-
Notifications
You must be signed in to change notification settings - Fork 8
Quickstart
Read on to get up-and-running with Allegro quickly.
It's assumed that you already have some knowledge of C or C++, and have a compiler installed - if not, see Learning C and C++.
Installing Allegro as a binary package is the easiest method for most operating systems.
Choose your weapon:
Linux
First, add the Allegro PPA. This gives you up-to-date versions of Allegro; the base repos only provide 5.2.3 at the time of writing.
sudo add-apt-repository ppa:allegro/5.2
Then, install Allegro:
sudo apt-get install liballegro*5.2 liballegro*5-dev
sudo apt-get install liballegro-ttf5-dev
sudo dnf install allegro5*
sudo pacman -S allegro
sudo zypper install liballegro*
Binary packages may be available for your distro; feel free to add them here if so.
Otherwise, select "Something else" below.
OSX
Install with Homebrew
brew install allegro
You will also need to install pkg-config, if it is not already installed.
brew install pkg-config
Windows
Install per-project using NuGet in PowerShell:
cd MyProjectDir\
Install-Package Allegro
Or, just install from within Visual Studio.
- Find msys Allegro packages and pick a binary package of the newest version: https://packages.msys2.org/search?q=allegro
- For example for 5.2.9: pacman -S mingw-w64-clang-x86_64-allegro
Android
Using Allegro for mobile apps is a bit trickier. We recommend building a desktop program first.
See the Maven repository.
iOS
Using Allegro for mobile apps is a bit trickier. We recommend building a desktop program first.
See the iOS README.
TODO: Adapt from this.
Something else
If you're running another OS or want to do something that's not possible with the provided binary packages (eg. static linking often isn't trivial with them), you'll need to build Allegro from source.
If you're having problems installing, refer to the in-depth instructions.
Once you've installed Allegro, you can check that it compiles into a simple program.
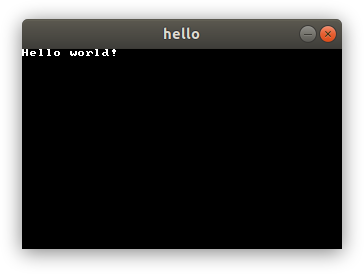
The program will just display the above window and exit when any key is pressed.
Create hello.c
with the below code:
View source
#include <allegro5/allegro5.h>
#include <allegro5/allegro_font.h>
#include <stdbool.h>
int main()
{
al_init();
al_install_keyboard();
ALLEGRO_TIMER* timer = al_create_timer(1.0 / 30.0);
ALLEGRO_EVENT_QUEUE* queue = al_create_event_queue();
ALLEGRO_DISPLAY* disp = al_create_display(320, 200);
ALLEGRO_FONT* font = al_create_builtin_font();
al_register_event_source(queue, al_get_keyboard_event_source());
al_register_event_source(queue, al_get_display_event_source(disp));
al_register_event_source(queue, al_get_timer_event_source(timer));
bool redraw = true;
ALLEGRO_EVENT event;
al_start_timer(timer);
while(1)
{
al_wait_for_event(queue, &event);
if(event.type == ALLEGRO_EVENT_TIMER)
redraw = true;
else if((event.type == ALLEGRO_EVENT_KEY_DOWN) || (event.type == ALLEGRO_EVENT_DISPLAY_CLOSE))
break;
if(redraw && al_is_event_queue_empty(queue))
{
al_clear_to_color(al_map_rgb(0, 0, 0));
al_draw_text(font, al_map_rgb(255, 255, 255), 0, 0, 0, "Hello world!");
al_flip_display();
redraw = false;
}
}
al_destroy_font(font);
al_destroy_display(disp);
al_destroy_timer(timer);
al_destroy_event_queue(queue);
return 0;
}
gcc hello.c -o hello $(pkg-config allegro-5 allegro_font-5 --libs --cflags)
./hello
Note that on Windows, a terminal window will also appear when you run your program. This is useful for debugging, but can be disabled by using the -mwindows
flag when compiling.
gcc hello.c -o hello.exe -lallegro -lallegro_font
hello.exe
gcc hello.c -o hello $(pkg-config allegro-5 allegro_main-5 allegro_font-5 --libs --cflags)
./hello
See Creating-macOS-bundles for how to distribute your game as an OSX bundle.
Configure Allegro for your project and then simply compile and run as normal.
Congratulations - you've got a working program! (hopefully.)
Next, read Allegro Vivace for a proper tutorial.
Or, dive straight into the reference manual.