-
Notifications
You must be signed in to change notification settings - Fork 5.2k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
(feat): Generate scaffold in cli #12298
Conversation
Hey @StorytellerCZ check if those changes that I've made makes sense to you |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I think these are good initial steps.
In this PR I focus on the creation of a new CLI command called generate, that will for the app user generate a scaffold API for him, this gives to the end user a little more time and a better DX since, he will be not needing to write this code that almost every MeteorJS RPC API has:
here is an example of usage. (in a simple js app)
it will generate the following code in
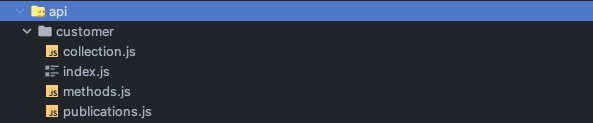
/imports/api
that will have the following code:
collection.js
methods.js
publication.js
index.js
Also, there is the same version of these methods using TypeScript.
Path option
for those that may want to create in another path, you can use the
--path
option in order to select where to place this boilerplate.It will generate the model in that path.
it will generate in
server/admin
the another-client code:collection.ts
methods.ts
publications.ts
index.ts
Using the Wizard
if you run the following command:
it will prompt the following questions. I've answered them enter for all of them
Using your own template
You can use your own templates for scaffolding your specific workloads. To do that, you should pass in a template directory URL so that it can copy it with its changes.
how do I rename things?
Out of the box I provide a few functions such as replacing
$$name$$
,$$PascalName$$
and$$camelName$$
these replacements come from this function:
What if I want to have my own way of templating?
There is an option called
--replaceFn
that when you pass in given a .js file with two functions it will override all templating that we have defaulted to use your given function.example of a replacer file
if you run your command like this:
it will generate files full of
$$PascalCase$$
using the meteor provided templates.A better example of this feature would be the following js file (like we do inside the cli):