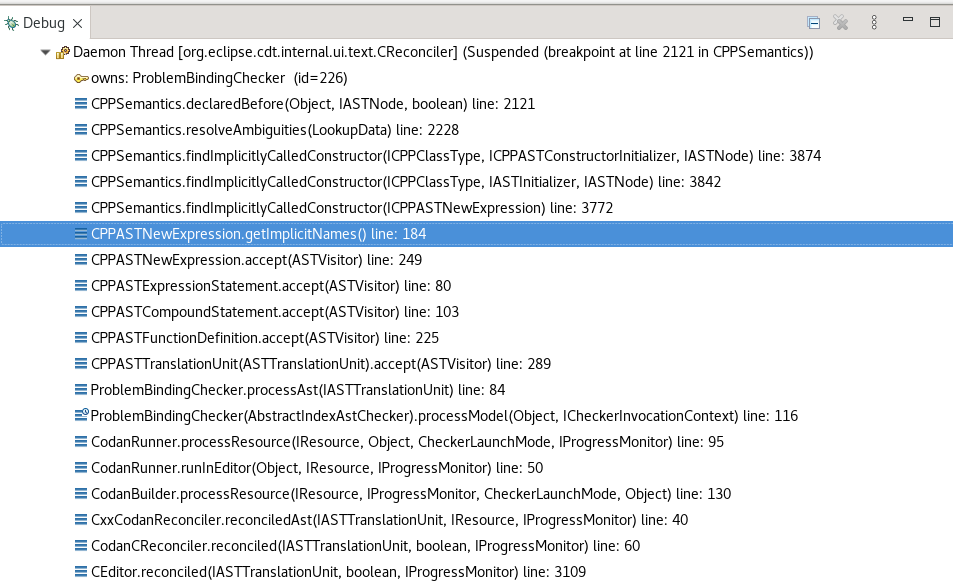
I debugged this issue and will try to provide a fix soon. My current findings are best illustrated by the following stack trace:
CPPSemantics::resolveAmbiguities
tries to find the binding for the constructor, by analyzing all constructors. Constructors which are not declared yet, are filtered out (CPPSemantics::2228
).The constructors are indexed, hence they are
IPDOMBinding
s.CPPSemantics::declaredBefore
treatsICPPInternalBinding
s andIPDOMBinding
s differently. In the latter case the implementation will use theIIndexFileSet
of theIASTTranslationUnit
and check if it contains the currently analyzed constructor.When the constructor is declared in the header file, it will be found in the index file set, but in our case it won't. As a result, the constructor candidate is filtered out and not passed to the following logic.
Fun Fact
When there is another constructor defined in the header file, which has a different signature, but the same number of arguments, the bug disappears:
This is due to the fact that
CPPSemantics::resolveAmbiguities
passes the list of the filtered constructors toCPPSemantics::resolveFunction
, which however uses the original (unfiltered list) and only uses the filtered list to exclude constructors by the number of its arguments.The text was updated successfully, but these errors were encountered: